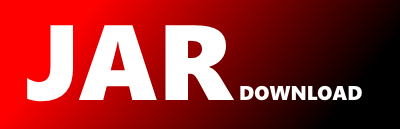
com.adobe.pdfservices.operation.pdfjobs.params.combinepdf.CombinePDFParams Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdfservices-sdk Show documentation
Show all versions of pdfservices-sdk Show documentation
Adobe PDF Services SDK allows you to access RESTful APIs to create, convert, and manipulate PDFs within your applications.
Older versions can be found under groupId: com.adobe.documentservices, artifactId: pdftools-sdk
/*
* Copyright 2024 Adobe
* All Rights Reserved.
*
* NOTICE: Adobe permits you to use, modify, and distribute this file in
* accordance with the terms of the Adobe license agreement accompanying
* it. If you have received this file from a source other than Adobe,
* then your use, modification, or distribution of it requires the prior
* written permission of Adobe.
*/
package com.adobe.pdfservices.operation.pdfjobs.params.combinepdf;
import com.adobe.pdfservices.operation.internal.params.CombinePDFJobInput;
import com.adobe.pdfservices.operation.internal.util.ObjectUtil;
import com.adobe.pdfservices.operation.internal.util.ValidationUtil;
import com.adobe.pdfservices.operation.io.Asset;
import com.adobe.pdfservices.operation.pdfjobs.jobs.CombinePDFJob;
import com.adobe.pdfservices.operation.pdfjobs.params.PDFServicesJobParams;
import com.adobe.pdfservices.operation.pdfjobs.params.PageRanges;
import java.util.ArrayList;
import java.util.List;
/**
* Parameters for combining PDFs using {@link CombinePDFJob}.
*/
public class CombinePDFParams implements PDFServicesJobParams {
private List assetsToCombine;
private CombinePDFParams(Builder builder) {
this.assetsToCombine = builder.assetsToCombine;
}
/**
* Returns a list of {@link CombinePDFJobInput} instances.
*
* @return a list of {@link CombinePDFJobInput} instances
*/
public List getFilesToCombine() {
return assetsToCombine;
}
/**
* Creates a new instance of {@link Builder}.
*
* @return a {@link Builder} instance
*/
public static Builder combinePDFParamsBuilder() {
return new Builder();
}
/**
* Builds a {@link CombinePDFParams} instance.
*/
public static class Builder {
private List assetsToCombine;
/**
* Constructs a {@code CompressPDFParams.Builder} instance.
*/
public Builder() {
assetsToCombine = new ArrayList<>();
}
/**
* Adds an {@link Asset} to combine.
*
* @param asset see {@link Asset}.
* @return this Builder instance to add any additional parameters
*/
public Builder addAsset(Asset asset) {
ObjectUtil.requireNonNull(asset, "No input was set for job");
PageRanges pageRanges = new PageRanges();
pageRanges.addAll();
assetsToCombine.add(new CombinePDFJobInput(asset, pageRanges));
return this;
}
/**
* Adds an {@link Asset} with {@link PageRanges}to combine.
*
* @param asset see {@link Asset}.
* @param pageRanges see {@link PageRanges}.
* @return this Builder instance to add any additional parameters
*/
public Builder addAsset(Asset asset, PageRanges pageRanges) {
ObjectUtil.requireNonNull(asset, "No input was set for job");
ObjectUtil.requireNonNull(pageRanges, "No page options was provided for input file");
assetsToCombine.add(new CombinePDFJobInput(asset, pageRanges));
return this;
}
/**
* Returns a new {@link CombinePDFParams} instance built from the current state of this builder.
*
* @return a {@code CombinePDFParams} instance
*/
public CombinePDFParams build() {
if (this.assetsToCombine.isEmpty()) {
throw new IllegalArgumentException("No input was provided for combining files");
}
Class extends Asset> firstAssetType = assetsToCombine.stream().findFirst().get().getAsset().getClass();
assetsToCombine.forEach(file -> {
if (!firstAssetType.equals(file.getAsset().getClass())) {
throw new IllegalArgumentException("All assets are not of same type");
}
ValidationUtil.validateAssetWithPageOptions(file);
});
if (assetsToCombine.stream().map(CombinePDFJobInput::getAsset).count() > 20) {
throw new IllegalArgumentException("Only 20 input assets can be combined in one combine job instance");
}
return new CombinePDFParams(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy