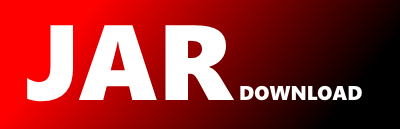
com.adobe.pdfservices.operation.pdfjobs.jobs.DeletePagesJob Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdfservices-sdk Show documentation
Show all versions of pdfservices-sdk Show documentation
Adobe PDF Services SDK allows you to access RESTful APIs to create, convert, and manipulate PDFs within your applications.
Older versions can be found under groupId: com.adobe.documentservices, artifactId: pdftools-sdk
/*
* Copyright 2024 Adobe
* All Rights Reserved.
*
* NOTICE: Adobe permits you to use, modify, and distribute this file in
* accordance with the terms of the Adobe license agreement accompanying
* it. If you have received this file from a source other than Adobe,
* then your use, modification, or distribution of it requires the prior
* written permission of Adobe.
*/
package com.adobe.pdfservices.operation.pdfjobs.jobs;
import com.adobe.pdfservices.operation.PDFServicesJob;
import com.adobe.pdfservices.operation.config.notifier.NotifierConfig;
import com.adobe.pdfservices.operation.exception.ServiceApiException;
import com.adobe.pdfservices.operation.internal.ExecutionContext;
import com.adobe.pdfservices.operation.internal.PDFServicesHelper;
import com.adobe.pdfservices.operation.internal.constants.CustomErrorMessages;
import com.adobe.pdfservices.operation.internal.constants.OperationHeaderInfoEndpointMap;
import com.adobe.pdfservices.operation.internal.dto.request.PlatformApiRequest;
import com.adobe.pdfservices.operation.internal.dto.request.pagemanipulation.DeletePageAction;
import com.adobe.pdfservices.operation.internal.dto.request.pagemanipulation.PageActionCommand;
import com.adobe.pdfservices.operation.internal.dto.request.pagemanipulation.PageManipulationExternalAssetRequest;
import com.adobe.pdfservices.operation.internal.dto.request.pagemanipulation.PageManipulationInternalAssetRequest;
import com.adobe.pdfservices.operation.internal.http.DefaultRequestHeaders;
import com.adobe.pdfservices.operation.internal.http.HttpResponse;
import com.adobe.pdfservices.operation.internal.util.ObjectUtil;
import com.adobe.pdfservices.operation.io.Asset;
import com.adobe.pdfservices.operation.io.CloudAsset;
import com.adobe.pdfservices.operation.io.ExternalAsset;
import com.adobe.pdfservices.operation.pdfjobs.params.deletepages.DeletePagesParams;
import java.util.ArrayList;
import java.util.List;
import java.util.UUID;
/**
* A job to delete pages in a PDF file.
*
*
* Sample Usage:
*
{@code
* InputStream inputStream = new FileInputStream(new File("SOURCE_PATH"));
*
* Credentials credentials = new ServicePrincipalCredentials(
* System.getenv("PDF_SERVICES_CLIENT_ID"),
* System.getenv("PDF_SERVICES_CLIENT_SECRET"));
*
* PDFServices pdfServices = new PDFServices(credentials);
*
* Asset asset = pdfServices.upload(inputStream, PDFServicesMediaType.PDF.getMediaType());
*
* PageRanges pageRangeForDeletion = new PageRanges();
* pageRangeForDeletion.addSinglePage(1);
* DeletePagesParams deletePagesParams = new DeletePagesParams(pageRangeForDeletion);
* DeletePagesJob deletePagesJob = new DeletePagesJob(asset, deletePagesParams);
*
* String location = pdfServices.submit(deletePagesJob);
* PDFServicesResponse pdfServicesResponse = pdfServices.getJobResult(location, DeletePagesResult.class);
*
* Asset deletePagesResult = pdfServicesResponse.getResult().getAsset();
* StreamAsset streamAsset = pdfServices.getContent(deletePagesResult);
* }
*/
public class DeletePagesJob extends PDFServicesJob {
private Asset inputAsset;
private Asset outputAsset;
private DeletePagesParams deletePagesParams;
/**
* Constructs a new {@code DeletePagesJob} instance.
*
* @param asset {@link Asset} object containing the input file; can not be null.
* @param deletePagesParams {@link DeletePagesParams} object containing the page ranges to be deleted; can not be
* null.
*/
public DeletePagesJob(Asset asset, DeletePagesParams deletePagesParams) {
ObjectUtil.requireNonNull(asset, String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL, "Input Asset"));
ObjectUtil.requireNonNull(deletePagesParams, String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL,
"Delete Pages parameters"));
this.inputAsset = asset;
this.deletePagesParams = deletePagesParams;
}
@Override
protected String process(ExecutionContext executionContext) throws ServiceApiException {
return this.process(executionContext, null);
}
@Override
protected String process(ExecutionContext executionContext, List notifyConfigList) throws ServiceApiException {
this.validate(executionContext);
PlatformApiRequest pageManipulationRequest = generatePlatformApiRequest(notifyConfigList);
String xRequestId = UUID.randomUUID().toString();
HttpResponse response = PDFServicesHelper.submitJob(executionContext, pageManipulationRequest,
xRequestId,
OperationHeaderInfoEndpointMap.DELETE_PAGES);
return response.getHeaders().get(DefaultRequestHeaders.LOCATION_HEADER_NAME);
}
/**
* Sets the output asset for the job.
* {@code @note} External assets can be set as output only when input is external asset as well
*
* @param asset {@link Asset} object representing the output asset; can not be null.
* @return {@code DeletePagesJob} instance
*/
public DeletePagesJob setOutput(Asset asset) {
ObjectUtil.requireNonNull(asset, String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL, "Output asset"));
if (this.inputAsset instanceof CloudAsset) {
throw new IllegalArgumentException(CustomErrorMessages.SET_OUTPUT_VALIDATE);
}
this.outputAsset = asset;
return this;
}
private List getPageActionCommands() {
List pageActionCommands = new ArrayList<>();
DeletePageAction deletePageAction = new DeletePageAction(this.deletePagesParams.getPageRanges().getRanges());
pageActionCommands.add(PageActionCommand.createFrom(deletePageAction));
return pageActionCommands;
}
private PlatformApiRequest generatePlatformApiRequest(List notifyConfigList) {
PlatformApiRequest pageManipulationRequest;
if (this.inputAsset instanceof CloudAsset) {
pageManipulationRequest =
new PageManipulationInternalAssetRequest(( (CloudAsset) this.inputAsset ).getAssetId(),
getPageActionCommands(), notifyConfigList);
} else {
pageManipulationRequest = new PageManipulationExternalAssetRequest((ExternalAsset) this.inputAsset,
getPageActionCommands(),
notifyConfigList).setOutput((ExternalAsset) outputAsset);
}
return pageManipulationRequest;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy