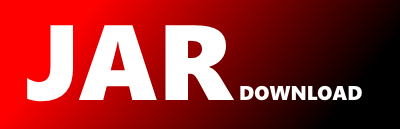
com.adobe.platform.operation.pdfops.options.ocr.OCROptions Maven / Gradle / Ivy
/*
* Copyright 2019 Adobe
* All Rights Reserved.
*
* NOTICE: Adobe permits you to use, modify, and distribute this file in
* accordance with the terms of the Adobe license agreement accompanying
* it. If you have received this file from a source other than Adobe,
* then your use, modification, or distribution of it requires the prior
* written permission of Adobe.
*/
package com.adobe.platform.operation.pdfops.options.ocr;
import java.util.Objects;
/**
* Parameters for converting PDF to a searchable PDF using {@link com.adobe.platform.operation.pdfops.OCROperation}.
*/
public class OCROptions {
private OCRSupportedLocale ocrLocale;
private OCRSupportedType ocrType;
private OCROptions(OCRSupportedLocale ocrLocale, OCRSupportedType ocrType) {
this.ocrLocale = ocrLocale;
this.ocrType = ocrType;
}
/**
* Determines the input language ({@link OCRSupportedLocale}) to be used for OCR
*/
public OCRSupportedLocale getOCRLocale() {
return ocrLocale;
}
/**
* Determines OCR Type. For details, check {@link OCRSupportedType}
*
* @return intended OCR type
*/
public OCRSupportedType getOCRType() {
return ocrType;
}
/**
* Creates a new {@link OCROptions} builder.
*
* @return a {@link OCROptions.Builder} instance
*/
public static OCROptions.Builder ocrOptionsBuilder() {
return new OCROptions.Builder();
}
/**
* Builds a {@link OCROptions} instance.
*/
public static class Builder {
private OCRSupportedLocale ocrLocale;
private OCRSupportedType ocrType;
/**
* Constructs a {@code OCROptions.Builder} instance.
*/
public Builder() {
}
/**
* Sets input language to be used for OCR, specified by {@link OCRSupportedLocale}.
*
* @param ocrSupportedLocale see {@link OCRSupportedLocale}. Default value is {@link OCRSupportedLocale#EN_US}
* @return this Builder instance to add any additional parameters
*/
public OCROptions.Builder withOCRLocale(OCRSupportedLocale ocrSupportedLocale) {
Objects.requireNonNull(ocrSupportedLocale, "OcrLocale must not be null");
this.ocrLocale = ocrSupportedLocale;
return this;
}
/**
* Sets OCR type, specified by {@link OCRSupportedType}
*
* @param ocrSupportedType see {@link OCRSupportedType}. Default value is {@link OCRSupportedType#SEARCHABLE_IMAGE}
* @return this Builder instance to add any additional parameters
*/
public OCROptions.Builder withOCRType(OCRSupportedType ocrSupportedType) {
Objects.requireNonNull(ocrSupportedType, "OcrType must not be null.");
this.ocrType = ocrSupportedType;
return this;
}
/**
* Returns a new {@link OCROptions} instance built from the current state of this builder.
*
* @return a new {@code OCROptions} instance
*/
public OCROptions build() {
return new OCROptions(ocrLocale, ocrType);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy