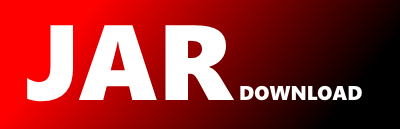
com.adobe.pdfservices.operation.pdfjobs.params.electronicseal.PDFElectronicSealParams Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdfservices-sdk Show documentation
Show all versions of pdfservices-sdk Show documentation
Adobe PDF Services SDK allows you to access RESTful APIs to create, convert, and manipulate PDFs within your applications.
Older versions can be found under groupId: com.adobe.documentservices, artifactId: pdftools-sdk
/*
* Copyright 2024 Adobe
* All Rights Reserved.
*
* NOTICE: Adobe permits you to use, modify, and distribute this file in
* accordance with the terms of the Adobe license agreement accompanying
* it. If you have received this file from a source other than Adobe,
* then your use, modification, or distribution of it requires the prior
* written permission of Adobe.
*/
package com.adobe.pdfservices.operation.pdfjobs.params.electronicseal;
import com.adobe.pdfservices.operation.internal.util.ValidationUtil;
import com.adobe.pdfservices.operation.pdfjobs.jobs.PDFElectronicSealJob;
import com.adobe.pdfservices.operation.pdfjobs.params.PDFServicesJobParams;
/**
* Parameters for electronically seal PDFs using {@link PDFElectronicSealJob}.
*/
public class PDFElectronicSealParams implements PDFServicesJobParams {
private SignatureFormat signatureFormat;
private DocumentLevelPermission documentLevelPermission;
private CertificateCredentials certificateCredentials;
private TSAOptions tsaOptions;
private FieldOptions fieldOptions;
private AppearanceOptions appearanceOptions;
private PDFElectronicSealParams(Builder builder) {
this.signatureFormat = builder.signatureFormat;
this.certificateCredentials = builder.certificateCredentials;
this.fieldOptions = builder.fieldOptions;
this.appearanceOptions = builder.appearanceOptions;
this.documentLevelPermission = builder.documentLevelPermission;
this.tsaOptions = builder.tsaOptions;
}
/**
* Returns the intended {@link SignatureFormat} to be used for applying electronic seal.
*
* @return the signature format
*/
public SignatureFormat getSignatureFormat() {
return signatureFormat;
}
/**
* Returns the {@link CertificateCredentials} instance containing information regarding Certificate Credentials.
*
* @return the {@link CertificateCredentials} instance
*/
public CertificateCredentials getCertificateCredentials() {
return certificateCredentials;
}
/**
* Returns the {@link FieldOptions} instance containing information regarding seal field position in PDF Document.
*
* @return the {@link FieldOptions} instance
*/
public FieldOptions getFieldOptions() {
return fieldOptions;
}
/**
* Returns the document level permission for changes allowed after sealing.
*
* @return the {@link DocumentLevelPermission} for changes allowed after sealing
*/
public DocumentLevelPermission getDocumentLevelPermission() {
return documentLevelPermission;
}
/**
* Returns the {@link TSAOptions} instance containing information regarding TSA server.
*
* @return a {@link TSAOptions} instance
*/
public TSAOptions getTSAOptions() {
return tsaOptions;
}
/**
* Returns the {@link AppearanceOptions} instance containing information regarding elements to be displayed in
* Seal Field.
*
* @return the {@link AppearanceOptions} instance
*/
public AppearanceOptions getAppearanceOptions() {
return appearanceOptions;
}
/**
* Creates a new {@link Builder}.
*
* @return a {@link Builder} instance
*/
public static Builder pdfElectronicSealParamsBuilder(CertificateCredentials certificateCredentials, FieldOptions fieldOptions) {
return new Builder(certificateCredentials, fieldOptions);
}
/**
* Builds a {@link PDFElectronicSealParams} instance.
*/
public static class Builder {
private SignatureFormat signatureFormat;
private DocumentLevelPermission documentLevelPermission;
private CertificateCredentials certificateCredentials;
private TSAOptions tsaOptions;
private FieldOptions fieldOptions;
private AppearanceOptions appearanceOptions;
/**
* Constructs a {@code Builder} instance.
*
* @param certificateCredentials {@link CertificateCredentials} to be used for applying electronic seal
* @param fieldOptions {@link FieldOptions} to be used for applying electronic seal
*/
public Builder(CertificateCredentials certificateCredentials, FieldOptions fieldOptions) {
this.signatureFormat = SignatureFormat.PKCS7;
this.certificateCredentials = certificateCredentials;
this.fieldOptions = fieldOptions;
this.documentLevelPermission = DocumentLevelPermission.FORM_FILLING;
}
/**
* Sets the {@link AppearanceOptions} for the seal.
*
* @param appearanceOptions {@link AppearanceOptions} for the seal;
* @return this Builder instance to add any additional parameters
*/
public Builder withAppearanceOptions(AppearanceOptions appearanceOptions) {
this.appearanceOptions = appearanceOptions;
return this;
}
/**
* Sets the time stamp authority options
*
* @param tsaOptions the time stamp authority options to be set for applying electronic seal
* @return this Builder instance to add any additional parameters
*/
public Builder withTSAOptions(TSAOptions tsaOptions) {
this.tsaOptions = tsaOptions;
return this;
}
/**
* Sets the document level permission for changes allowed after sealing.
*
* @param documentLevelPermission {@link DocumentLevelPermission} document level permission for changes
* allowed after sealing
* @return this Builder instance to add any additional parameters
*/
public Builder withDocumentLevelPermission(DocumentLevelPermission documentLevelPermission) {
this.documentLevelPermission = documentLevelPermission;
return this;
}
/**
* Sets the {@link SignatureFormat} for the seal.
*
* @param signatureFormat {@link SignatureFormat} to be used for applying electronic seal
* @return this Builder instance to add any additional parameters
*/
public Builder withSignatureFormat(SignatureFormat signatureFormat) {
this.signatureFormat = signatureFormat;
return this;
}
/**
* Returns a new {@link PDFElectronicSealParams} instance built from the current state of this builder.
*
* @return a new {@code PDFElectronicSealParams} instance
*/
public PDFElectronicSealParams build() {
PDFElectronicSealParams pdfElectronicSealParams = new PDFElectronicSealParams(this);
ValidationUtil.validatePDFElectronicSealParams(pdfElectronicSealParams);
return pdfElectronicSealParams;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy