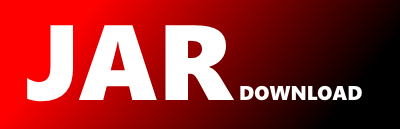
com.adobe.pdfservices.operation.pdfjobs.params.PageRanges Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdfservices-sdk Show documentation
Show all versions of pdfservices-sdk Show documentation
Adobe PDF Services SDK allows you to access RESTful APIs to create, convert, and manipulate PDFs within your applications.
Older versions can be found under groupId: com.adobe.documentservices, artifactId: pdftools-sdk
/*
* Copyright 2024 Adobe
* All Rights Reserved.
*
* NOTICE: Adobe permits you to use, modify, and distribute this file in
* accordance with the terms of the Adobe license agreement accompanying
* it. If you have received this file from a source other than Adobe,
* then your use, modification, or distribution of it requires the prior
* written permission of Adobe.
*/
package com.adobe.pdfservices.operation.pdfjobs.params;
import com.adobe.pdfservices.operation.internal.params.PageRange;
import java.util.ArrayList;
import java.util.List;
import java.util.stream.Collectors;
/**
* Page ranges of a file, inclusive of start and end page index, where page index starts from 1.
*/
public class PageRanges {
private static final int RANGES_MAX_LIMIT = 100;
private List ranges;
/**
* Constructs a new PageRanges instance with no pages added.
*/
public PageRanges() {
ranges = new ArrayList<>();
}
/**
* Adds a single page. Page index starts from 1.
*
* @param page single page index
*/
public void addSinglePage(int page) {
ranges.add(new PageRange(page, page));
}
/**
* Adds a page range. Page indexes start from 1.
*
* @param start start page index, inclusive
* @param end end page index, inclusive
*/
public void addRange(int start, int end) {
ranges.add(new PageRange(start, end));
}
/**
* Adds a page range from {@code start} page index to the last page. Page index starts from 1.
*
* @param start start page index
*/
public void addAllFrom(int start) {
ranges.add(new PageRange(start, null));
}
/**
* Adds a page range representing all pages.
*/
public void addAll() {
ranges.add(new PageRange(1, null));
}
/**
* Used internally by this SDK, not intended to be called by clients.
*
* @return string representation
*/
@Override
public String toString() {
return this.ranges.stream().map(PageRange::toString).collect(Collectors.joining(","));
}
/**
* Used internally by this SDK, not intended to be called by clients.
*/
public void validate() {
if (this.ranges.size() > RANGES_MAX_LIMIT) {
throw new IllegalArgumentException("Maximum allowed limit exceeded for page ranges.");
}
this.ranges.forEach(PageRange::validate);
}
/**
* Used internally by this SDK, not intended to be called by clients.
*/
public boolean isEmpty() {
return ranges.isEmpty();
}
/**
* Used internally by this SDK, not intended to be called by clients.
*/
public List getRanges() {
return ranges;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy