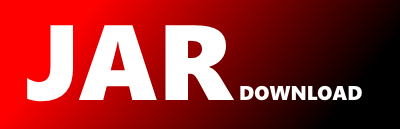
com.adobe.pdfservices.operation.config.ClientConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdfservices-sdk Show documentation
Show all versions of pdfservices-sdk Show documentation
Adobe PDF Services SDK allows you to access RESTful APIs to create, convert, and manipulate PDFs within your applications.
Older versions can be found under groupId: com.adobe.documentservices, artifactId: pdftools-sdk
/*
* Copyright 2024 Adobe
* All Rights Reserved.
*
* NOTICE: Adobe permits you to use, modify, and distribute this file in
* accordance with the terms of the Adobe license agreement accompanying
* it. If you have received this file from a source other than Adobe,
* then your use, modification, or distribution of it requires the prior
* written permission of Adobe.
*/
package com.adobe.pdfservices.operation.config;
import com.adobe.pdfservices.operation.Region;
import com.adobe.pdfservices.operation.config.proxy.ProxyScheme;
import com.adobe.pdfservices.operation.config.proxy.ProxyServerConfig;
import com.adobe.pdfservices.operation.config.proxy.UsernamePasswordCredentials;
import com.adobe.pdfservices.operation.internal.InternalClientConfig;
import com.adobe.pdfservices.operation.internal.constants.CustomErrorMessages;
import com.adobe.pdfservices.operation.internal.constants.PDFServicesURI;
import com.adobe.pdfservices.operation.internal.util.JsonUtil;
import com.adobe.pdfservices.operation.internal.util.ObjectUtil;
import com.adobe.pdfservices.operation.internal.util.StringUtil;
import com.fasterxml.jackson.databind.JsonNode;
import java.io.File;
import java.io.IOException;
/**
* Encapsulates the API request configurations.
*/
public class ClientConfig {
private static final String PDF_SERVICES = "pdf_services";
private static final String PDF_SERVICES_URI = "pdf_services_uri";
private static final String CONNECT_TIMEOUT_KEY = "connectTimeout";
private static final String SOCKET_TIMEOUT_KEY = "socketTimeout";
private static final String PROXY_HOST = "host";
private static final String PROXY_SERVER_CONFIG = "proxyServerConfig";
private static final String PROXY_PORT = "port";
private static final String PROXY_SCHEME = "proxyScheme";
private static final String REGION = "region";
private static final String PROXY_CREDENTIALS = "usernamePasswordCredentials";
private static final String PROXY_USERNAME = "username";
private static final String PROXY_PASSWORD = "password";
/**
* Creates a new {@code ClientConfig} builder.
*
* @return a {@code ClientConfig.Builder} instance.
*/
public static Builder builder() {
return new Builder();
}
/**
* Builds a {@link ClientConfig} instance.
*/
public static class Builder {
private Integer connectTimeout;
private Integer socketTimeout;
private String pdfServicesUri;
private ProxyServerConfig proxyServerConfig;
/**
* Constructs a {@code Builder} instance.
*/
public Builder() {
}
/**
* Sets the region code.
*
* @param region a {@link Region} instance. Default value is US.
* @return this Builder instance to add any additional parameters
*/
public Builder setRegion(Region region) {
ObjectUtil.requireNonNull(region, String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL, "Region"));
this.pdfServicesUri = PDFServicesURI.getURIForRegion(region);
return this;
}
/**
* Sets the connect timeout. It should be greater than zero.
*
* @param connectTimeout determines the timeout in milliseconds until a connection is established in the API
* calls. Default value is 10000 milliseconds.
* @return this Builder instance to add any additional parameters
*/
public Builder withConnectTimeout(Integer connectTimeout) {
this.connectTimeout = connectTimeout;
return this;
}
/**
* Sets the configuration for proxy server.
*
* @param proxyServerConfig a {@link ProxyServerConfig} instance for providing proxy server configuration.
* @return this Builder instance to add any additional parameters
*/
public Builder withProxyServerConfig(ProxyServerConfig proxyServerConfig) {
ObjectUtil.requireNonNull(proxyServerConfig, String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL,
"Proxy Server Config"));
this.proxyServerConfig = proxyServerConfig;
return this;
}
/**
* Sets the socket timeout. It should be greater than zero.
*
* @param socketTimeout Defines the socket timeout in milliseconds, which is the timeout for waiting for data
* or,
* put differently, a maximum period inactivity between two consecutive data packets.
* Default value is 2000 milliseconds
* @return this Builder instance to add any additional parameters
*/
public Builder withSocketTimeout(Integer socketTimeout) {
ObjectUtil.requireNonNull(socketTimeout, String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL,
"Socket Timeout"));
this.socketTimeout = socketTimeout;
return this;
}
/**
* Sets the connect timeout, socket timeout, processing timeout and proxy server config using the JSON client
* config file path.
* Default value for socket timeout is 2000 milliseconds, for connect timeout is 10000 milliseconds
* and for processing timeout is 600000 milliseconds (10 minutes).
*
* JSON structure:
*
* {
* "connectTimeout": "4000",
* "socketTimeout": "20000",
* "proxyServerConfig": {
* "host": "127.0.0.1",
* "port": "8080",
* "scheme": "https",
* "usernamePasswordCredentials": {
* "username": "username",
* "password": "password"
* }
* },
* "region": "EU"
* }
*
*
* @return this Builder instance to add any additional parameters
*/
public Builder fromFile(String clientConfigFilePath) {
try {
JsonNode clientConfig = JsonUtil.readJsonTreeFromFile(new File(clientConfigFilePath));
JsonNode regionNode = clientConfig.get(ClientConfig.REGION);
if (regionNode != null) {
this.pdfServicesUri = PDFServicesURI.getURIForRegion(Region.get(regionNode.asText()));
}
JsonNode pdfServicesConfig = clientConfig.get(ClientConfig.PDF_SERVICES);
if (pdfServicesConfig != null) {
JsonNode pdfServicesUriNode = pdfServicesConfig.get(ClientConfig.PDF_SERVICES_URI);
if (pdfServicesUriNode != null) {
this.pdfServicesUri = pdfServicesUriNode.asText();
}
}
JsonNode connectTimeoutNode = clientConfig.get(ClientConfig.CONNECT_TIMEOUT_KEY);
if (connectTimeoutNode != null) {
if (StringUtil.isPositiveInteger(connectTimeoutNode.asText())) {
this.connectTimeout = connectTimeoutNode.asInt();
} else {
throw new IllegalArgumentException(String.format("Invalid value for connect timeout %s Must " +
"be valid integer greater than 0",
connectTimeoutNode.asText()));
}
}
JsonNode socketTimeoutNode = clientConfig.get(ClientConfig.SOCKET_TIMEOUT_KEY);
if (socketTimeoutNode != null) {
if (StringUtil.isPositiveInteger(socketTimeoutNode.asText())) {
this.socketTimeout = socketTimeoutNode.asInt();
} else {
throw new IllegalArgumentException(String.format("Invalid value for socket timeout %s. Must " +
"be valid integer greater than 0",
socketTimeoutNode.asText()));
}
}
this.proxyServerConfig = createProxyServerConfig(clientConfig);
return this;
} catch (IOException ioException) {
throw new IllegalArgumentException("Invalid Client config file provided.");
}
}
/**
* Builds configuration for Proxy server, includes configuration for authentication at proxy server.
*
* @param clientConfig determines configuration provided from config file.
*/
private ProxyServerConfig createProxyServerConfig(JsonNode clientConfig) {
JsonNode proxyServerConfigNode = clientConfig.get(ClientConfig.PROXY_SERVER_CONFIG);
if (proxyServerConfigNode == null) {
return null;
}
ProxyServerConfig.Builder proxyServerConfigBuilder = new ProxyServerConfig.Builder();
JsonNode proxyHostNode = proxyServerConfigNode.get(ClientConfig.PROXY_HOST);
if (validateJsonNode(proxyHostNode)) {
proxyServerConfigBuilder.withHost(proxyHostNode.asText());
} else {
throw new IllegalArgumentException(String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL_OR_EMPTY
, "Proxy host"));
}
JsonNode proxyPortNode = proxyServerConfigNode.get(ClientConfig.PROXY_PORT);
if (proxyPortNode != null) {
if (StringUtil.isPositiveInteger(proxyPortNode.asText())) {
proxyServerConfigBuilder.withPort(proxyPortNode.asInt());
} else {
throw new IllegalArgumentException("Invalid value for proxy port. Must be a valid integer greater" +
" than 0");
}
}
JsonNode proxySchemeNode = proxyServerConfigNode.get(PROXY_SCHEME);
if (proxySchemeNode != null) {
if (validateJsonNode(proxySchemeNode)) {
proxyServerConfigBuilder.withProxyScheme(ProxyScheme.get(proxySchemeNode.asText()));
} else {
throw new IllegalArgumentException(String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL_OR_EMPTY, "Proxy scheme"));
}
}
JsonNode credentialsNode = proxyServerConfigNode.get(PROXY_CREDENTIALS);
if (credentialsNode != null) {
JsonNode userName = credentialsNode.get(PROXY_USERNAME);
JsonNode password = credentialsNode.get(PROXY_PASSWORD);
if (!validateJsonNode(userName)) {
throw new IllegalArgumentException(String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL_OR_EMPTY, "Username"));
}
if (!validateJsonNode(password)) {
throw new IllegalArgumentException(String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL_OR_EMPTY, "Password"));
}
UsernamePasswordCredentials usernamePasswordCredentials =
new UsernamePasswordCredentials(userName.asText(), password.asText());
proxyServerConfigBuilder.withCredentials(usernamePasswordCredentials);
}
return proxyServerConfigBuilder.build();
}
private boolean validateJsonNode(JsonNode node) {
return node != null && !node.isNull() && StringUtil.isNotBlank(node.asText());
}
/**
* Returns a new {@link ClientConfig} instance built from the current state of this builder.
*
* @return a new {@code ClientConfig} instance.
*/
public ClientConfig build() {
return new InternalClientConfig(this.connectTimeout, this.socketTimeout, this.pdfServicesUri,
this.proxyServerConfig);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy