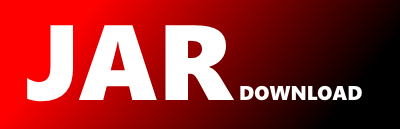
com.day.cq.wcm.msm.api.Blueprint Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* Copyright 1997-2011 Day Management AG
* Barfuesserplatz 6, 4001 Basel, Switzerland
* All Rights Reserved.
*
* This software is the confidential and proprietary information of
* Day Management AG, ("Confidential Information"). You shall not
* disclose such Confidential Information and shall use it only in
* accordance with the terms of the license agreement you entered into
* with Day.
*/
package com.day.cq.wcm.msm.api;
import java.util.Calendar;
import java.util.Iterator;
import java.util.List;
import java.util.Locale;
import org.apache.sling.api.adapter.Adaptable;
import org.apache.sling.api.resource.Resource;
import org.apache.sling.api.resource.ValueMap;
import com.day.cq.commons.Filter;
import com.day.cq.commons.JSONItem;
import com.day.cq.commons.LabeledResource;
import com.day.cq.tagging.Tag;
import com.day.cq.wcm.api.Page;
import com.day.cq.wcm.api.PageManager;
import com.day.cq.wcm.api.Template;
import com.day.cq.wcm.api.WCMException;
/**
* Defines the interface of a CQ MSM Blueprint.
* A Blueprint is a Template for a Site, it defines the Pages that are part of
* a Site and the {@link RolloutConfig actions} that should be applied upon a
* rollout of a Page from the Site template.
* Note:
* The support for the Page-Api is deprecated since 5.4.0
* The Blueprint is no longer required to be a Page.
*/
public interface Blueprint extends com.day.cq.wcm.api.msm.Blueprint, LabeledResource, Adaptable, JSONItem {
/**
* Returns the path to an icon for this template or null
if
* this template does not provide an icon.
*
* @return the path to an icon or null
*/
String getIconPath();
/**
* Returns the path to a thumbnail for this template or null
* if the template does not provide a thumbnail.
*
* @return the path to a thumbnail or null
*/
String getThumbnailPath();
/**
* Returns the ranking in the template list.
*
* @return the ranking
*/
Long getRanking();
/**
* Returns the site path of the blueprint
* @return path
*/
public String getSitePath();
/**
* Access the Configuration defined for a Page that is part of this
* Blueprint.
* Give the path path to the Page you are interested in relative to the
* Blueprints {@link #getSitePath() root}.
* If you want to access the configuration for the root itself pass
* null
.
* The method returns the RolloutConfig that is effective on the given path.
* If you only want to access the Config that is specified on the given Page
* set the exact argument to be true.
* Note: It is not required that the path exists.
*
* @param relPath a Path relative to the Blueprint's Site root.
* @param exact if false the configuration that is effective is returned, if
* true only the configuration configured at the path is returned
* @return List of {@link com.day.cq.wcm.msm.api.RolloutConfig RolloutConfigs}
* for the given Path or null
if none
* @throws WCMException in case of error accessing the Blueprint
* @since 5.4
*/
List getBlueprintRolloutConfig(String relPath, boolean exact)
throws WCMException;
/**
* @deprecated since 5.4, access page instead
*/
@Deprecated
PageManager getPageManager();
/**
* @deprecated since 5.4, access page instead
*/
@Deprecated
Resource getContentResource();
/**
* @deprecated since 5.4, access page instead
*/
@Deprecated
Resource getContentResource(String relPath);
/**
* @deprecated since 5.4, access page instead
*/
@Deprecated
Iterator listChildren();
/**
* @deprecated since 5.4, access page instead
*/
@Deprecated
Iterator listChildren(Filter filter);
/**
* @deprecated since 5.4, access page instead
*/
@Deprecated
boolean hasChild(String name);
/**
* @deprecated since 5.4, access page instead
*/
@Deprecated
int getDepth();
/**
* @deprecated since 5.4, Page Blueprint is not required to be a Page
*/
@Deprecated
Page getParent();
/**
* @deprecated since 5.4, Page Blueprint is not required to be a Page
*/
@Deprecated
Page getParent(int level);
/**
* @deprecated since 5.4, Page Blueprint is not required to be a Page
*/
@Deprecated
Page getAbsoluteParent(int level);
/**
* @deprecated since 5.4, Page Blueprint is not required to be a Page
*/
@Deprecated
ValueMap getProperties();
/**
* @deprecated since 5.4, Page Blueprint is not required to be a Page
*/
@Deprecated
ValueMap getProperties(String relPath);
/**
* @deprecated since 5.4, Page Blueprint is not required to be a Page
*/
@Deprecated
String getPageTitle();
/**
* @deprecated since 5.4, Page Blueprint is not required to be a Page
*/
@Deprecated
String getNavigationTitle();
/**
* @deprecated since 5.4, Page Blueprint is not required to be a Page
*/
@Deprecated
boolean isHideInNav();
/**
* @deprecated since 5.4, Page Blueprint is not required to be a Page
*/
@Deprecated
boolean hasContent();
/**
* @deprecated since 5.4, Page Blueprint is not required to be a Page
*/
@Deprecated
boolean isValid();
/**
* @deprecated since 5.4, Page Blueprint is not required to be a Page
*/
@Deprecated
long timeUntilValid();
/**
* @deprecated since 5.4, Page Blueprint is not required to be a Page
*/
@Deprecated
Calendar getOnTime();
/**
* @deprecated since 5.4, Page Blueprint is not required to be a Page
*/
@Deprecated
Calendar getOffTime();
/**
* @deprecated since 5.4, Page Blueprint is not required to be a Page
*/
@Deprecated
String getLastModifiedBy();
/**
* @deprecated since 5.4, Page Blueprint is not required to be a Page
*/
@Deprecated
Calendar getLastModified();
/**
* @deprecated since 5.4, Page Blueprint is not required to be a Page
*/
@Deprecated
String getVanityUrl();
/**
* @deprecated since 5.4, Page Blueprint is not required to be a Page
*/
@Deprecated
Tag[] getTags();
/**
* @deprecated since 5.4, Page Blueprint is not required to be a Page
*/
@Deprecated
void lock() throws WCMException;
/**
* @deprecated since 5.4, Page Blueprint is not required to be a Page
*/
@Deprecated
boolean isLocked();
/**
* @deprecated since 5.4, Page Blueprint is not required to be a Page
*/
@Deprecated
String getLockOwner();
/**
* @deprecated since 5.4, Page Blueprint is not required to be a Page
*/
@Deprecated
boolean canUnlock();
/**
* @deprecated since 5.4, Page Blueprint is not required to be a Page
*/
@Deprecated
void unlock() throws WCMException;
/**
* @deprecated since 5.4, Page Blueprint is not required to be a Page
*/
@Deprecated
Template getTemplate();
/**
* @deprecated since 5.4, Page Blueprint is not required to be a Page
*/
@Deprecated
Locale getLanguage(boolean ignoreContent);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy