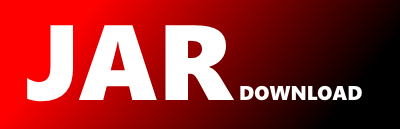
com.adobe.pdfservices.operation.config.proxy.ProxyServerConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdfservices-sdk Show documentation
Show all versions of pdfservices-sdk Show documentation
Adobe PDF Services SDK allows you to access RESTful APIs to create, convert, and manipulate PDFs within your applications.
Older versions can be found under groupId: com.adobe.documentservices, artifactId: pdftools-sdk
/*
* Copyright 2024 Adobe
* All Rights Reserved.
*
* NOTICE: Adobe permits you to use, modify, and distribute this file in
* accordance with the terms of the Adobe license agreement accompanying
* it. If you have received this file from a source other than Adobe,
* then your use, modification, or distribution of it requires the prior
* written permission of Adobe.
*/
package com.adobe.pdfservices.operation.config.proxy;
import com.adobe.pdfservices.operation.internal.GlobalConfig;
import com.adobe.pdfservices.operation.internal.constants.CustomErrorMessages;
import com.adobe.pdfservices.operation.internal.util.ObjectUtil;
/**
* Encapsulates the proxy server configurations.
*/
public class ProxyServerConfig {
private final String host;
private final Integer port;
private final ProxyScheme scheme;
private final ProxyAuthenticationCredentials credentials;
private ProxyServerConfig(String host, Integer port, ProxyScheme scheme,
ProxyAuthenticationCredentials credentials) {
this.host = host;
this.port = (port == null) ? GlobalConfig.getProxyPort() : port;
this.scheme = scheme;
this.credentials = credentials;
}
/**
* Creates a new {@code ProxyServerConfig} builder.
*
* @return a {@code ProxyServerConfig.Builder} instance
*/
public static Builder builder() {
return new Builder();
}
/**
* Gets the host name of proxy server.
*
* @return A String denoting host
*/
public String getHost() {
return host;
}
/**
* Gets the port number of proxy server.
*
* @return A String denoting port
*/
public Integer getPort() {
return (port != null) ? port : GlobalConfig.getProxyPort();
}
/**
* Gets the scheme of proxy server.
*
* @return An instance {@link ProxyScheme}
*/
public ProxyScheme getProxyScheme() {
return scheme;
}
/**
* Gets the credentials for authenticating with a proxy server.
*
* @return An instance {@link ProxyAuthenticationCredentials}
*/
public ProxyAuthenticationCredentials getCredentials() {
return credentials;
}
/**
* Builds a {@link ProxyServerConfig} instance.
*/
public static class Builder {
public String host;
public Integer port;
public ProxyScheme scheme;
public ProxyAuthenticationCredentials credentials;
/**
* Constructs a {@code Builder} instance.
*/
public Builder() {
}
/**
* Sets the host name of proxy server.
*
* @param host Host name. Cannot be null or empty
* @return this Builder instance to add any additional parameters
*/
public Builder withHost(String host) {
ObjectUtil.requireNonNull(host, String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL, "Host"));
this.host = host;
return this;
}
/**
* Sets the port number of proxy server. It should be greater than 0.
* Scheme's default port is used if not provided.
*
* @param port Port number; can not be null.
* @return this Builder instance to add any additional parameters
*/
public Builder withPort(Integer port) {
ObjectUtil.requireNonNull(port, String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL, "Port"));
this.port = port;
return this;
}
/**
* Sets the scheme of proxy server. Possible values are HTTP and HTTPS. Default value is HTTP.
*
* @param proxyScheme Proxy scheme. See {@link ProxyScheme} for scheme values; can not be null.
* @return this Builder instance to add any additional parameters
*/
public Builder withProxyScheme(ProxyScheme proxyScheme) {
ObjectUtil.requireNonNull(proxyScheme, String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL, "Proxy " +
"Scheme"));
this.scheme = proxyScheme;
return this;
}
/**
* Sets the credentials for authenticating with a proxy server.
*
* @param credentials a {@link ProxyAuthenticationCredentials} instance; can not be null.
* @return this Builder instance to add any additional parameters
*/
public Builder withCredentials(ProxyAuthenticationCredentials credentials) {
ObjectUtil.requireNonNull(credentials, String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL, "Proxy " +
"Authentication Credentials"));
this.credentials = credentials;
return this;
}
/**
* Returns a new {@link ProxyServerConfig} instance built from the current state of this builder.
*
* @return a new {@code ProxyServerConfig} instance
*/
public ProxyServerConfig build() {
return new ProxyServerConfig(this.host, this.port, this.scheme, this.credentials);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy