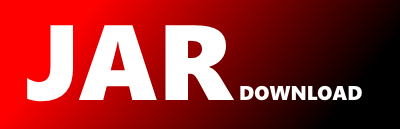
com.adobe.pdfservices.operation.pdfjobs.result.pdfproperties.Document Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdfservices-sdk Show documentation
Show all versions of pdfservices-sdk Show documentation
Adobe PDF Services SDK allows you to access RESTful APIs to create, convert, and manipulate PDFs within your applications.
Older versions can be found under groupId: com.adobe.documentservices, artifactId: pdftools-sdk
/*
* Copyright 2024 Adobe
* All Rights Reserved.
*
* NOTICE: Adobe permits you to use, modify, and distribute this file in
* accordance with the terms of the Adobe license agreement accompanying
* it. If you have received this file from a source other than Adobe,
* then your use, modification, or distribution of it requires the prior
* written permission of Adobe.
*/
package com.adobe.pdfservices.operation.pdfjobs.result.pdfproperties;
import java.util.List;
/**
* This class provides information about the document level properties of the specified PDF file, such as tags,
* fonts, PDF Version etc.
*/
public class Document {
private Boolean isLinearized;
private String pdfeComplianceLevel;
private Boolean isTagged;
private Boolean isPortfolio;
private Boolean isCertified;
private Boolean isEncrypted;
private InfoDict infoDict;
private Boolean isFTPDF;
private String pdfVersion;
private Boolean hasAcroform;
private String fileSize;
private Boolean isSigned;
private Integer incrementalSaveCount;
private Boolean hasEmbeddedFiles;
private Boolean isXFA;
private List fonts;
private String pdfaComplianceLevel;
private String pdfvtComplianceLevel;
private String pdfxComplianceLevel;
private String pdfuaComplianceLevel;
private String xmp;
private Integer pageCount;
/**
* Returns a boolean specifying whether the specified PDF file is linearized.
*
* @return {@code true} if the specified PDF file is linearized. False otherwise.
*/
public Boolean isLinearized() {
return isLinearized;
}
/**
* Returns a boolean specifying whether the specified PDF file is tagged.
*
* @return {@code true} if the specified PDF file is tagged. False otherwise.
*/
public Boolean isTagged() {
return isTagged;
}
/**
* Returns a boolean specifying whether the specified PDF file is a portfolio PDF.
*
* @return {@code true} if the specified PDF file is a portfolio PDF. False otherwise.
*/
public Boolean isPortfolio() {
return isPortfolio;
}
/**
* Returns a boolean specifying whether the specified PDF file has been digitally signed with a certifying
* signature.
*
* @return {@code true} if the specified PDF file has been digitally signed with a certifying signature. False
* otherwise.
*/
public Boolean isCertified() {
return isCertified;
}
/**
* Returns a boolean specifying whether the specified PDF file is encrypted.
*
* @return {@code true} if the specified PDF file is encrypted. False otherwise.
*/
public Boolean isEncrypted() {
return isEncrypted;
}
/**
* Returns a boolean specifying whether the specified PDF file is a Fully Tagged PDF.
*
* @return {@code true} if the specified PDF file is a Fully Tagged PDF. False otherwise.
*/
public Boolean isFullyTaggedPDF() {
return isFTPDF;
}
/**
* Returns a boolean specifying whether the specified PDF file contains any form fields.
*
* @return {@code true} if the specified PDF file contains any form fields. False otherwise.
*/
public Boolean isAcroform() {
return hasAcroform;
}
/**
* Returns a boolean specifying whether the specified PDF file is digitally signed.
*
* @return {@code true} if the specified PDF file is digitally signed. False otherwise.
*/
public Boolean isSigned() {
return isSigned;
}
/**
* Returns a boolean specifying whether the specified PDF file is based on the XFA (Extensible Forms
* Architecture) format.
*
* @return {@code true} if the specified PDF file is based on the XFA format. False otherwise
*/
public Boolean isXFA() {
return isXFA;
}
/**
* Returns a boolean specifying whether the specified PDF file contains any embedded files.
*
* @return {@code true} if the specified PDF file contains any embedded files. False otherwise.
*/
public Boolean containsEmbeddedFiles() {
return hasEmbeddedFiles;
}
/**
* Returns a list of {@link Font} instances present in the specified PDF file.
*
* @return A List of {@link Font} instances
*/
public List getFonts() {
return fonts;
}
/**
* Returns a string specifying the PDF/A compliance level for the specified PDF file - e.g "PDF/A-4" etc.
*
* @return A String denoting the PDF/A compliance level for the specified PDF file.
*/
public String getPDFAComplianceLevel() {
return pdfaComplianceLevel;
}
/**
* Returns a string specifying the PDF/VT compliance level for the specified PDF file - e.g. "PDF/VT-3" etc.
*
* @return A String denoting the PDF/VT compliance level for the specified PDF file.
*/
public String getPDFVTComplianceLevel() {
return pdfvtComplianceLevel;
}
/**
* Returns a string specifying the PDF/X compliance level for the specified PDF file - e.g. "PDF/X-4" etc.
*
* @return A String denoting the PDF/X compliance level for the specified PDF file.
*/
public String getPDFXComplianceLevel() {
return pdfxComplianceLevel;
}
/**
* Returns a string specifying the PDF/UA compliance level for the specified PDF file - e.g. "PDF/UA-1" etc.
*
* @return A String denoting the PDF/UA compliance level for the specified PDF file.
*/
public String getPDFUAComplianceLevel() {
return pdfuaComplianceLevel;
}
/**
* Returns a string specifying the full metadata of the specified PDF file as provided by the author and producer
* of the document.
*
* @return A String denoting the full metadata of the specified PDF file as provided by the author and producer
* of the document.
*/
public String getXMPMetadata() {
return xmp;
}
/**
* Returns a number specifying the number of pages in the specified PDF file.
*
* @return An Integer denoting the number of pages in the specified PDF file.
*/
public Integer getPageCount() {
return pageCount;
}
/**
* Returns a string specifying the PDF/E compliance level for the specified PDF file - e.g. "PDF/E-1" etc.
*
* @return A String denoting the PDF/E compliance level for the specified PDF file.
*/
public String getPDFEComplianceLevel() {
return pdfeComplianceLevel;
}
/**
* Returns an {@link InfoDict} instance that specifies information related to the specified PDF file such as
* creation date, author etc.
*
* @return an {@link InfoDict} instance.
*/
public InfoDict getInfoDict() {
return infoDict;
}
/**
* Returns a string specifying the version of the specified PDF file - e.g. "1.7", "2.0" etc.
*
* @return A String denoting the version of the specified PDF file.
*/
public String getPDFVersion() {
return pdfVersion;
}
/**
* Returns the size of the specified PDF file.
*
* @return A String denoting the size of the specified PDF file.
*/
public String getFileSize() {
return fileSize;
}
/**
* Returns the count of the number of times the specified PDF file is edited and saved.
*
* @return An Integer denoting the count of the number of times the specified PDF file is edited and saved.
*/
public Integer getIncrementalSaveCount() {
return incrementalSaveCount;
}
void setLinearized(Boolean linearized) {
isLinearized = linearized;
}
void setPdfeComplianceLevel(String pdfeComplianceLevel) {
this.pdfeComplianceLevel = pdfeComplianceLevel;
}
void setTagged(Boolean tagged) {
isTagged = tagged;
}
void setPortfolio(Boolean portfolio) {
isPortfolio = portfolio;
}
void setCertified(Boolean certified) {
isCertified = certified;
}
void setEncrypted(Boolean encrypted) {
isEncrypted = encrypted;
}
void setInfoDict(InfoDict infoDict) {
this.infoDict = infoDict;
}
void setFTPDF(Boolean FTPDF) {
isFTPDF = FTPDF;
}
void setPdfVersion(String pdfVersion) {
this.pdfVersion = pdfVersion;
}
void setHasAcroform(Boolean hasAcroform) {
this.hasAcroform = hasAcroform;
}
void setFileSize(String fileSize) {
this.fileSize = fileSize;
}
void setSigned(Boolean signed) {
isSigned = signed;
}
void setIncrementalSaveCount(Integer incrementalSaveCount) {
this.incrementalSaveCount = incrementalSaveCount;
}
void setHasEmbeddedFiles(Boolean hasEmbeddedFiles) {
this.hasEmbeddedFiles = hasEmbeddedFiles;
}
void setXFA(Boolean XFA) {
isXFA = XFA;
}
void setFonts(List fonts) {
this.fonts = fonts;
}
void setPdfaComplianceLevel(String pdfaComplianceLevel) {
this.pdfaComplianceLevel = pdfaComplianceLevel;
}
void setPdfvtComplianceLevel(String pdfvtComplianceLevel) {
this.pdfvtComplianceLevel = pdfvtComplianceLevel;
}
void setPdfxComplianceLevel(String pdfxComplianceLevel) {
this.pdfxComplianceLevel = pdfxComplianceLevel;
}
void setPdfuaComplianceLevel(String pdfuaComplianceLevel) {
this.pdfuaComplianceLevel = pdfuaComplianceLevel;
}
void setXmp(String xmp) {
this.xmp = xmp;
}
void setPageCount(Integer pageCount) {
this.pageCount = pageCount;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy