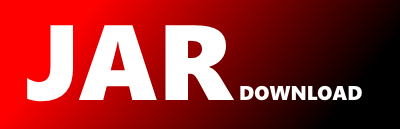
com.adobe.cq.testing.client.security.AbstractProfile Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-cloud-testing-clients Show documentation
Show all versions of aem-cloud-testing-clients Show documentation
AEM related clients and testing utilities for AEM as a Cloud Service
/*
* Copyright 2017 Adobe Systems Incorporated
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.adobe.cq.testing.client.security;
import org.apache.sling.testing.clients.ClientException;
import org.codehaus.jackson.JsonNode;
import java.util.HashMap;
import java.util.Iterator;
import java.util.LinkedHashMap;
/**
* Define and load authorizable's profile properties
*/
public class AbstractProfile implements Profile {
public static final String NODE_PROFILE = "profile";
protected AbstractAuthorizable authorizable;
/**
* Collect the properties for the profile
*/
protected LinkedHashMap profileProps = new LinkedHashMap<>();
/**
* Default constructor for an existing authorizable
*
* @param authorizable any {@link Authorizable} extending the {@link AbstractAuthorizable}
* @param authorizable type
* @throws ClientException if the request to load the authorizable failed
*
*/
public AbstractProfile(T authorizable)
throws ClientException {
if (authorizable == null) {
throw new IllegalArgumentException("Authorizable has to exist and therefore may not be null!");
}
this.authorizable = authorizable;
//load profile properties
loadProperties();
}
/**
* Get profile properties from {@link Authorizable}
*
* @return profile properties as {@link JsonNode}
* @throws ClientException if the request failed
*
*/
public JsonNode getProfileNode() throws ClientException {
return authorizable.getProfile();
}
public HashMap getProperties() {
return profileProps;
}
public void setProperties(HashMap propertiesMap) {
if (propertiesMap == null) {
throw new IllegalArgumentException("Properties map for profile may not be empty!");
}
profileProps.putAll(propertiesMap);
}
/**
* Load properties from Authorizable
*
* @throws ClientException if the request failed
*
*/
private void loadProperties() throws ClientException {
JsonNode profileNode = getProfileNode();
if (profileNode != null) {
for (Iterator fieldNames = profileNode.getFieldNames(); fieldNames.hasNext(); ) {
String fieldName = fieldNames.next();
profileProps.put(fieldName, profileNode.get(fieldName).getTextValue());
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy