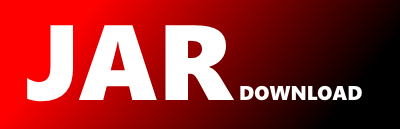
com.day.cq.dam.commons.util.S73DHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
package com.day.cq.dam.commons.util;
import java.util.ArrayList;
import java.util.Iterator;
import javax.jcr.Node;
import javax.jcr.NodeIterator;
import javax.jcr.RepositoryException;
import javax.jcr.Session;
import org.apache.sling.api.resource.Resource;
import org.apache.sling.api.resource.ValueMap;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.day.cq.dam.commons.util.S73DConstants;
//import com.day.cq.dam.api.Asset;
import com.adobe.granite.asset.api.Asset;
import com.day.cq.commons.jcr.JcrConstants;
import com.day.cq.dam.api.DamConstants;
import com.day.cq.dam.api.s7dam.constants.S7damConstants;
public class S73DHelper {
private static final Logger log = LoggerFactory.getLogger(S73DHelper.class);
/**
*
* @param resource resource instance
* @return true if the 3D package is installed and configured as active otherwise returns false.
*
*/
static final String [] MimeTypes3D =
{
"application/x-v3d",
"application/x-maya",
"application/x-fbx",
"application/x-obj",
"application/x-max"
};
public static boolean isV3DInstalled(Session session)
{
try
{
Node rootNode = session.getRootNode();
Node pathNode = null;
pathNode = rootNode.getNode(S73DConstants.SETTINGS_NODE);
if(pathNode!=null)
{
String propertyName="isActive";
if (pathNode.hasProperty(propertyName))
return pathNode.getProperty(propertyName).getBoolean();
else
return true; // assume active if property not set
}
} catch (RepositoryException e1) {
log.error("3D-ERROR: sV3DInstalled Failed while looking for V3D App");
e1.printStackTrace();
return false;
}
return false;
}
/**
* Returns the S7 type for the given resource.
*
* @param resource resource instance
* @return the S7 type or null if the type is missing
*
*/
public static String getS7Type(Resource resource)
{
if(resource==null)
{
return null;
}
Resource contentResource = resource.getChild(JcrConstants.JCR_CONTENT);
ValueMap vm = (contentResource != null) ? contentResource.adaptTo(ValueMap.class) : null;
return (vm != null) ? vm.get(S7damConstants.PN_S7_TYPE, String.class) : null;
}
/**
* Checks whether the given resource represent an S7 3D model or not.
* @param resource resource instance
* @return true if the resource is marked as a 3D resource, false otherwise
*/
public static boolean isS73D(Resource resource)
{
if(resource==null)
{
return false;
}
String s7Type = getS7Type(resource);
if (S73DConstants.S73D_OBJECT.equals(s7Type)
|| S73DConstants.S73D_SCENE.equals(s7Type)
|| S73DConstants.S73D_STAGE.equals(s7Type)){
//log.info("isS73D: 3D RESOURCE FOUND");
return true;
}
return false;
}
/**
* Does the resource contain a model folder - indicating 3D ingestion has been applied
* @param resource resource instance
* @return true if a model directory exists, otherwise false
*/
public static boolean hasModel(Resource resource)
{
if(resource==null)
return false;
if(!is3DMimeType(resource))
return false;
Resource contentResource = resource.getChild(JcrConstants.JCR_CONTENT);
Node content = (contentResource != null) ? contentResource.adaptTo(Node.class) : null;
try {
if(content!=null && content.hasNode("model"))
return true;
else
return false;
} catch (RepositoryException e) {
log.error("3D-ERROR: hasModel Failed ");
e.printStackTrace();
return false;
}
}
/**
* Checks whether the given resource represent an S7 3D model or not.
* @param resource resource instance
* @return true if the resource is marked as a 3D resource, false otherwise
*/
public static boolean is3DMimeType(Resource resource)
{
assert(resource!=null);
Asset asset = resource.adaptTo(Asset.class);
return (get3DMimeType(asset)!=null);
}
/**
* Get a list of dependencies.
* @param resource resource instance
* @return a string array of dependency paths or null if not 3D or no dependencies
*/
public static String[] getDependencyPaths(Resource resource)
{
assert(resource!=null);
ArrayList deps = new ArrayList();
if(isS73D(resource))
{
try {
Asset asset = resource.adaptTo(Asset.class);
Iterator extends Asset> ai;
for (ai = asset.listRelated("links"); ai.hasNext();)
{
Node ni = ai.next().adaptTo(Node.class);
deps.add(ni.getProperty("sling:resource").getString());
}
} catch (RepositoryException e) {
log.error("3D-ERROR: getDependencyPaths Failed ");
e.printStackTrace();
return null;
}
}
if(deps.isEmpty())
return null;
String dependencies[] = new String[deps.size()];
for(int i=0;i0);
}
else
return false;
} catch (RepositoryException e) {
log.error("3D-ERROR: isIncomplete Failed");
e.printStackTrace();
return false;
}
}
return false;
}
/**
* Returns the progress value for the given resource
* @param resource resource instance
* @return percentage string if the resource represent a S7 model and has a progress value
* empty string otherwise
*/
public static String getProgressValue(Resource resource)
{
assert(resource!=null);
if(isS73D(resource))
{
Resource contentResource = resource.getChild("jcr:content/progress");
if (contentResource != null) {
ValueMap vm = contentResource.adaptTo(ValueMap.class);
String value = vm.get("Progress", "");
return value;
}
}
return "";
}
/**
* Returns the progress operation for the given resource
* @param resource resource instance
* @return operation string if the resource represent a S7 model and has a progress value
* empty string otherwise
*/
public static String getProgressOperation(Resource resource)
{
assert(resource!=null);
if(isS73D(resource))
{
Resource contentResource = resource.getChild("jcr:content/progress");
if (contentResource != null) {
ValueMap vm = contentResource.adaptTo(ValueMap.class);
String value = vm.get("Operation", "");
return value;
}
}
return "";
}
/**
* Returns true if the metadata based mime type is recognized as a 3D resource - uses DC_FORMAT
* @param resource resource
* @return true if set to 3D mime type, false if not
*/
public static boolean has3DMimeTypeDC(Resource resource)
{
Resource contentResource = resource.getChild("jcr:content/metadata");
if (contentResource != null)
{
ValueMap vm = contentResource.adaptTo(ValueMap.class);
String value = vm.get(DamConstants.DC_FORMAT, "").toLowerCase();
for(String type: MimeTypes3D)
{
if(value.equals(type))
return true;
}
}
return false;
}
/**
* Returns true if the metadata based mime type is recognized as a 3D resource - uses JCR_FORMAT
* @param resource resource
* @return true if set to 3D mime type, false if not
*/
public static boolean has3DMimeTypeJCR(Resource resource)
{
Resource contentResource = resource.getChild("jcr:content/metadata");
if (contentResource != null)
{
ValueMap vm = contentResource.adaptTo(ValueMap.class);
String value = vm.get(JcrConstants.JCR_MIMETYPE, "").toLowerCase();
for(String type: MimeTypes3D)
{
if(value.equals(type))
return true;
}
}
return false;
}
/**
* Returns the mime type string for a 3D asset
* @param asset asset
* @return mime type string if 3D asset or null if not
*/
public static String get3DMimeType(Asset asset)
{
if(asset==null)
return null;
else
return get3DMimeType(asset.getName());
}
/**
* Returns the mime type string for an asset or file path based on the extension
* @param fileName filename
* @return mime type string if recognized 3D extension or null if not
*/
public static String get3DMimeType(String fileName)
{
String mimeType=null;
String extension = fileName.substring(fileName.lastIndexOf('.') + 1);
// System.out.println("extension: " + extension);
if (extension.equalsIgnoreCase("v3d"))
mimeType = "application/x-v3d";
else if (extension.equalsIgnoreCase("ma") || extension.equalsIgnoreCase("mb"))
mimeType = "application/x-maya"; // problematic as this may indeed be a mathematica file
else if (extension.equalsIgnoreCase("fbx"))
mimeType = "application/x-fbx";
else if (extension.equalsIgnoreCase("obj"))
mimeType = "application/x-obj";
else if (extension.equalsIgnoreCase("max"))
mimeType = "application/x-max";
return mimeType;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy