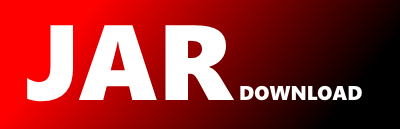
com.adobe.pdfservices.operation.pdfjobs.params.extractpdf.ExtractPDFParams Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdfservices-sdk Show documentation
Show all versions of pdfservices-sdk Show documentation
Adobe PDF Services SDK allows you to access RESTful APIs to create, convert, and manipulate PDFs within your applications.
Older versions can be found under groupId: com.adobe.documentservices, artifactId: pdftools-sdk
/*
* Copyright 2024 Adobe
* All Rights Reserved.
*
* NOTICE: Adobe permits you to use, modify, and distribute this file in
* accordance with the terms of the Adobe license agreement accompanying
* it. If you have received this file from a source other than Adobe,
* then your use, modification, or distribution of it requires the prior
* written permission of Adobe.
*/
package com.adobe.pdfservices.operation.pdfjobs.params.extractpdf;
import com.adobe.pdfservices.operation.internal.util.CollectionUtil;
import com.adobe.pdfservices.operation.pdfjobs.jobs.ExtractPDFJob;
import com.adobe.pdfservices.operation.pdfjobs.params.PDFServicesJobParams;
import java.util.ArrayList;
import java.util.List;
/**
* Parameters to extract content from PDF using {@link ExtractPDFJob}.
*/
public class ExtractPDFParams implements PDFServicesJobParams {
private TableStructureType tableStructureType;
private boolean addCharInfo;
private boolean getStylingInfo;
private List elementsToExtractRenditions;
private List elementsToExtract;
private ExtractPDFParams(Builder builder) {
this.elementsToExtractRenditions = builder.elementsToExtractRenditions;
this.elementsToExtract = builder.elementsToExtract;
this.addCharInfo = builder.addCharInfo;
this.tableStructureType = builder.tableStructureType;
this.getStylingInfo = builder.getStylingInfo;
}
/**
* Returns the {@link TableStructureType} of the resulting rendition
*
* @return the table structure type
*/
public TableStructureType getTableStructureType() {
return tableStructureType;
}
/**
* Returns {@link Boolean}, whether character level information was invoked for operation.
*
* @return the char info boolean
*/
public Boolean getAddCharInfo() {
return addCharInfo;
}
/**
* Returns {@link Boolean}, whether styling information was invoked for operation.
*
* @return the include styling boolean
*/
public Boolean getStylingInfo() {
return getStylingInfo;
}
/**
* Returns the list of {@link ExtractRenditionsElementType} invoked for job.
*
* @return the list of {@link ExtractRenditionsElementType}
*/
public List getElementsToExtractRenditions() {
return elementsToExtractRenditions;
}
/**
* Returns the list of elements (Text and/or Tables) invoked for operation
*
* @return the list of elements to extract
*/
public List getElementsToExtract() {
return elementsToExtract;
}
/**
* Creates a new {@link Builder}.
*
* @return a {@code ExtractPDFParams.Builder} instance
*/
public static Builder extractPDFParamsBuilder() {
return new Builder();
}
/**
* Static Builder class for Extract PDF Params
*/
public static class Builder {
private TableStructureType tableStructureType;
private boolean addCharInfo;
private boolean getStylingInfo;
private List elementsToExtractRenditions =
new ArrayList();
private List elementsToExtract = new ArrayList();
/**
* Constructs a {@code Builder} instance.
*/
private Builder() {
}
/**
* Adds an {@link ExtractElementType} to the list of elements to be extracted.
*
* @param elementToExtract {@link ExtractElementType} parameter
* @return this Builder instance to add any additional parameters
*/
public Builder addElementToExtract(ExtractElementType elementToExtract) {
if (elementToExtract != null) {
this.elementsToExtract.add(elementToExtract);
}
return this;
}
/**
* Adds {@link ExtractElementType} to the list of elements to be extracted.
*
* @param elementsToExtract The list of {@link ExtractElementType} to extract
* @return this Builder instance to add any additional parameters
*/
public Builder addElementsToExtract(List elementsToExtract) {
if (!CollectionUtil.isEmpty(elementsToExtract)) {
this.elementsToExtract.addAll(elementsToExtract);
}
return this;
}
/**
* Adds a {@link ExtractRenditionsElementType}
*
* @param elementToExtractRenditions the {@link ExtractRenditionsElementType} parameter
* @return this Builder instance to add any additional parameters
*/
public Builder addElementToExtractRenditions(ExtractRenditionsElementType elementToExtractRenditions) {
if (elementToExtractRenditions != null) {
this.elementsToExtractRenditions.add(elementToExtractRenditions);
}
return this;
}
/**
* Adds {@link ExtractRenditionsElementType} to the list of elements to be extracted.
*
* @param elementsToExtractRenditions The list of {@link ExtractRenditionsElementType} to extract
* @return this Builder instance to add any additional parameters
*/
public Builder addElementsToExtractRenditions(List elementsToExtractRenditions) {
if (!CollectionUtil.isEmpty(elementsToExtractRenditions)) {
this.elementsToExtractRenditions.addAll(elementsToExtractRenditions);
}
return this;
}
/**
* Adds the {@link TableStructureType} for table renditions
*
* @param tableStructureType {@link TableStructureType} for output type of table structure.
* @return this Builder instance to add any additional parameters
*/
public Builder addTableStructureFormat(TableStructureType tableStructureType) {
this.tableStructureType = tableStructureType;
return this;
}
/**
* Boolean specifying whether to add character level bounding boxes to output json
*
* @param addCharInfo Boolean
* @return this Builder instance to add any additional parameters
*/
public Builder addCharInfo(Boolean addCharInfo) {
this.addCharInfo = addCharInfo;
return this;
}
/**
* {@link Boolean} specifying whether to add styling information to output json
*
* @param getStylingInfo {@link Boolean}
* @return this Builder instance to add any additional parameters
*/
public Builder addGetStylingInfo(Boolean getStylingInfo) {
this.getStylingInfo = getStylingInfo;
return this;
}
/**
* Returns a new {@link ExtractPDFParams} instance built from the current state of this builder.
*
* @return a new {@code ExtractPDFParams} instance
*/
public ExtractPDFParams build() {
return new ExtractPDFParams(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy