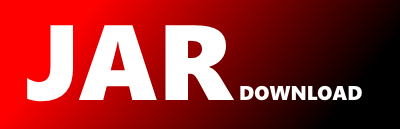
com.adobe.pdfservices.operation.pdfjobs.jobs.RemoveProtectionJob Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdfservices-sdk Show documentation
Show all versions of pdfservices-sdk Show documentation
Adobe PDF Services SDK allows you to access RESTful APIs to create, convert, and manipulate PDFs within your applications.
Older versions can be found under groupId: com.adobe.documentservices, artifactId: pdftools-sdk
/*
* Copyright 2024 Adobe
* All Rights Reserved.
*
* NOTICE: Adobe permits you to use, modify, and distribute this file in
* accordance with the terms of the Adobe license agreement accompanying
* it. If you have received this file from a source other than Adobe,
* then your use, modification, or distribution of it requires the prior
* written permission of Adobe.
*/
package com.adobe.pdfservices.operation.pdfjobs.jobs;
import com.adobe.pdfservices.operation.PDFServicesJob;
import com.adobe.pdfservices.operation.config.notifier.NotifierConfig;
import com.adobe.pdfservices.operation.exception.ServiceApiException;
import com.adobe.pdfservices.operation.internal.ExecutionContext;
import com.adobe.pdfservices.operation.internal.PDFServicesHelper;
import com.adobe.pdfservices.operation.internal.constants.CustomErrorMessages;
import com.adobe.pdfservices.operation.internal.constants.OperationHeaderInfoEndpointMap;
import com.adobe.pdfservices.operation.internal.dto.request.PlatformApiRequest;
import com.adobe.pdfservices.operation.internal.dto.request.removeprotection.RemoveProtectionExternalAssetRequest;
import com.adobe.pdfservices.operation.internal.dto.request.removeprotection.RemoveProtectionInternalAssetRequest;
import com.adobe.pdfservices.operation.internal.http.DefaultRequestHeaders;
import com.adobe.pdfservices.operation.internal.http.HttpResponse;
import com.adobe.pdfservices.operation.internal.util.ObjectUtil;
import com.adobe.pdfservices.operation.io.Asset;
import com.adobe.pdfservices.operation.io.CloudAsset;
import com.adobe.pdfservices.operation.io.ExternalAsset;
import com.adobe.pdfservices.operation.pdfjobs.params.removeprotection.RemoveProtectionParams;
import java.util.List;
import java.util.UUID;
/**
* A job that allows to remove password security from a PDF document.
*
*
* Sample Usage:
*
{@code
* InputStream inputStream = new FileInputStream(new File("SOURCE_PATH"));
*
* Credentials credentials = new ServicePrincipalCredentials(
* System.getenv("PDF_SERVICES_CLIENT_ID"),
* System.getenv("PDF_SERVICES_CLIENT_SECRET"));
*
* PDFServices pdfServices = new PDFServices(credentials);
*
* Asset asset = pdfServices.upload(inputStream, PDFServicesMediaType.PDF.getMediaType());
*
* RemoveProtectionParams removeProtectionParams = new RemoveProtectionParams("mypassword");
* RemoveProtectionJob removeProtectionJob = new RemoveProtectionJob(asset, removeProtectionParams);
*
* String location = pdfServices.submit(removeProtectionJob);
* PDFServicesResponse pdfServicesResponse = pdfServices.getJobResult(location, RemoveProtectionResult.class);
*
* Asset resultAsset = pdfServicesResponse.getResult().getAsset();
* StreamAsset streamAsset = pdfServices.getContent(resultAsset);
* }
*/
public class RemoveProtectionJob extends PDFServicesJob {
private Asset inputAsset;
private Asset outputAsset;
private RemoveProtectionParams removeProtectionParams;
/**
* Constructs a new {@code RemoveProtectionJob} instance.
*
* @param asset {@link Asset} object containing the input file; can not be null.
* @param removeProtectionParams {@link RemoveProtectionParams} object containing the password for removing
* protection; can not be null.
*/
public RemoveProtectionJob(Asset asset, RemoveProtectionParams removeProtectionParams) {
ObjectUtil.requireNonNull(asset, String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL, "Input Asset"));
ObjectUtil.requireNonNull(removeProtectionParams, String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL,
"Remove Protection parameters"));
this.inputAsset = asset;
this.removeProtectionParams = removeProtectionParams;
}
@Override
protected String process(ExecutionContext executionContext) throws ServiceApiException {
return this.process(executionContext, null);
}
@Override
protected String process(ExecutionContext executionContext, List notifyConfigList) throws ServiceApiException {
this.validate(executionContext);
PlatformApiRequest removeProtectionRequest = generatePlatformApiRequest(notifyConfigList);
String xRequestId = UUID.randomUUID().toString();
HttpResponse response = PDFServicesHelper.submitJob(executionContext, removeProtectionRequest,
xRequestId,
OperationHeaderInfoEndpointMap.REMOVE_PROTECTION);
return response.getHeaders().get(DefaultRequestHeaders.LOCATION_HEADER_NAME);
}
/**
* Sets the output asset for the job.
* {@code @note} External assets can be set as output only when input is external asset as well
*
* @param asset {@link Asset} object representing the output asset; can not be null.
* @return {@code RemoveProtectionJob} instance
*/
public RemoveProtectionJob setOutput(Asset asset) {
ObjectUtil.requireNonNull(asset, String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL, "Output asset"));
if (this.inputAsset instanceof CloudAsset) {
throw new IllegalArgumentException(CustomErrorMessages.SET_OUTPUT_VALIDATE);
}
this.outputAsset = asset;
return this;
}
private PlatformApiRequest generatePlatformApiRequest(List notifyConfigList) {
PlatformApiRequest removeProtectionRequest;
if (this.inputAsset instanceof CloudAsset) {
removeProtectionRequest =
new RemoveProtectionInternalAssetRequest(( (CloudAsset) this.inputAsset ).getAssetId(),
this.removeProtectionParams, notifyConfigList);
} else {
removeProtectionRequest = new RemoveProtectionExternalAssetRequest((ExternalAsset) this.inputAsset,
this.removeProtectionParams,
notifyConfigList).setOutput((ExternalAsset) outputAsset);
}
return removeProtectionRequest;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy