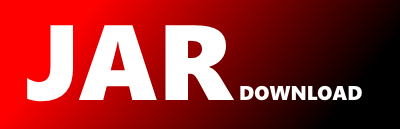
com.adobe.pdfservices.operation.internal.dto.response.pdfproperties.DocumentProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdfservices-sdk Show documentation
Show all versions of pdfservices-sdk Show documentation
Adobe PDF Services SDK allows you to access RESTful APIs to create, convert, and manipulate PDFs within your applications.
Older versions can be found under groupId: com.adobe.documentservices, artifactId: pdftools-sdk
/*
* Copyright 2019 Adobe
* All Rights Reserved.
*
* NOTICE: Adobe permits you to use, modify, and distribute this file in
* accordance with the terms of the Adobe license agreement accompanying
* it. If you have received this file from a source other than Adobe,
* then your use, modification, or distribution of it requires the prior
* written permission of Adobe.
*/
package com.adobe.pdfservices.operation.internal.dto.response.pdfproperties;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.List;
@JsonIgnoreProperties(ignoreUnknown = true)
public class DocumentProperties {
private Boolean isLinearized;
private String pdfeComplianceLevel;
private Boolean isTagged;
private Boolean isPortfolio;
private Boolean isCertified;
private Boolean isEncrypted;
private InfoDictProperties infoDict;
private Boolean isFTPDF;
private String pdfVersion;
private Boolean hasAcroform;
private String fileSize;
private Boolean isSigned;
private Integer incrementalSaveCount;
private Boolean hasEmbeddedFiles;
private Boolean isXFA;
private List fonts;
private String pdfaComplianceLevel;
private String pdfvtComplianceLevel;
private String pdfxComplianceLevel;
private String pdfuaComplianceLevel;
private String xmp;
private Integer pageCount;
@JsonCreator
public DocumentProperties(@JsonProperty("is_linearized") Boolean isLinearized, @JsonProperty(
"pdfe_compliance_level") String pdfeComplianceLevel, @JsonProperty("is_tagged") Boolean isTagged,
@JsonProperty("is_portfolio") Boolean isPortfolio,
@JsonProperty("is_certified") Boolean isCertified,
@JsonProperty("is_encrypted") Boolean isEncrypted,
@JsonProperty("info_dict") InfoDictProperties infoDict,
@JsonProperty("is_FTPDF") Boolean isFTPDF,
@JsonProperty("pdf_version") String pdfVersion,
@JsonProperty("has_acroform") Boolean hasAcroform,
@JsonProperty("file_size") String fileSize, @JsonProperty("is_signed") Boolean isSigned
, @JsonProperty("incremental_save_count") Integer incrementalSaveCount, @JsonProperty("has_embedded_files"
) Boolean hasEmbeddedFiles, @JsonProperty("is_XFA") Boolean isXFA,
@JsonProperty("fonts") List fonts, @JsonProperty("pdfa_compliance_level"
) String pdfaComplianceLevel, @JsonProperty("pdfvt_compliance_level") String pdfvtComplianceLevel, @JsonProperty(
"pdfx_compliance_level") String pdfxComplianceLevel,
@JsonProperty("pdfua_compliance_level") String pdfuaComplianceLevel, @JsonProperty("XMP"
) String xmp, @JsonProperty("page_count") Integer pageCount) {
this.isLinearized = isLinearized;
this.pdfeComplianceLevel = pdfeComplianceLevel;
this.isTagged = isTagged;
this.isPortfolio = isPortfolio;
this.isCertified = isCertified;
this.isEncrypted = isEncrypted;
this.infoDict = infoDict;
this.isFTPDF = isFTPDF;
this.pdfVersion = pdfVersion;
this.hasAcroform = hasAcroform;
this.fileSize = fileSize;
this.isSigned = isSigned;
this.incrementalSaveCount = incrementalSaveCount;
this.hasEmbeddedFiles = hasEmbeddedFiles;
this.isXFA = isXFA;
this.fonts = fonts;
this.pdfaComplianceLevel = pdfaComplianceLevel;
this.pdfvtComplianceLevel = pdfvtComplianceLevel;
this.pdfxComplianceLevel = pdfxComplianceLevel;
this.pdfuaComplianceLevel = pdfuaComplianceLevel;
this.xmp = xmp;
this.pageCount = pageCount;
}
public Boolean getLinearized() {
return isLinearized;
}
public String getPdfeComplianceLevel() {
return pdfeComplianceLevel;
}
public Boolean getTagged() {
return isTagged;
}
public Boolean getPortfolio() {
return isPortfolio;
}
public Boolean getCertified() {
return isCertified;
}
public Boolean getEncrypted() {
return isEncrypted;
}
public InfoDictProperties getInfoDict() {
return infoDict;
}
public Boolean getFTPDF() {
return isFTPDF;
}
public String getPdfVersion() {
return pdfVersion;
}
public Boolean getHasAcroform() {
return hasAcroform;
}
public String getFileSize() {
return fileSize;
}
public Boolean getSigned() {
return isSigned;
}
public Integer getIncrementalSaveCount() {
return incrementalSaveCount;
}
public Boolean getHasEmbeddedFiles() {
return hasEmbeddedFiles;
}
public Boolean getXFA() {
return isXFA;
}
public List getFonts() {
return fonts;
}
public String getPdfaComplianceLevel() {
return pdfaComplianceLevel;
}
public String getPdfvtComplianceLevel() {
return pdfvtComplianceLevel;
}
public String getPdfxComplianceLevel() {
return pdfxComplianceLevel;
}
public String getPdfuaComplianceLevel() {
return pdfuaComplianceLevel;
}
public String getXmp() {
return xmp;
}
public Integer getPageCount() {
return pageCount;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy