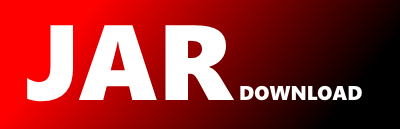
com.adobe.pdfservices.operation.internal.PDFServicesHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdfservices-sdk Show documentation
Show all versions of pdfservices-sdk Show documentation
Adobe PDF Services SDK allows you to access RESTful APIs to create, convert, and manipulate PDFs within your applications.
Older versions can be found under groupId: com.adobe.documentservices, artifactId: pdftools-sdk
/*
* Copyright 2024 Adobe
* All Rights Reserved.
*
* NOTICE: Adobe permits you to use, modify, and distribute this file in
* accordance with the terms of the Adobe license agreement accompanying
* it. If you have received this file from a source other than Adobe,
* then your use, modification, or distribution of it requires the prior
* written permission of Adobe.
*/
package com.adobe.pdfservices.operation.internal;
import com.adobe.pdfservices.operation.PDFServicesJobStatus;
import com.adobe.pdfservices.operation.PDFServicesJobStatusResponse;
import com.adobe.pdfservices.operation.PDFServicesResponse;
import com.adobe.pdfservices.operation.exception.SDKException;
import com.adobe.pdfservices.operation.exception.ServiceApiException;
import com.adobe.pdfservices.operation.exception.ServiceUsageException;
import com.adobe.pdfservices.operation.internal.constants.OperationHeaderInfoEndpointMap;
import com.adobe.pdfservices.operation.internal.constants.RequestKey;
import com.adobe.pdfservices.operation.internal.dto.request.PlatformApiRequest;
import com.adobe.pdfservices.operation.internal.dto.request.upload.AssetUploadURIRequest;
import com.adobe.pdfservices.operation.internal.dto.response.AssetDownloadUriResponse;
import com.adobe.pdfservices.operation.internal.dto.response.PlatformApiAutotagResponse;
import com.adobe.pdfservices.operation.internal.dto.response.PlatformApiExtractResponse;
import com.adobe.pdfservices.operation.internal.dto.response.PlatformApiJobStatusResponse;
import com.adobe.pdfservices.operation.internal.dto.response.PlatformApiMultiAssetResponse;
import com.adobe.pdfservices.operation.internal.dto.response.PlatformApiPDFPropertiesResponse;
import com.adobe.pdfservices.operation.internal.dto.response.PlatformApiSingleAssetResponse;
import com.adobe.pdfservices.operation.internal.dto.response.error.JobErrorResponse;
import com.adobe.pdfservices.operation.internal.dto.response.pdfproperties.Metadata;
import com.adobe.pdfservices.operation.internal.dto.response.upload.AssetUploadURIResponse;
import com.adobe.pdfservices.operation.internal.exception.OperationException;
import com.adobe.pdfservices.operation.internal.http.BaseHttpRequest;
import com.adobe.pdfservices.operation.internal.http.DefaultRequestHeaders;
import com.adobe.pdfservices.operation.internal.http.HttpClient;
import com.adobe.pdfservices.operation.internal.http.HttpClientFactory;
import com.adobe.pdfservices.operation.internal.http.HttpResponse;
import com.adobe.pdfservices.operation.internal.util.AssetUploadUtil;
import com.adobe.pdfservices.operation.internal.util.JsonUtil;
import com.adobe.pdfservices.operation.internal.util.ValidationUtil;
import com.adobe.pdfservices.operation.io.Asset;
import com.adobe.pdfservices.operation.io.CloudAsset;
import com.adobe.pdfservices.operation.io.StreamAsset;
import com.adobe.pdfservices.operation.pdfjobs.result.AutotagPDFResult;
import com.adobe.pdfservices.operation.pdfjobs.result.CombinePDFResult;
import com.adobe.pdfservices.operation.pdfjobs.result.CompressPDFResult;
import com.adobe.pdfservices.operation.pdfjobs.result.CreatePDFResult;
import com.adobe.pdfservices.operation.pdfjobs.result.DeletePagesResult;
import com.adobe.pdfservices.operation.pdfjobs.result.DocumentMergeResult;
import com.adobe.pdfservices.operation.pdfjobs.result.ExportPDFResult;
import com.adobe.pdfservices.operation.pdfjobs.result.ExportPDFToImagesResult;
import com.adobe.pdfservices.operation.pdfjobs.result.ExtractPDFResult;
import com.adobe.pdfservices.operation.pdfjobs.result.HTMLToPDFResult;
import com.adobe.pdfservices.operation.pdfjobs.result.InsertPagesResult;
import com.adobe.pdfservices.operation.pdfjobs.result.LinearizePDFResult;
import com.adobe.pdfservices.operation.pdfjobs.result.OCRResult;
import com.adobe.pdfservices.operation.pdfjobs.result.PDFElectronicSealResult;
import com.adobe.pdfservices.operation.pdfjobs.result.PDFPropertiesResult;
import com.adobe.pdfservices.operation.pdfjobs.result.PDFServicesJobResult;
import com.adobe.pdfservices.operation.pdfjobs.result.ProtectPDFResult;
import com.adobe.pdfservices.operation.pdfjobs.result.RemoveProtectionResult;
import com.adobe.pdfservices.operation.pdfjobs.result.ReorderPagesResult;
import com.adobe.pdfservices.operation.pdfjobs.result.ReplacePagesResult;
import com.adobe.pdfservices.operation.pdfjobs.result.RotatePagesResult;
import com.adobe.pdfservices.operation.pdfjobs.result.SplitPDFResult;
import com.adobe.pdfservices.operation.pdfjobs.result.pdfproperties.PDFProperties;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.DeserializationFeature;
import com.fasterxml.jackson.databind.ObjectMapper;
import org.apache.commons.io.IOUtils;
import org.apache.http.HttpHeaders;
import org.json.JSONObject;
import org.json.JSONTokener;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.IOException;
import java.io.InputStream;
import java.lang.reflect.InvocationTargetException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.UUID;
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.Future;
import java.util.concurrent.TimeUnit;
public class PDFServicesHelper {
private static final Logger LOGGER = LoggerFactory.getLogger(PDFServicesHelper.class);
private static final String OPERATION = "/operation/";
private static final String ASSETS = "/assets";
private static final String CONTENT_TYPE_JSON = "application/json";
public static Asset upload(ExecutionContext context, InputStream inputStream, String mediaType) throws ServiceApiException {
LOGGER.info("Started uploading asset");
ValidationUtil.validateExecutionContext(context);
//generating x-request-id
String xRequestId = UUID.randomUUID().toString();
LOGGER.debug("Uploading asset with request id {}", xRequestId);
AssetUploadURIRequest assetUploadURIRequest = new AssetUploadURIRequest(mediaType);
ObjectMapper objectMapper = new ObjectMapper();
String requestBody;
try {
requestBody = objectMapper.writeValueAsString(assetUploadURIRequest);
} catch (JsonProcessingException e) {
throw new SDKException("Error occurred while creating request", e);
}
HttpResponse getUploadURIResponse = createPreSignedUri(context, requestBody,
xRequestId);
uploadAsset(context, getUploadURIResponse.getBody().getUploadUri(), inputStream, mediaType);
LOGGER.info("Finished uploading asset");
return new CloudAsset(getUploadURIResponse.getBody().getAssetID(), null);
}
public static List uploadAssets(ExecutionContext context, List streamAssetList) throws ServiceApiException {
LOGGER.info("Started uploading assets");
ValidationUtil.validateExecutionContext(context);
List assets = new ArrayList<>();
// parallelize the uploads
ExecutorService executor = Executors.newFixedThreadPool(10);
List> callablesTasks = new ArrayList<>();
for (StreamAsset streamAsset : streamAssetList) {
Callable task = new AssetUploadUtil(context, streamAsset);
callablesTasks.add(task);
}
List> futuresResults;
try {
futuresResults = executor.invokeAll(callablesTasks);
} catch (InterruptedException e) {
throw new SDKException("Error occurred while uploading.");
}
for (Future futureResult : futuresResults) {
try {
assets.add(futureResult.get(5, TimeUnit.MINUTES));
} catch (ExecutionException e) {
if (e.getCause() instanceof ServiceApiException) {
throw (ServiceApiException) e.getCause();
}
if (e.getCause() instanceof SDKException) {
throw (SDKException) e.getCause();
}
if (e.getCause() instanceof OperationException) {
OperationException oe = (OperationException) e.getCause();
throw new ServiceApiException(oe.getErrorMessage(), oe.getRequestTrackingId(), oe.getStatusCode()
, oe.getReportErrorCode());
}
if (e.getCause() instanceof ServiceUsageException) {
throw (ServiceUsageException) e.getCause();
}
throw new ServiceApiException("Error occurred while uploading.");
} catch (Exception e) {
throw new SDKException("Error occurred while uploading.");
} finally {
executor.shutdown();
}
}
executor.shutdown();
// end of parallelize
LOGGER.info("Finished uploading assets");
return assets;
}
public static HttpResponse submitJob(ExecutionContext context, PlatformApiRequest platformApiRequest,
String xRequestId,
OperationHeaderInfoEndpointMap operationHeaderInfoEndpointMap) throws ServiceApiException {
LOGGER.info("Started submitting {} job", operationHeaderInfoEndpointMap.getHeaderInfo());
LOGGER.debug("Submitting {} job with request id {}", operationHeaderInfoEndpointMap.getHeaderInfo(),
xRequestId);
try {
HttpClient httpClient = HttpClientFactory.getDefaultHttpClient();
String requestBody = getRequestBodyAsString(platformApiRequest);
BaseHttpRequest baseHttpRequest =
(BaseHttpRequest) context.getBaseRequestFromRequestContext(RequestKey.PLATFORM);
baseHttpRequest.withTemplate(baseHttpRequest.getTemplateString() + OPERATION + operationHeaderInfoEndpointMap.getEndpoint())
.withHeader(DefaultRequestHeaders.DC_REQUEST_ID_HEADER_KEY, xRequestId)
.withHeader(DefaultRequestHeaders.X_DCSDK_OPS_INFO_HEADER_NAME,
operationHeaderInfoEndpointMap.getHeaderInfo())
.withBody(requestBody).withContentType(CONTENT_TYPE_JSON);
LOGGER.info("Finished submitting {} job", operationHeaderInfoEndpointMap.getHeaderInfo());
return httpClient.send(baseHttpRequest, String.class);
} catch (OperationException oe) {
throw new ServiceApiException(oe.getErrorMessage(), oe.getRequestTrackingId(), oe.getStatusCode(),
oe.getReportErrorCode());
}
}
public static PDFServicesResponse getJobResult(ExecutionContext context, String location,
Class responseType) throws ServiceApiException {
LOGGER.info("Started getting job result");
ValidationUtil.validateExecutionContext(context);
PDFServicesResponse pdfServicesResponse = null;
while (pdfServicesResponse == null || PDFServicesJobStatus.IN_PROGRESS.getValue()
.equals(pdfServicesResponse.getStatus())) {
pdfServicesResponse = pollJob(context, location, responseType);
Integer retryAfter = pdfServicesResponse.getRetryInterval();
try {
LOGGER.debug("Retry polling for job result after {} seconds", retryAfter);
TimeUnit.SECONDS.sleep(retryAfter);
} catch (InterruptedException e) {
throw new SDKException("Thread interrupted while waiting for operation execution status!!", e);
}
}
LOGGER.info("Finished getting job result");
return pdfServicesResponse;
}
private static PDFServicesResponse pollJob(ExecutionContext context, String location,
Class resultClass) throws ServiceApiException {
ValidationUtil.validateExecutionContext(context);
//generating x-request-id
String xRequestId = UUID.randomUUID().toString();
LOGGER.debug("Started polling for status with request id {}", xRequestId);
BaseHttpRequest baseHttpRequest = (BaseHttpRequest) context.getBaseRequestFromRequestContext(RequestKey.STATUS);
baseHttpRequest.withHeader(DefaultRequestHeaders.DC_REQUEST_ID_HEADER_KEY, xRequestId).withTemplate(location);
HttpClient httpClient = HttpClientFactory.getDefaultHttpClient();
T result = null;
String status;
Map headers;
JobErrorResponse errorResponse;
try {
if (getSingleAssetResultClasses().contains(resultClass)) {
HttpResponse response = httpClient.send(baseHttpRequest,
PlatformApiSingleAssetResponse.class);
status = response.getBaseResponseDto().getStatus();
headers = response.getHeaders();
result = PDFServicesJobStatus.DONE.getValue().equals(status) ? resultClass.getConstructor(Asset.class)
.newInstance(response.getBaseResponseDto().getCloudAsset()) : null;
errorResponse = PDFServicesJobStatus.FAILED.getValue().equals(status) ? response.getBaseResponseDto()
.getErrorResponse() : null;
} else if (AutotagPDFResult.class.equals(resultClass)) {
HttpResponse response = httpClient.send(baseHttpRequest,
PlatformApiAutotagResponse.class);
status = response.getBaseResponseDto().getStatus();
headers = response.getHeaders();
result = PDFServicesJobStatus.DONE.getValue()
.equals(status) ? resultClass.getConstructor(Asset.class, Asset.class, Asset.class)
.newInstance(response.getBaseResponseDto().getTaggedPDF(),
response.getBaseResponseDto().getReport(),
response.getBaseResponseDto().getResource()) : null;
errorResponse = PDFServicesJobStatus.FAILED.getValue().equals(status) ? response.getBaseResponseDto()
.getErrorResponse() : null;
} else if (ExportPDFToImagesResult.class.equals(resultClass)) {
HttpResponse response = httpClient.send(baseHttpRequest,
PlatformApiMultiAssetResponse.class);
status = response.getBaseResponseDto().getStatus();
headers = response.getHeaders();
result = PDFServicesJobStatus.DONE.getValue().equals(status) ? resultClass.getConstructor(List.class)
.newInstance(response.getBaseResponseDto().getCloudAssets()) : null;
errorResponse = PDFServicesJobStatus.FAILED.getValue().equals(status) ? response.getBaseResponseDto()
.getErrorResponse() : null;
} else if (SplitPDFResult.class.equals(resultClass)) {
HttpResponse response = httpClient.send(baseHttpRequest,
PlatformApiMultiAssetResponse.class);
status = response.getBaseResponseDto().getStatus();
headers = response.getHeaders();
result = PDFServicesJobStatus.DONE.getValue().equals(status) ? resultClass.getConstructor(List.class, Asset.class)
.newInstance(response.getBaseResponseDto().getCloudAssets(), response.getBaseResponseDto().getCloudAsset()) : null;
errorResponse = PDFServicesJobStatus.FAILED.getValue().equals(status) ? response.getBaseResponseDto()
.getErrorResponse() : null;
} else if (ExtractPDFResult.class.equals(resultClass)) {
HttpResponse response = httpClient.send(baseHttpRequest,
PlatformApiExtractResponse.class);
status = response.getBaseResponseDto().getStatus();
headers = response.getHeaders();
JSONObject contentJSON = !Objects.isNull(response.getBaseResponseDto().getContent()) ?
fetchExtractContentJSON(context, response.getBaseResponseDto().getContent()) : null;
result = PDFServicesJobStatus.DONE.getValue()
.equals(status) ? resultClass.getConstructor(Asset.class, Asset.class, JSONObject.class)
.newInstance(response.getBaseResponseDto().getContent(),
response.getBaseResponseDto().getResource(),
contentJSON) : null;
errorResponse = PDFServicesJobStatus.FAILED.getValue().equals(status) ? response.getBaseResponseDto()
.getErrorResponse() : null;
} else if (PDFPropertiesResult.class.equals(resultClass)) {
HttpResponse response = httpClient.send(baseHttpRequest,
PlatformApiPDFPropertiesResponse.class);
status = response.getBaseResponseDto().getStatus();
headers = response.getHeaders();
if (PDFServicesJobStatus.DONE.getValue().equals(status)) {
Metadata metadata = JsonUtil.deserializeJsonString(response.getBaseResponseDto().getMetadata()
.toString(), Metadata.class);
PDFProperties pdfProperties = getPdfPropertiesObject(metadata);
result = resultClass.getConstructor(PDFProperties.class, String.class)
.newInstance(pdfProperties, response.getBaseResponseDto().getMetadata().toString());
}
errorResponse = PDFServicesJobStatus.FAILED.getValue().equals(status) ? response.getBaseResponseDto()
.getErrorResponse() : null;
} else {
throw new SDKException("No result class found.");
}
} catch (InstantiationException | IllegalAccessException | InvocationTargetException |
NoSuchMethodException e) {
throw new SDKException("Error occurred while polling", e);
} catch (OperationException oe) {
throw new ServiceApiException(oe.getErrorMessage(), oe.getRequestTrackingId(), oe.getStatusCode(),
oe.getReportErrorCode());
}
if (PDFServicesJobStatus.FAILED.getValue().equals(status)) {
String requestId = headers.get("x-request-id");
throw new ServiceApiException(errorResponse.getMessage(), requestId, errorResponse.getStatus(),
errorResponse.getCode());
}
LOGGER.debug("Finished polling for status");
return new PDFServicesResponse<>(status, headers, result);
}
public static PDFServicesJobStatusResponse getJobStatus(ExecutionContext context, String location) throws ServiceApiException {
LOGGER.info("Started getting job status");
ValidationUtil.validateExecutionContext(context);
//generating x-request-id
String xRequestId = UUID.randomUUID().toString();
LOGGER.debug("Getting job status with request id {}", xRequestId);
BaseHttpRequest baseHttpRequest = (BaseHttpRequest) context.getBaseRequestFromRequestContext(RequestKey.STATUS);
baseHttpRequest.withHeader(DefaultRequestHeaders.DC_REQUEST_ID_HEADER_KEY, xRequestId).withTemplate(location);
HttpClient httpClient = HttpClientFactory.getDefaultHttpClient();
HttpResponse response = httpClient.send(baseHttpRequest,
PlatformApiJobStatusResponse.class);
String status = response.getBaseResponseDto().getStatus();
Map headers = response.getHeaders();
JobErrorResponse errorResponse = PDFServicesJobStatus.FAILED.getValue()
.equals(status) ? response.getBaseResponseDto()
.getErrorResponse() : null;
if (PDFServicesJobStatus.FAILED.getValue().equals(status)) {
String requestId = headers.get("x-request-id");
throw new ServiceApiException(errorResponse.getMessage(), requestId, errorResponse.getStatus(),
errorResponse.getCode());
}
LOGGER.info("Finished getting job status");
return new PDFServicesJobStatusResponse(status, headers);
}
public static StreamAsset getContent(ExecutionContext context, Asset asset) {
LOGGER.info("Started getting content");
ValidationUtil.validateExecutionContext(context);
String downloadURI = ((CloudAsset) asset).getDownloadURI();
LOGGER.debug("Getting content for asset id {}", ((CloudAsset) asset).getAssetId());
try {
HttpClient httpClient = HttpClientFactory.getDefaultHttpClient();
BaseHttpRequest baseHttpRequest =
(BaseHttpRequest) context.getUnauthenticatedBaseRequestFromRequestContext(RequestKey.DOWNLOAD);
baseHttpRequest.withTemplate(downloadURI);
HttpResponse response = httpClient.send(baseHttpRequest, String.class);
LOGGER.info("Finished getting content");
return new StreamAsset(response.getResponseContent(), response.getHeaders().get(HttpHeaders.CONTENT_TYPE));
} catch (Exception e) {
throw new SDKException("Error occurred while downloading the asset", e);
}
}
public static void deleteAsset(ExecutionContext context, Asset asset) throws ServiceApiException {
LOGGER.info("Started deleting asset");
ValidationUtil.validateExecutionContext(context);
if (!(asset instanceof CloudAsset)) {
throw new SDKException("Only internal storage is supported for delete asset.");
}
//generating x-request-id
String xRequestId = UUID.randomUUID().toString();
String assetId = ((CloudAsset) asset).getAssetId();
LOGGER.debug("Deleting asset with asset id {} and request id {}", assetId, xRequestId);
try {
HttpClient httpClient = HttpClientFactory.getDefaultHttpClient();
BaseHttpRequest baseHttpRequest =
(BaseHttpRequest) context.getBaseRequestFromRequestContext(RequestKey.PLATFORM_DELETE_ASSET);
baseHttpRequest.withTemplate(baseHttpRequest.getTemplateString() + ASSETS + "/" + assetId)
.withHeader(DefaultRequestHeaders.DC_REQUEST_ID_HEADER_KEY, xRequestId)
.withContentType(CONTENT_TYPE_JSON);
httpClient.send(baseHttpRequest, String.class);
} catch (OperationException oe) {
throw new ServiceApiException(oe.getErrorMessage(), oe.getRequestTrackingId(), oe.getStatusCode(),
oe.getReportErrorCode());
} catch (Exception e) {
throw new SDKException("Error occurred while deleting the asset", e);
}
LOGGER.info("Finished deleting asset");
}
public static Asset refreshDownloadURI(ExecutionContext context, Asset asset) throws ServiceApiException {
LOGGER.info("Started refreshing asset");
ValidationUtil.validateExecutionContext(context);
if (!(asset instanceof CloudAsset)) {
throw new SDKException("Only internal storage is supported for refreshing download URI.");
}
//generating x-request-id
String xRequestId = UUID.randomUUID().toString();
String assetId = ((CloudAsset) asset).getAssetId();
LOGGER.debug("Refreshing asset with asset id {} and request id {}", assetId, xRequestId);
String downloadUri;
try {
HttpClient httpClient = HttpClientFactory.getDefaultHttpClient();
BaseHttpRequest baseHttpRequest =
(BaseHttpRequest) context.getBaseRequestFromRequestContext(RequestKey.PLATFORM_GET_ASSET);
baseHttpRequest.withTemplate(baseHttpRequest.getTemplateString() + ASSETS + "/" + assetId)
.withHeader(DefaultRequestHeaders.DC_REQUEST_ID_HEADER_KEY, xRequestId)
.withContentType(CONTENT_TYPE_JSON);
HttpResponse response = httpClient.send(baseHttpRequest,
AssetDownloadUriResponse.class);
downloadUri = response.getBaseResponseDto().getDownloadUri();
} catch (OperationException oe) {
throw new ServiceApiException(oe.getErrorMessage(), oe.getRequestTrackingId(), oe.getStatusCode(),
oe.getReportErrorCode());
} catch (Exception e) {
throw new SDKException("Error occurred while refreshing download uri of the asset", e);
}
LOGGER.info("Finished refreshing asset");
return new CloudAsset(assetId, downloadUri);
}
private static HttpResponse createPreSignedUri(ExecutionContext context,
String requestBody, String xRequestId) throws ServiceApiException {
try {
HttpClient httpClient = HttpClientFactory.getDefaultHttpClient();
BaseHttpRequest baseHttpRequest =
(BaseHttpRequest) context.getBaseRequestFromRequestContext(RequestKey.PLATFORM);
baseHttpRequest.withTemplate(baseHttpRequest.getTemplateString() + ASSETS)
.withHeader(DefaultRequestHeaders.DC_REQUEST_ID_HEADER_KEY, xRequestId).withBody(requestBody)
.withContentType(CONTENT_TYPE_JSON);
return httpClient.send(baseHttpRequest, AssetUploadURIResponse.class);
} catch (OperationException oe) {
throw new ServiceApiException(oe.getErrorMessage(), oe.getRequestTrackingId(), oe.getStatusCode(),
oe.getReportErrorCode());
}
}
private static void uploadAsset(ExecutionContext context, String uri, InputStream inputStream, String mediaType) {
try {
HttpClient httpClient = HttpClientFactory.getDefaultHttpClient();
BaseHttpRequest baseHttpRequest =
(BaseHttpRequest) context.getUnauthenticatedBaseRequestFromRequestContext(RequestKey.UPLOAD);
baseHttpRequest.withTemplate(uri).withHeader(HttpHeaders.CONTENT_TYPE, mediaType);
baseHttpRequest.withBody(IOUtils.toByteArray(inputStream));
httpClient.send(baseHttpRequest, String.class);
} catch (IOException io) {
throw new SDKException("Unexpected error while uploading file", io);
}
}
private static String getRequestBodyAsString(PlatformApiRequest platformApiRequest) {
ObjectMapper objectMapper = new ObjectMapper();
try {
return objectMapper.writeValueAsString(platformApiRequest);
} catch (JsonProcessingException e) {
throw new SDKException("Error occurred while creating request", e);
}
}
private static JSONObject fetchExtractContentJSON(ExecutionContext context, Asset asset) {
String downloadURI = ((CloudAsset) asset).getDownloadURI();
try {
HttpClient httpClient = HttpClientFactory.getDefaultHttpClient();
BaseHttpRequest baseHttpRequest =
(BaseHttpRequest) context.getUnauthenticatedBaseRequestFromRequestContext(RequestKey.DOWNLOAD);
baseHttpRequest.withTemplate(downloadURI);
HttpResponse response = httpClient.send(baseHttpRequest, String.class);
return new JSONObject(new JSONTokener(response.getResponseContent()));
} catch (Exception e) {
throw new SDKException("Error occurred while downloading the extract content JSON asset", e);
}
}
private static PDFProperties getPdfPropertiesObject(Metadata metadata) {
ObjectMapper mapper = new ObjectMapper();
mapper.disable(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES);
return mapper.convertValue(metadata, PDFProperties.class);
}
private static List> getSingleAssetResultClasses() {
return Arrays.asList(LinearizePDFResult.class, DocumentMergeResult.class, DeletePagesResult.class,
RotatePagesResult.class, PDFElectronicSealResult.class, CompressPDFResult.class,
CombinePDFResult.class, ExportPDFResult.class, OCRResult.class, ProtectPDFResult.class,
InsertPagesResult.class, ReplacePagesResult.class, ReorderPagesResult.class,
CreatePDFResult.class, HTMLToPDFResult.class, RemoveProtectionResult.class);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy