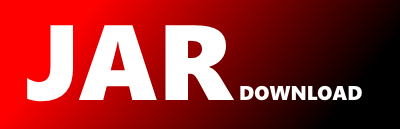
com.adobe.pdfservices.operation.internal.util.ValidationUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdfservices-sdk Show documentation
Show all versions of pdfservices-sdk Show documentation
Adobe PDF Services SDK allows you to access RESTful APIs to create, convert, and manipulate PDFs within your applications.
Older versions can be found under groupId: com.adobe.documentservices, artifactId: pdftools-sdk
/*
* Copyright 2019 Adobe
* All Rights Reserved.
*
* NOTICE: Adobe permits you to use, modify, and distribute this file in
* accordance with the terms of the Adobe license agreement accompanying
* it. If you have received this file from a source other than Adobe,
* then your use, modification, or distribution of it requires the prior
* written permission of Adobe.
*/
package com.adobe.pdfservices.operation.internal.util;
import com.adobe.pdfservices.operation.internal.ExecutionContext;
import com.adobe.pdfservices.operation.internal.constants.CustomErrorMessages;
import com.adobe.pdfservices.operation.internal.dto.request.pagemanipulation.PageAction;
import com.adobe.pdfservices.operation.internal.params.CombinePDFJobInput;
import com.adobe.pdfservices.operation.internal.params.PageRange;
import com.adobe.pdfservices.operation.io.Asset;
import com.adobe.pdfservices.operation.pdfjobs.params.PageRanges;
import com.adobe.pdfservices.operation.pdfjobs.params.documentmerge.DocumentMergeParams;
import com.adobe.pdfservices.operation.pdfjobs.params.electronicseal.CSCCredential;
import com.adobe.pdfservices.operation.pdfjobs.params.electronicseal.FieldLocation;
import com.adobe.pdfservices.operation.pdfjobs.params.electronicseal.FieldOptions;
import com.adobe.pdfservices.operation.pdfjobs.params.electronicseal.PDFElectronicSealParams;
import com.adobe.pdfservices.operation.pdfjobs.params.protectpdf.EncryptionAlgorithm;
import com.adobe.pdfservices.operation.pdfjobs.params.protectpdf.PasswordProtectParams;
import com.adobe.pdfservices.operation.pdfjobs.params.protectpdf.ProtectPDFParams;
import java.nio.charset.StandardCharsets;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.List;
import java.util.Map;
import static java.lang.String.format;
public class ValidationUtil {
private static final int PASSWORD_MAX_LENGTH = 128; // 128 bytes
private static final int PAGE_ACTIONS_MAX_LIMIT = 200;
private static final String USER_STRING = "User";
private static final String OWNER_STRING = "Owner";
private static final int REMOVE_PROTECTION_PASSWORD_MAX_LENGTH = 150; // 150 characters
private static final int SPLIT_PDF_OUTPUT_COUNT_LIMIT = 20;
public static void validateExecutionContext(ExecutionContext context) {
ObjectUtil.requireNonNull(context, "Context not initialized before invoking the operation");
if (context.getClientConfig() == null) {
throw new IllegalArgumentException("Client configuration not initialized before invoking the operation");
}
context.validate();
}
public static void validateAssetWithPageOptions(CombinePDFJobInput input) {
ObjectUtil.requireNonNull(input.getAsset(), "No input asset was set for the submitted job");
validatePageOptions(input);
}
public static void validatePDFElectronicSealParams(PDFElectronicSealParams pdfElectronicSealParams) {
if (pdfElectronicSealParams == null) {
throw new IllegalArgumentException(String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL, "PDF " +
"Electronic Seal Params"));
}
if (pdfElectronicSealParams.getCertificateCredentials() == null) {
throw new IllegalArgumentException(String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL,
"Certificate credentials"));
}
if (pdfElectronicSealParams.getFieldOptions() == null) {
throw new IllegalArgumentException(String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL, "Field " +
"options"));
}
CSCCredential cscCredential = (CSCCredential) pdfElectronicSealParams.getCertificateCredentials();
validateCSCCredential(cscCredential);
FieldOptions fieldOptions = pdfElectronicSealParams.getFieldOptions();
validateFieldOptions(fieldOptions);
}
private static void validateFieldOptions(FieldOptions fieldOptions) {
if (StringUtil.isBlank(fieldOptions.getFieldName())) {
throw new IllegalArgumentException(String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL_OR_EMPTY,
"Field name in field options"));
}
if (fieldOptions.getPageNumber() != null && fieldOptions.getPageNumber() <= 0) {
throw new IllegalArgumentException("Page number in field options is invalid.");
}
FieldLocation fieldLocation = fieldOptions.getFieldLocation();
if (fieldLocation != null) {
validateFieldLocation(fieldLocation);
}
}
private static void validateFieldLocation(FieldLocation fieldLocation) {
if (fieldLocation.getLeft() == null) {
throw new IllegalArgumentException(String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL, "Left " +
"coordinate in field location"));
}
if (fieldLocation.getTop() == null) {
throw new IllegalArgumentException(String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL, "Top " +
"coordinate in field location"));
}
if (fieldLocation.getRight() == null) {
throw new IllegalArgumentException(String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL, "Right " +
"coordinate in field location"));
}
if (fieldLocation.getBottom() == null) {
throw new IllegalArgumentException(String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL, "Bottom " +
"coordinate in field location"));
}
}
private static void validateCSCCredential(CSCCredential cscCredential) {
if (StringUtil.isBlank(cscCredential.getCredentialID())) {
throw new IllegalArgumentException(String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL_OR_EMPTY,
"Credential ID in CSC credentials"));
}
if (StringUtil.isBlank(cscCredential.getPin())) {
throw new IllegalArgumentException(String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL_OR_EMPTY,
"Pin in CSC credentials"));
}
if (cscCredential.getCscAuthContext() == null) {
throw new IllegalArgumentException(String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL, "Auth " +
"context in CSC credentials"));
}
if (StringUtil.isBlank(cscCredential.getCscAuthContext().getAccessToken())) {
throw new IllegalArgumentException(String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL_OR_EMPTY,
"Access token in auth context for CSC credentials"));
}
if (StringUtil.isBlank(cscCredential.getProviderName())) {
throw new IllegalArgumentException(String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL_OR_EMPTY,
"Provider name in CSC credentials"));
}
if (StringUtil.isBlank(cscCredential.getCscAuthContext().getTokenType())) {
throw new IllegalArgumentException(String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL_OR_EMPTY,
"Token type in auth context for CSC credentials"));
}
}
private static void validatePageOptions(CombinePDFJobInput input) {
PageRanges pageRanges = input.getPageRanges();
if (pageRanges == null) {
throw new IllegalArgumentException("No page options provided for combining files PDFs");
}
pageRanges.validate();
}
private static void validatePassword(String password, boolean isUserPassword, String encryptionAlgorithm) {
if (StringUtil.isEmpty(password)) {
throw new IllegalArgumentException(format("%s Password cannot be empty", isUserPassword ? USER_STRING :
OWNER_STRING));
}
if (password.length() > PASSWORD_MAX_LENGTH) {
throw new IllegalArgumentException(format("%s Password length cannot exceed %d bytes", isUserPassword ?
USER_STRING :
OWNER_STRING,
PASSWORD_MAX_LENGTH));
}
// Password validation for AES_128 encryption algorithm
if (encryptionAlgorithm.equals(EncryptionAlgorithm.AES_128.getValue())) {
if (!StandardCharsets.ISO_8859_1.newEncoder().canEncode(password)) {
throw new IllegalArgumentException(format("%s Password supports only LATIN-I characters for AES-128 " +
"encryption", isUserPassword ? USER_STRING :
OWNER_STRING));
}
}
}
public static void validateProtectPDFParams(ProtectPDFParams protectPDFParams) {
// Validations for PasswordProtectOptions
if (protectPDFParams instanceof PasswordProtectParams) {
PasswordProtectParams passwordProtectParams = (PasswordProtectParams) protectPDFParams;
// Validate encryption algo
if (passwordProtectParams.getEncryptionAlgorithm() == null) {
throw new IllegalArgumentException(String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL,
"Encryption algorithm"));
}
if (StringUtil.isNull(passwordProtectParams.getUserPassword()) && StringUtil.isNull(passwordProtectParams.getOwnerPassword())) {
throw new IllegalArgumentException("One of the password (user/owner) is required");
}
if (!StringUtil.isNull(passwordProtectParams.getOwnerPassword()) && !StringUtil.isNull(passwordProtectParams.getUserPassword())) {
if (passwordProtectParams.getOwnerPassword().equals(passwordProtectParams.getUserPassword())) {
throw new IllegalArgumentException("User and owner password cannot be same");
}
}
// Validate user password
if (!StringUtil.isNull(passwordProtectParams.getUserPassword()))
validatePassword(passwordProtectParams.getUserPassword(), true,
passwordProtectParams.getEncryptionAlgorithm()
.getValue());
// Validate owner password
if (!StringUtil.isNull(passwordProtectParams.getOwnerPassword()))
validatePassword(passwordProtectParams.getOwnerPassword(), false,
passwordProtectParams.getEncryptionAlgorithm()
.getValue());
// OwnerPassword is mandatory in case the permissions are provided
if (passwordProtectParams.getPermissions() != null && StringUtil.isNull(passwordProtectParams.getOwnerPassword()))
throw new IllegalArgumentException("The document permissions cannot be applied without setting owner " +
"password");
}
}
public static void validateInsertAssetInputs(Asset baseAsset,
Map> assetsToInsert) {
if (baseAsset == null) {
throw new IllegalArgumentException(String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL, "Base " +
"asset"));
}
if (assetsToInsert == null || assetsToInsert.isEmpty()) {
throw new IllegalArgumentException("No assets to insert in the base input asset");
}
for (Map.Entry> entry : assetsToInsert.entrySet()) {
if (entry.getKey() < 1) {
throw new IllegalArgumentException("Base file page should be greater than 0");
}
for (CombinePDFJobInput combinePDFJobInput : entry.getValue()) {
validatePageRanges(combinePDFJobInput.getPageRanges());
}
}
}
public static void validateReplaceFilesInputs(Asset baseAsset, Map assetsToReplace) {
if (baseAsset == null) {
throw new IllegalArgumentException(String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL, "Base " +
"asset"));
}
if (assetsToReplace == null || assetsToReplace.isEmpty()) {
throw new IllegalArgumentException("No assets to replace in the base input asset");
}
for (Map.Entry entry : assetsToReplace.entrySet()) {
if (entry.getKey() < 1) {
throw new IllegalArgumentException("Base asset page should be greater than 0");
}
validatePageRanges(entry.getValue().getPageRanges());
}
}
public static void validatePageRanges(PageRanges pageRanges) {
if (pageRanges == null || pageRanges.isEmpty()) {
throw new IllegalArgumentException("No page ranges were set for the operation");
}
pageRanges.validate();
}
public static void validatePageRangesOverlap(PageRanges pageRanges) {
// Creating a copy in case the pageRange order matters in the original pageRanges
List pageRangeList = new ArrayList<>();
pageRangeList.addAll(pageRanges.getRanges());
Collections.sort(pageRangeList, Comparator.comparingInt(PageRange::getStart));
for (int i = 1; i < pageRangeList.size(); i++) {
if (pageRangeList.get(i - 1).getEnd() == null || pageRangeList.get(i).getStart() <= pageRangeList.get(i - 1)
.getEnd()) {
throw new IllegalArgumentException("The overlapping page ranges are not allowed");
}
}
}
public static void validateRotatePageActions(List pageActions) {
if (pageActions.isEmpty()) {
throw new IllegalArgumentException("No rotation specified for the operation");
}
if (pageActions.size() > PAGE_ACTIONS_MAX_LIMIT) {
throw new IllegalArgumentException("Too many rotations not allowed.");
}
for (PageAction pageAction : pageActions) {
if (pageAction.getPageRanges().isEmpty()) {
throw new IllegalArgumentException("No page ranges were set for the operation");
}
}
}
public static void validatePasswordToRemoveProtection(String password) {
if (StringUtil.isBlank(password)) {
throw new IllegalArgumentException(String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL_OR_EMPTY,
"Password"));
}
if (password.length() > REMOVE_PROTECTION_PASSWORD_MAX_LENGTH) {
throw new IllegalArgumentException(format("Allowed maximum length of password is %d characters",
REMOVE_PROTECTION_PASSWORD_MAX_LENGTH));
}
}
public static void validateSplitPDFOperationParams(PageRanges pageRanges, Integer pageCount, Integer fileCount) {
if (pageRanges == null && pageCount == null && fileCount == null) {
throw new IllegalArgumentException("One of the options(page ranges/file count/page count) is required for" +
" splitting a PDF document");
}
if (pageRanges != null) {
if (pageCount != null || fileCount != null) {
throw new IllegalArgumentException("Only one of option (page ranges/page count/file count) can be " +
"specified for splitting a PDF document");
}
if (pageRanges.getRanges().size() > SPLIT_PDF_OUTPUT_COUNT_LIMIT) {
throw new IllegalArgumentException("Too many page ranges specified");
}
validatePageRangesOverlap(pageRanges);
pageRanges.validate();
return;
}
if (pageCount != null) {
if (fileCount != null) {
throw new IllegalArgumentException("Only one of option (page ranges/page count/file count) can be " +
"specified for splitting a PDF document");
}
if (pageCount <= 0) {
throw new IllegalArgumentException("Page count should be greater than 0");
}
return;
}
if (fileCount <= 0) {
throw new IllegalArgumentException("File count should be greater than 0");
}
if (fileCount > SPLIT_PDF_OUTPUT_COUNT_LIMIT) {
throw new IllegalArgumentException(format("Input PDF file cannot be split into more than %d documents",
SPLIT_PDF_OUTPUT_COUNT_LIMIT));
}
}
public static void validateDocumentMergeParams(DocumentMergeParams documentMergeParams) {
if (documentMergeParams.getJsonDataForMerge() == null) {
throw new IllegalArgumentException(String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL, "Input JSON" +
" data"));
}
if (documentMergeParams.getJsonDataForMerge().length() == 0) {
throw new IllegalArgumentException(String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL_OR_EMPTY,
"Input JSON Data"));
}
if (documentMergeParams.getOutputFormat() == null) {
throw new IllegalArgumentException(String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL, "Output " +
"format"));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy