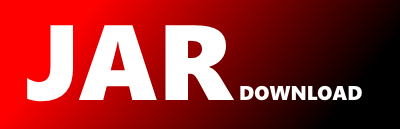
com.adobe.pdfservices.operation.pdfjobs.jobs.OCRJob Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdfservices-sdk Show documentation
Show all versions of pdfservices-sdk Show documentation
Adobe PDF Services SDK allows you to access RESTful APIs to create, convert, and manipulate PDFs within your applications.
Older versions can be found under groupId: com.adobe.documentservices, artifactId: pdftools-sdk
/*
* Copyright 2024 Adobe
* All Rights Reserved.
*
* NOTICE: Adobe permits you to use, modify, and distribute this file in
* accordance with the terms of the Adobe license agreement accompanying
* it. If you have received this file from a source other than Adobe,
* then your use, modification, or distribution of it requires the prior
* written permission of Adobe.
*/
package com.adobe.pdfservices.operation.pdfjobs.jobs;
import com.adobe.pdfservices.operation.PDFServicesJob;
import com.adobe.pdfservices.operation.config.notifier.NotifierConfig;
import com.adobe.pdfservices.operation.exception.ServiceApiException;
import com.adobe.pdfservices.operation.internal.ExecutionContext;
import com.adobe.pdfservices.operation.internal.PDFServicesHelper;
import com.adobe.pdfservices.operation.internal.constants.CustomErrorMessages;
import com.adobe.pdfservices.operation.internal.constants.OperationHeaderInfoEndpointMap;
import com.adobe.pdfservices.operation.internal.dto.request.PlatformApiRequest;
import com.adobe.pdfservices.operation.internal.dto.request.ocr.OCRExternalAssetRequest;
import com.adobe.pdfservices.operation.internal.dto.request.ocr.OCRInternalAssetRequest;
import com.adobe.pdfservices.operation.internal.http.DefaultRequestHeaders;
import com.adobe.pdfservices.operation.internal.http.HttpResponse;
import com.adobe.pdfservices.operation.internal.util.ObjectUtil;
import com.adobe.pdfservices.operation.io.Asset;
import com.adobe.pdfservices.operation.io.CloudAsset;
import com.adobe.pdfservices.operation.io.ExternalAsset;
import com.adobe.pdfservices.operation.pdfjobs.params.ocr.OCRParams;
import com.adobe.pdfservices.operation.pdfjobs.params.ocr.OCRSupportedLocale;
import com.adobe.pdfservices.operation.pdfjobs.params.ocr.OCRSupportedType;
import java.util.List;
import java.util.UUID;
/**
* A job that convert a PDF file into a searchable PDF file. Allows specifying locale({@link OCRSupportedLocale}) and
* OCR Type
* ({@link OCRSupportedType}) for performing OCR (Optical Character Recognition)
*
* Sample Usage:
*
{@code
* InputStream inputStream = new FileInputStream(new File("SOURCE_PATH"));
*
* Credentials credentials = new ServicePrincipalCredentials(
* System.getenv("PDF_SERVICES_CLIENT_ID"),
* System.getenv("PDF_SERVICES_CLIENT_SECRET"));
*
* PDFServices pdfServices = new PDFServices(credentials);
*
* Asset asset = pdfServices.upload(inputStream, PDFServicesMediaType.PDF.getMediaType());
*
* OCRJob ocrJob = new OCRJob(asset);
*
* String location = pdfServices.submit(ocrJOB);
* PDFServicesResponse pdfServicesResponse = pdfServices.getJobResult(location, OCRResult.class);
*
* Asset resultAsset = pdfServicesResponse.getResult().getAsset();
* StreamAsset StreamAsset = pdfServices.getContent(linearizeAssetResult);
* }
*/
public class OCRJob extends PDFServicesJob {
private Asset inputAsset;
private Asset outputAsset;
private OCRParams ocrParams;
/**
* Constructs a new {@code OCRJob} instance.
*
* @param asset {@link Asset} object containing the input file; can not be null.
*/
public OCRJob(Asset asset) {
ObjectUtil.requireNonNull(asset, String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL, "Input Asset"));
this.inputAsset = asset;
}
@Override
protected String process(ExecutionContext executionContext) throws ServiceApiException {
return this.process(executionContext, null);
}
@Override
protected String process(ExecutionContext executionContext, List notifyConfigList) throws ServiceApiException {
this.validate(executionContext);
PlatformApiRequest ocrRequest = generatePlatformApiRequest(notifyConfigList);
String xRequestId = UUID.randomUUID().toString();
HttpResponse response = PDFServicesHelper.submitJob(executionContext, ocrRequest, xRequestId,
OperationHeaderInfoEndpointMap.OCR);
return response.getHeaders().get(DefaultRequestHeaders.LOCATION_HEADER_NAME);
}
/**
* Set params for the job.
*
* @param ocrParams {@link OCRParams} object containing the OCR parameters; can not be null.
* @return this {@link OCRJob} instance
*/
public OCRJob setParams(OCRParams ocrParams) {
ObjectUtil.requireNonNull(ocrParams, String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL, "OCR " +
"parameters"));
this.ocrParams = ocrParams;
return this;
}
/**
* Sets the output asset for the job.
* {@code @note} External assets can be set as output only when input is external asset as well
*
* @param asset {@link Asset} object representing the output asset; can not be null.
* @return {@code OCRJob} instance
*/
public OCRJob setOutput(Asset asset) {
ObjectUtil.requireNonNull(asset, String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL, "Output asset"));
if (this.inputAsset instanceof CloudAsset) {
throw new IllegalArgumentException(CustomErrorMessages.SET_OUTPUT_VALIDATE);
}
this.outputAsset = asset;
return this;
}
private PlatformApiRequest generatePlatformApiRequest(List notifyConfigList) {
PlatformApiRequest ocrRequest;
if (this.inputAsset instanceof CloudAsset) {
ocrRequest = new OCRInternalAssetRequest(( (CloudAsset) this.inputAsset ).getAssetId(), this.ocrParams,
notifyConfigList);
} else {
ocrRequest = new OCRExternalAssetRequest((ExternalAsset) this.inputAsset, this.ocrParams,
notifyConfigList).setOutput((ExternalAsset) outputAsset);
}
return ocrRequest;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy