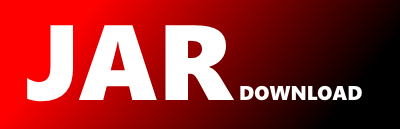
com.adobe.pdfservices.operation.pdfjobs.params.documentmerge.DocumentMergeParams Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdfservices-sdk Show documentation
Show all versions of pdfservices-sdk Show documentation
Adobe PDF Services SDK allows you to access RESTful APIs to create, convert, and manipulate PDFs within your applications.
Older versions can be found under groupId: com.adobe.documentservices, artifactId: pdftools-sdk
/*
* Copyright 2024 Adobe
* All Rights Reserved.
*
* NOTICE: Adobe permits you to use, modify, and distribute this file in
* accordance with the terms of the Adobe license agreement accompanying
* it. If you have received this file from a source other than Adobe,
* then your use, modification, or distribution of it requires the prior
* written permission of Adobe.
*/
package com.adobe.pdfservices.operation.pdfjobs.params.documentmerge;
import com.adobe.pdfservices.operation.internal.constants.CustomErrorMessages;
import com.adobe.pdfservices.operation.internal.util.ObjectUtil;
import com.adobe.pdfservices.operation.internal.util.ValidationUtil;
import com.adobe.pdfservices.operation.pdfjobs.jobs.DocumentMergeJob;
import com.adobe.pdfservices.operation.pdfjobs.params.PDFServicesJobParams;
import org.json.JSONObject;
import java.util.Objects;
/**
* Parameters for document generation using {@link DocumentMergeJob}.
*/
public class DocumentMergeParams implements PDFServicesJobParams {
private JSONObject jsonDataForMerge;
private OutputFormat outputFormat;
private Fragments fragments;
private DocumentMergeParams(Builder builder) {
this.jsonDataForMerge = builder.jsonDataForMerge;
this.outputFormat = builder.outputFormat;
this.fragments = builder.fragments;
}
/**
* Represents the input datasource for the document merge process as a JSON Object
*
* @return the input JSON Data to be merged with document template
*/
public JSONObject getJsonDataForMerge() {
return jsonDataForMerge;
}
/**
* Returns the intended {@link OutputFormat} of the generated document.
*
* @return the {@link OutputFormat}
*/
public OutputFormat getOutputFormat() {
return outputFormat;
}
/**
* Returns the input {@link Fragments} data for document merge process.
*
* @return the input {@link Fragments} data for the document merge process
*/
public Fragments getFragments() {
return fragments;
}
/**
* Creates a new {@link Builder}.
*
* @return a {@link Builder} instance
*/
public static Builder documentMergeParamsBuilder() {
return new Builder();
}
/**
* Builds a {@link DocumentMergeParams} instance.
*/
public static class Builder {
private JSONObject jsonDataForMerge;
private OutputFormat outputFormat;
private Fragments fragments;
/**
* Constructs a {@code CompressPDFParams.Builder} instance.
*/
public Builder() {
}
/**
* Sets the input JSON data for the document merge process.
*
* @param jsonDataForMerge json to be used in the document merge job; can not be null.
* @return this Builder instance to add any additional parameters
*/
public Builder withJsonDataForMerge(JSONObject jsonDataForMerge) {
ObjectUtil.requireNonNull(jsonDataForMerge, String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL,
"Json Data For Merge"));
this.jsonDataForMerge = jsonDataForMerge;
return this;
}
/**
* Sets the {@link OutputFormat} for the document merge process.
*
* @param outputFormat output format for the document merge result; can not be null.
* @return this Builder instance to add any additional parameters
*/
public Builder withOutputFormat(OutputFormat outputFormat) {
ObjectUtil.requireNonNull(outputFormat, String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL,
"Output Format"));
this.outputFormat = outputFormat;
return this;
}
/**
* Sets the {@link Fragments} for the document merge process.
*
* @param fragments fragments for document merge job; can not be null.
* @return this Builder instance to add any additional parameters
*/
public Builder withFragments(Fragments fragments) {
ObjectUtil.requireNonNull(fragments, String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL,
"Fragments"));
this.fragments = fragments;
return this;
}
/**
* Returns a new {@link DocumentMergeParams} instance built from the current state of this builder.
*
* @return a new {@code DocumentMergeParams} instance
*/
public DocumentMergeParams build() {
this.outputFormat = Objects.isNull(this.outputFormat) ? OutputFormat.PDF : this.outputFormat;
DocumentMergeParams documentMergeParams = new DocumentMergeParams(this);
ValidationUtil.validateDocumentMergeParams(documentMergeParams);
return documentMergeParams;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy