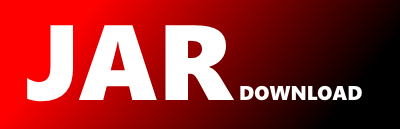
com.adobe.cq.ui.commons.admin.views.PublicationStatusUtils Maven / Gradle / Ivy
/************************************************************************* * * ADOBE CONFIDENTIAL * __________________ * * Copyright 2016 Adobe Systems Incorporated * All Rights Reserved. * * NOTICE: All information contained herein is, and remains * the property of Adobe Systems Incorporated and its suppliers, * if any. The intellectual and technical concepts contained * herein are proprietary to Adobe Systems Incorporated and its * suppliers and are protected by trade secret or copyright law. * Dissemination of this information or reproduction of this material * is strictly forbidden unless prior written permission is obtained * from Adobe Systems Incorporated. * **************************************************************************/ package com.adobe.cq.ui.commons.admin.views; import com.adobe.granite.workflow.exec.WorkItem; import org.slf4j.Logger; import org.slf4j.LoggerFactory; import java.text.DateFormat; import java.util.Locale; import java.util.List; import java.util.LinkedList; import java.util.Collections; import java.util.Map; import java.util.HashMap; import java.util.Comparator; import java.util.ArrayList; import org.apache.commons.lang.StringUtils; import org.apache.sling.api.resource.ResourceResolver; import com.adobe.granite.security.user.util.AuthorizableUtil; import com.adobe.granite.workflow.exec.Workflow; import com.adobe.granite.workflow.job.AbsoluteTimeoutHandler; import com.adobe.granite.workflow.status.WorkflowStatus; import com.day.cq.i18n.I18n; import com.day.cq.commons.servlets.AbstractListServlet; import com.day.cq.replication.Agent; import com.day.cq.replication.AgentManager; import com.day.cq.replication.ReplicationQueue; public class PublicationStatusUtils { //Request publication workflow models used to cover pending for activation private static final String REQUEST_ACTIVATION_WORKFLOW_ID = "/etc/workflow/models/request_for_activation/jcr:content/model"; private static final String REQUEST_DEACTIVATION_WORKFLOW_ID = "/etc/workflow/models/request_for_deactivation/jcr:content/model"; private PublicationStatusUtils() {} private static final Logger log = LoggerFactory.getLogger(PublicationStatusUtils.class); private static String formatAbsoluteDate(Long time, Locale locale) { return DateFormat.getDateTimeInstance(DateFormat.SHORT, DateFormat.SHORT, locale).format(time); } private static boolean isScheduledActivationWorkflow(Workflow workflow) { if (workflow == null) return false; return workflow.getWorkflowModel().getId().equals(AbstractListServlet.ListItem.SCHEDULED_ACTIVATION_WORKFLOW_ID) || workflow.getWorkflowModel().getId().equals(REQUEST_ACTIVATION_WORKFLOW_ID); } private static boolean isScheduledDeactivationWorkflow(Workflow workflow) { if (workflow == null) return false; return workflow.getWorkflowModel().getId().equals(AbstractListServlet.ListItem.SCHEDULED_DEACTIVATION_WORKFLOW_ID) || workflow.getWorkflowModel().getId().equals(REQUEST_DEACTIVATION_WORKFLOW_ID); } /** * Returns the status of the scheduled publications. The returned list allows to join it at usage as required e.g. use
for line breaks in HTML. * @param replicationEntries * @param path * @param i18n * @return */ public static List<br>
for line breaks in HTML. * @param scheduledWorkflows * @param i18n * @param locale * @param resourceResolver * @return */ public static ListgetScheduledStatus(List scheduledWorkflows, I18n i18n, Locale locale, ResourceResolver resourceResolver) { List status = new ArrayList (); for (Workflow scheduledWorkflow : scheduledWorkflows) { if (isScheduledActivationWorkflow(scheduledWorkflow)) { status.add(i18n.get("Publication Pending")); status.add(i18n.get("Version") + ": " + scheduledWorkflow.getWorkflowData().getMetaDataMap().get("resourceVersion", String.class)); } else { status.add(i18n.get("Un-publication Pending")); } status.add("Scheduled:"); Long date = scheduledWorkflow.getWorkflowData().getMetaDataMap().get(AbsoluteTimeoutHandler.ABS_TIME, Long.class); if (date != null) { status.add(formatAbsoluteDate(date, locale)); } status.add(" (" + AuthorizableUtil.getFormattedName(resourceResolver, scheduledWorkflow.getInitiator()) + ")"); } return status; } /** * Returns the status of pending publications. The returned list allows to join it at usage as required e.g. use <br>
getPendingStatus(Map replicationEntries, String path, I18n i18n) { List status = new ArrayList (); if (replicationEntries == null || replicationEntries.isEmpty()) { return status; } if (StringUtils.isEmpty(path)) { return status; } ReplicationQueue.Entry replicationInfo = replicationEntries.get(path); if (replicationInfo == null) { return status; } String actionType = replicationInfo.getAction().getType().getName(); Integer maxQueuePos = replicationInfo.getQueuePosition(); if (actionType == null) return status; if ("Activate".equals(actionType)) { status.add(i18n.get("Publication Pending.")); status.add(i18n.get("#{0} in the queue.", "0 is the position in the queue", maxQueuePos)); } else { status.add(i18n.get("Un-publication Pending.")); status.add(i18n.get("#{0} in the queue.", "0 is the position in the queue", maxQueuePos)); } return status; } /** * Returns the scheduled publication workflows. * @param workflowStatus * @return */ public static List getScheduledWorkflows(WorkflowStatus workflowStatus) { List scheduledWorkflows = new LinkedList (); // Get the scheduled workflows if (workflowStatus != null && workflowStatus.isInRunningWorkflow(false)) { List workflows = workflowStatus.getWorkflows(false); for (Workflow workflow : workflows) { if (isScheduledActivationWorkflow(workflow) || isScheduledDeactivationWorkflow(workflow)) { scheduledWorkflows.add(workflow); } } } // Sort the scheduled workflows by time started Collections.sort(scheduledWorkflows, new Comparator () { public int compare(Workflow o1, Workflow o2) { return o1.getTimeStarted().compareTo(o2.getTimeStarted()); } }); return scheduledWorkflows; } /** * Creates a pending replication map for existing replication agents * * @param agentManager The replication agent manager * @return The pending replication map */ public static Map buildPendingReplicationMap(AgentManager agentManager) { Map replicationMap = new HashMap (); if (agentManager == null) { return replicationMap; } for (Agent agent : agentManager.getAgents().values()) { if (!agent.isInMaintenanceMode()) { ReplicationQueue queue = agent.getQueue(); if (queue != null) { for (ReplicationQueue.Entry entry : queue.entries()) { String path = entry.getAction().getPath(); int currentItemPosition = entry.getQueuePosition(); if (!replicationMap.containsKey(path)) { replicationMap.put(path, entry); } else if (replicationMap.get(path).getQueuePosition() < currentItemPosition) { replicationMap.put(path, entry); } } } } } return replicationMap; } }
© 2015 - 2025 Weber Informatics LLC | Privacy Policy