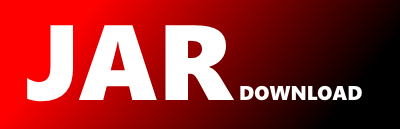
com.adobe.pdfservices.operation.internal.PlatformServiceRequestContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdfservices-sdk Show documentation
Show all versions of pdfservices-sdk Show documentation
Adobe PDF Services SDK allows you to access RESTful APIs to create, convert, and manipulate PDFs within your applications.
Older versions can be found under groupId: com.adobe.documentservices, artifactId: pdftools-sdk
/*
* Copyright 2019 Adobe
* All Rights Reserved.
*
* NOTICE: Adobe permits you to use, modify, and distribute this file in
* accordance with the terms of the Adobe license agreement accompanying
* it. If you have received this file from a source other than Adobe,
* then your use, modification, or distribution of it requires the prior
* written permission of Adobe.
*/
package com.adobe.pdfservices.operation.internal;
import com.adobe.pdfservices.operation.internal.constants.RequestKey;
import com.adobe.pdfservices.operation.internal.http.BaseHttpRequest;
import com.adobe.pdfservices.operation.internal.http.DefaultRequestHeaders;
import com.adobe.pdfservices.operation.internal.http.HttpMethod;
import com.adobe.pdfservices.operation.internal.http.HttpRequest;
import com.adobe.pdfservices.operation.internal.http.RequestType;
import org.apache.http.HttpHeaders;
import java.util.HashMap;
import java.util.Map;
public class PlatformServiceRequestContext {
private static final String ACCEPT_HEADER_VALUE = "application/json, text/plain, */*";
private Map baseRequestMap;
public PlatformServiceRequestContext(String platformServiceOpsCreateUri) {
baseRequestMap = new HashMap<>();
baseRequestMap.put(RequestKey.STATUS, new BaseHttpRequest(HttpMethod.GET).withRequestKey(RequestKey.STATUS));
baseRequestMap.put(RequestKey.PLATFORM,
new BaseHttpRequest(HttpMethod.POST).withTemplate(platformServiceOpsCreateUri)
.withRequestKey(RequestKey.PLATFORM));
baseRequestMap.put(RequestKey.PLATFORM_GET_ASSET,
new BaseHttpRequest(HttpMethod.GET).withTemplate(platformServiceOpsCreateUri)
.withRequestKey(RequestKey.PLATFORM_GET_ASSET));
baseRequestMap.put(RequestKey.PLATFORM_DELETE_ASSET,
new BaseHttpRequest(HttpMethod.DELETE).withTemplate(platformServiceOpsCreateUri)
.withRequestKey(RequestKey.PLATFORM_DELETE_ASSET));
baseRequestMap.put(RequestKey.UPLOAD, new BaseHttpRequest(HttpMethod.PUT).withRequestKey(RequestKey.UPLOAD));
baseRequestMap.put(RequestKey.DOWNLOAD,
new BaseHttpRequest(HttpMethod.GET).withRequestKey(RequestKey.DOWNLOAD));
}
public synchronized HttpRequest getBaseRequest(RequestKey requestKey) {
HttpRequest baseRequest = baseRequestMap.get(requestKey);
return copy(baseRequest);
}
private HttpRequest copy(HttpRequest httpRequest) {
RequestType requestType = httpRequest.getRequestType();
HttpRequest httpRequestCopy = null;
switch (requestType) {
case REGULAR:
if (RequestKey.PLATFORM.equals(httpRequest.getRequestKey()) || RequestKey.PLATFORM_GET_ASSET.equals(httpRequest.getRequestKey()) || RequestKey.PLATFORM_DELETE_ASSET.equals(httpRequest.getRequestKey())) {
httpRequestCopy =
new BaseHttpRequest(httpRequest.getHttpMethod()).withRequestKey(httpRequest.getRequestKey())
.withTemplate(httpRequest.getTemplateString()).withHeaders(getDefaultHeaders());
} else {
httpRequestCopy =
new BaseHttpRequest(httpRequest.getHttpMethod()).withRequestKey(httpRequest.getRequestKey())
.withHeaders(getDefaultHeaders());
}
break;
}
return httpRequestCopy;
}
private Map getDefaultHeaders() {
Map headers = new HashMap<>();
headers.put(HttpHeaders.ACCEPT, ACCEPT_HEADER_VALUE);
headers.put(DefaultRequestHeaders.DC_APP_INFO_HEADER_KEY, GlobalConfig.getAppInfo());
headers.put(DefaultRequestHeaders.PREFER_HEADER_NAME, DefaultRequestHeaders.PREFER_HEADER_VALUE);
return headers;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy