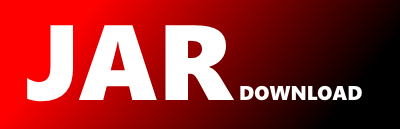
com.adobe.pdfservices.operation.internal.InternalClientConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdfservices-sdk Show documentation
Show all versions of pdfservices-sdk Show documentation
Adobe PDF Services SDK allows you to access RESTful APIs to create, convert, and manipulate PDFs within your applications.
Older versions can be found under groupId: com.adobe.documentservices, artifactId: pdftools-sdk
/*
* Copyright 2024 Adobe
* All Rights Reserved.
*
* NOTICE: Adobe permits you to use, modify, and distribute this file in
* accordance with the terms of the Adobe license agreement accompanying
* it. If you have received this file from a source other than Adobe,
* then your use, modification, or distribution of it requires the prior
* written permission of Adobe.
*/
package com.adobe.pdfservices.operation.internal;
import com.adobe.pdfservices.operation.config.ClientConfig;
import com.adobe.pdfservices.operation.config.proxy.ProxyServerConfig;
import com.adobe.pdfservices.operation.config.proxy.UsernamePasswordCredentials;
import com.adobe.pdfservices.operation.internal.constants.CustomErrorMessages;
import com.adobe.pdfservices.operation.internal.constants.PDFServicesURI;
import com.adobe.pdfservices.operation.internal.util.StringUtil;
public class InternalClientConfig extends ClientConfig {
private Integer connectTimeout;
private Integer socketTimeout;
private String pdfServiceUri;
private ProxyServerConfig proxyServerConfig;
public InternalClientConfig(Integer connectTimeout, Integer socketTimeout, String pdfServiceUri,
ProxyServerConfig proxyServerConfig) {
this.connectTimeout = connectTimeout != null ? connectTimeout : GlobalConfig.getConnectTimeout();
this.socketTimeout = socketTimeout != null ? socketTimeout : GlobalConfig.getSocketTimeout();
this.pdfServiceUri = pdfServiceUri != null ? pdfServiceUri : PDFServicesURI.getDefaultURI();
this.proxyServerConfig = proxyServerConfig;
}
public InternalClientConfig() {
this.connectTimeout = GlobalConfig.getConnectTimeout();
this.socketTimeout = GlobalConfig.getSocketTimeout();
this.pdfServiceUri = PDFServicesURI.getDefaultURI();
}
public Integer getConnectTimeout() {
return connectTimeout;
}
public Integer getSocketTimeout() {
return socketTimeout;
}
public ProxyServerConfig getProxyServerConfig() {
return proxyServerConfig;
}
public void validate() {
if (this.socketTimeout <= 0) {
throw new IllegalArgumentException(String.format("Invalid value for socket timeout %s Must be valid " +
"integer greater than 0", this.socketTimeout));
}
if (this.connectTimeout <= 0) {
throw new IllegalArgumentException(String.format("Invalid value for connect timeout %s Must be valid " +
"integer greater than 0", this.connectTimeout));
}
if (proxyServerConfig != null) {
if (this.proxyServerConfig.getPort() <= 0 && !this.proxyServerConfig.getPort()
.equals(GlobalConfig.getProxyPort())) {
throw new IllegalArgumentException("Invalid value for proxy port. Must be valid integer greater than " +
"0");
}
if (StringUtil.isBlank(this.proxyServerConfig.getHost())) {
throw new IllegalArgumentException(String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL_OR_EMPTY
, "Proxy host"));
}
UsernamePasswordCredentials credentials =
(UsernamePasswordCredentials) this.proxyServerConfig.getCredentials();
if (credentials != null && StringUtil.isBlank(credentials.getUsername())) {
throw new IllegalArgumentException(String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL_OR_EMPTY
, "Username"));
}
if (credentials != null && StringUtil.isBlank(credentials.getPassword())) {
throw new IllegalArgumentException(String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL_OR_EMPTY
, "Password"));
}
}
}
public String getPdfServiceUri() {
return pdfServiceUri;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy