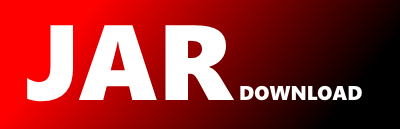
com.adobe.pdfservices.operation.internal.http.BaseHttpRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdfservices-sdk Show documentation
Show all versions of pdfservices-sdk Show documentation
Adobe PDF Services SDK allows you to access RESTful APIs to create, convert, and manipulate PDFs within your applications.
Older versions can be found under groupId: com.adobe.documentservices, artifactId: pdftools-sdk
/*
* Copyright 2019 Adobe
* All Rights Reserved.
*
* NOTICE: Adobe permits you to use, modify, and distribute this file in
* accordance with the terms of the Adobe license agreement accompanying
* it. If you have received this file from a source other than Adobe,
* then your use, modification, or distribution of it requires the prior
* written permission of Adobe.
*/
package com.adobe.pdfservices.operation.internal.http;
import com.adobe.pdfservices.operation.internal.auth.AuthenticationMethod;
import com.adobe.pdfservices.operation.internal.auth.Authenticator;
import com.adobe.pdfservices.operation.internal.auth.SessionToken;
import com.adobe.pdfservices.operation.internal.constants.RequestKey;
import org.apache.http.Consts;
import org.apache.http.HttpEntity;
import org.apache.http.HttpHeaders;
import org.apache.http.NameValuePair;
import org.apache.http.client.entity.EntityBuilder;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.entity.StringEntity;
import java.nio.charset.StandardCharsets;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.stream.Collectors;
public class BaseHttpRequest implements HttpRequest {
private String uriTemplate;
private HttpMethod httpMethod;
private RequestType requestType;
private Map headers;
private AuthenticationMethod authenticationMethod;
private HttpEntity entity;
private Authenticator authenticator;
private RequestKey requestKey;
/**
* Config which deals with http timeouts and request specific params.
*/
private HttpRequestConfig requestConfig;
public BaseHttpRequest(String requestKey, HttpMethod httpMethod, String uriTemplate) {
Objects.requireNonNull(requestKey);
Objects.requireNonNull(httpMethod);
Objects.requireNonNull(uriTemplate);
this.httpMethod = httpMethod;
this.uriTemplate = uriTemplate;
this.headers = new HashMap<>();
this.authenticationMethod = AuthenticationMethod.AUTH_HEADER_PRIMARY;
this.requestType = RequestType.REGULAR;
this.requestKey = RequestKey.getRequestKeyFromValue(requestKey);
}
public BaseHttpRequest(HttpMethod httpMethod) {
Objects.requireNonNull(httpMethod);
this.httpMethod = httpMethod;
this.headers = new HashMap<>();
this.authenticationMethod = AuthenticationMethod.AUTH_HEADER_PRIMARY;
this.requestType = RequestType.REGULAR;
this.requestKey = null;
}
@Override
public HttpRequest withContentType(String contentType) {
return withHeader(HttpHeaders.CONTENT_TYPE, contentType);
}
@Override
public HttpRequest withHeader(String headerName, String headerValue) {
this.headers.put(headerName, headerValue);
return this;
}
/**
* Will overwrite headers as well
*
* @param headers
* @return
*/
@Override
public HttpRequest withHeaders(Map headers) {
Map headersCopy = getHeadersCopy(headers);
this.headers.putAll(headersCopy);
return this;
}
@Override
public HttpRequest withUriParam(String name, String value) {
return null;
}
@Override
public HttpRequest withUrlEncodedFormParams(List formParams) {
this.entity = new UrlEncodedFormEntity(formParams, Consts.UTF_8);
return this;
}
@Override
public HttpRequest withBody(String body) {
this.entity = new StringEntity(body, StandardCharsets.UTF_8);
return this;
}
public HttpRequest withBody(byte[] bytes) {
this.entity = EntityBuilder.create().setBinary(bytes).build();
return this;
}
@Override
public HttpRequest withAuthenticationMethod(AuthenticationMethod authMethod) {
this.authenticationMethod = authMethod;
return this;
}
@Override
public HttpRequest withAuthenticator(Authenticator authenticator) {
this.authenticator = authenticator;
this.headers.put(DefaultRequestHeaders.X_API_KEY_HEADER_NAME, this.authenticator.getClientId());
return this;
}
@Override
public HttpRequest withConfig(HttpRequestConfig requestConfig) {
this.requestConfig = requestConfig;
return this;
}
@Override
public HttpRequest withRequestKey(RequestKey requestKey) {
this.requestKey = requestKey;
return this;
}
@Override
public void authenticate() {
if (authenticationMethod.isAuthRequired()) {
if (authenticator == null) {
throw new IllegalStateException("No authenticator provided for request. Cannot add authorization " +
"headers");
}
SessionToken sessionToken = this.authenticator.getSessionToken(requestConfig);
withHeader(HttpHeaders.AUTHORIZATION, String.format("Bearer %s", sessionToken.getAccessToken()));
}
}
@Override
public void forceAuthenticate() {
if (authenticationMethod.isAuthRequired()) {
if (authenticator == null) {
throw new IllegalStateException("No authenticator provided for request. Cannot add authorization " +
"headers");
}
SessionToken sessionToken = this.authenticator.refreshSessionToken(requestConfig);
withHeader(HttpHeaders.AUTHORIZATION, String.format("Bearer %s", sessionToken.getAccessToken()));
}
}
@Override
public HttpRequestConfig getConfig() {
return requestConfig;
}
@Override
public HttpRequest withTemplate(String uriTemplate) {
this.uriTemplate = uriTemplate;
return this;
}
@Override
public HttpRequest withAcceptType(String acceptHeader) {
return withHeader(HttpHeaders.ACCEPT, acceptHeader);
}
@Override
public StringEntity getEntity() {
return (StringEntity) entity;
}
@Override
public HttpEntity getInputStreamEntity() {
return entity;
}
@Override
public HttpMethod getHttpMethod() {
return httpMethod;
}
@Override
public AuthenticationMethod getAuthMethod() {
return authenticationMethod;
}
@Override
public Map getHeaders() {
return getHeadersCopy(this.headers);
}
@Override
public String getFinalUri() {
return this.uriTemplate;
}
@Override
public String getTemplateString() {
return uriTemplate;
}
@Override
public RequestType getRequestType() {
return requestType;
}
@Override
public Authenticator getAuthenticator() {
return authenticator;
}
private Map getHeadersCopy(Map headersToCopy) {
return headersToCopy.entrySet().stream().collect(Collectors.toMap(Map.Entry::getKey, Map.Entry::getValue));
}
@Override
public RequestKey getRequestKey() {
return requestKey;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy