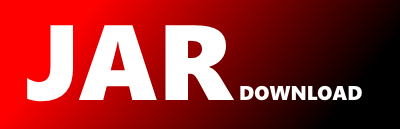
com.adobe.pdfservices.operation.pdfjobs.params.electronicseal.CSCCredential Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdfservices-sdk Show documentation
Show all versions of pdfservices-sdk Show documentation
Adobe PDF Services SDK allows you to access RESTful APIs to create, convert, and manipulate PDFs within your applications.
Older versions can be found under groupId: com.adobe.documentservices, artifactId: pdftools-sdk
/*
* Copyright 2024 Adobe
* All Rights Reserved.
*
* NOTICE: Adobe permits you to use, modify, and distribute this file in
* accordance with the terms of the Adobe license agreement accompanying
* it. If you have received this file from a source other than Adobe,
* then your use, modification, or distribution of it requires the prior
* written permission of Adobe.
*/
package com.adobe.pdfservices.operation.pdfjobs.params.electronicseal;
/**
* Parameters for representing the CSC Provider based credentials as a subtype
* for {@link CertificateCredentials}.
*/
public class CSCCredential extends CertificateCredentials {
private String providerName;
private String credentialID;
private String pin;
private CSCAuthContext cscAuthContext;
private CSCCredential(Builder builder) {
this.providerName = builder.providerName;
this.credentialID = builder.credentialID;
this.pin = builder.pin;
this.cscAuthContext = builder.cscAuthContext;
}
/**
* Returns the name of trust service provider.
*
* @return trust service provider name
*/
public String getProviderName() {
return providerName;
}
/**
* Returns the certificate credential ID of user.
*
* @return credential ID
*/
public String getCredentialID() {
return credentialID;
}
/**
* Returns the PIN to be used for validating credentials.
*
* @return the PIN value
*/
public String getPin() {
return pin;
}
/**
* Returns the credential authorization parameters.
*
* @return the credential auth params
*/
public CSCAuthContext getCscAuthContext() {
return cscAuthContext;
}
/**
* Builds a {@link CSCCredential} instance.
*/
public static class Builder {
private String providerName;
private String credentialID;
private String pin;
private CSCAuthContext cscAuthContext;
/**
* Constructs a {@code CSCCredential.Builder} instance
*/
protected Builder() {
}
/**
* Sets the name of trust service provider being used.
*
* @param providerName the provider name
* @return this Builder instance to add any additional parameters
*/
public Builder withProviderName(String providerName) {
this.providerName = providerName;
return this;
}
/**
* Sets the TSP credential ID.
* It is the digital ID stored with the TSP provider that should be used for sealing.
*
* @param credentialID the credential ID
* @return this Builder instance to add any additional parameters
*/
public Builder withCredentialID(String credentialID) {
this.credentialID = credentialID;
return this;
}
/**
* Sets the PIN associated with credential ID.
*
* @param pin the pin
* @return this Builder instance to add any additional parameters
*/
public Builder withPin(String pin) {
this.pin = pin;
return this;
}
/**
* Sets the CSC auth context.
* It encapsulates the service authorization data required to communicate with the TSP and access CSC
* provider APIs.
*
* @param cscAuthContext the CSC auth context
* @return this Builder instance to add any additional parameters
*/
public Builder withCSCAuthContext(CSCAuthContext cscAuthContext) {
this.cscAuthContext = cscAuthContext;
return this;
}
/**
* Returns a new {@link CSCCredential} instance built from the current state of this builder.
*
* @return a new {@code CSCCredential} instance
*/
public CSCCredential build() {
return new CSCCredential(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy