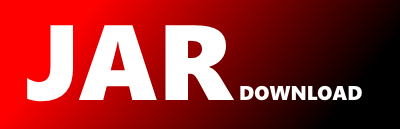
com.adobe.pdfservices.operation.pdfjobs.result.pdfproperties.PermissionSettings Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdfservices-sdk Show documentation
Show all versions of pdfservices-sdk Show documentation
Adobe PDF Services SDK allows you to access RESTful APIs to create, convert, and manipulate PDFs within your applications.
Older versions can be found under groupId: com.adobe.documentservices, artifactId: pdftools-sdk
/*
* Copyright 2024 Adobe
* All Rights Reserved.
*
* NOTICE: Adobe permits you to use, modify, and distribute this file in
* accordance with the terms of the Adobe license agreement accompanying
* it. If you have received this file from a source other than Adobe,
* then your use, modification, or distribution of it requires the prior
* written permission of Adobe.
*/
package com.adobe.pdfservices.operation.pdfjobs.result.pdfproperties;
/**
* This class provides information about the permission settings of the specified PDF file, such as restricting print
* permissions,
* edit permissions etc.
*/
public class PermissionSettings {
public Boolean assistiveTechnology;
public Boolean formFilling;
public Boolean copying;
public Boolean pageExtraction;
public Boolean documentAssembly;
public Boolean commenting;
public String printing;
public Boolean editing;
/**
* Returns a boolean specifying whether the specified PDF file will be supported by a screen reader.
*
* @return {@code true} if the specified PDF file will be supported by a screen reader. False otherwise.
*/
public Boolean hasAssistiveTechnology() {
return assistiveTechnology;
}
/**
* Returns a boolean specifying whether form filling is permitted in the specified PDF file.
*
* @return {@code true} if form filling is permitted in the specified PDF file. False otherwise.
*/
public Boolean isFormFillingAllowed() {
return formFilling;
}
/**
* Returns a boolean specifying whether copying the content of the specified PDF file is permitted.
*
* @return {@code true} if copying the content of the specified PDF file is permitted. False otherwise.
*/
public Boolean isCopyingAllowed() {
return copying;
}
/**
* Returns a boolean specifying whether extracting pages of the specified PDF file is permitted.
*
* @return {@code true} if extracting pages of the specified PDF file is permitted. False otherwise.
*/
public Boolean isPageExtractionAllowed() {
return pageExtraction;
}
/**
* Returns a boolean specifying whether adding or inserting pages in the specified PDF file is permitted.
*
* @return {@code true} if adding or inserting pages in the specified PDF file is permitted. False otherwise.
*/
public Boolean isDocumentAssemblyAllowed() {
return documentAssembly;
}
/**
* Returns a boolean specifying whether adding comments in the specified PDF file is permitted.
*
* @return {@code true} if adding comments in the specified PDF file is permitted. False otherwise.
*/
public Boolean isCommentingAllowed() {
return commenting;
}
/**
* Returns a boolean specifying whether editing the content in the specified PDF file is permitted.
*
* @return {@code true} if editing the content in the specified PDF file is permitted. False otherwise.
*/
public Boolean isEditingAllowed() {
return editing;
}
/**
* Returns a string specifying permission level for printing the file.
*
* @return A String denoting the permission level for printing the file.
*/
public String getPrintingPermission() {
return printing;
}
void setAssistiveTechnology(Boolean assistiveTechnology) {
this.assistiveTechnology = assistiveTechnology;
}
void setFormFilling(Boolean formFilling) {
this.formFilling = formFilling;
}
void setCopying(Boolean copying) {
this.copying = copying;
}
void setPageExtraction(Boolean pageExtraction) {
this.pageExtraction = pageExtraction;
}
void setDocumentAssembly(Boolean documentAssembly) {
this.documentAssembly = documentAssembly;
}
void setCommenting(Boolean commenting) {
this.commenting = commenting;
}
void setPrinting(String printing) {
this.printing = printing;
}
void setEditing(Boolean editing) {
this.editing = editing;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy