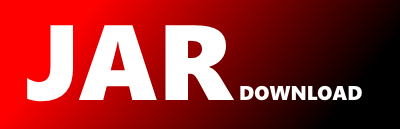
com.adobe.pdfservices.operation.pdfjobs.params.insertpages.InsertPagesParams Maven / Gradle / Ivy
Show all versions of pdfservices-sdk Show documentation
/*
* Copyright 2024 Adobe
* All Rights Reserved.
*
* NOTICE: Adobe permits you to use, modify, and distribute this file in
* accordance with the terms of the Adobe license agreement accompanying
* it. If you have received this file from a source other than Adobe,
* then your use, modification, or distribution of it requires the prior
* written permission of Adobe.
*/
package com.adobe.pdfservices.operation.pdfjobs.params.insertpages;
import com.adobe.pdfservices.operation.internal.constants.CustomErrorMessages;
import com.adobe.pdfservices.operation.internal.params.CombinePDFJobInput;
import com.adobe.pdfservices.operation.internal.util.ObjectUtil;
import com.adobe.pdfservices.operation.internal.util.ValidationUtil;
import com.adobe.pdfservices.operation.io.Asset;
import com.adobe.pdfservices.operation.pdfjobs.jobs.InsertPagesPDFJob;
import com.adobe.pdfservices.operation.pdfjobs.params.PDFServicesJobParams;
import com.adobe.pdfservices.operation.pdfjobs.params.PageRanges;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.TreeMap;
/**
* Parameters for inserting pages in a PDF using {@link InsertPagesPDFJob}.
*/
public class InsertPagesParams implements PDFServicesJobParams {
private Map> assetsToInsert;
private Asset baseAsset;
private InsertPagesParams(Builder builder) {
this.baseAsset = builder.baseAsset;
this.assetsToInsert = builder.assetsToInsert;
}
/**
* Returns the mapping of base {@link Asset Asset's} page number and {@link Asset} to be inserted along with
* {@link PageRanges}.
*
* @return mapping of base {@link Asset} page number and {@link Asset} to be inserted along with {@link PageRanges}.
*/
public Map> getAssetsToInsert() {
return assetsToInsert;
}
/**
* Returns the base PDF {@link Asset} to which pages will be inserted.
*
* @return an {@link Asset} instance
*/
public Asset getBaseAsset() {
return baseAsset;
}
/**
* Creates a new {@link Builder}.
*
* @return a {@link Builder} instance
*/
public static Builder insertPagesParamsBuilder(Asset asset) {
return new Builder(asset);
}
/**
* Builds a {@link InsertPagesParams} instance.
*/
public static class Builder {
private Map> assetsToInsert;
private Asset baseAsset;
/**
* Constructs a {@code Builder} instance.
*/
public Builder(Asset asset) {
ObjectUtil.requireNonNull(asset, String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL, "Base asset"));
this.baseAsset = asset;
this.assetsToInsert = new TreeMap<>();
}
/**
* Adds all the pages of the input PDF file to be inserted at the specified page of the base PDF file.
*
* This method can be invoked multiple times with the same or different input PDF files.
*
* @param inputAsset a PDF file for insertion; can not be null
* @param basePage page of the base PDF file
*/
public Builder addPagesToInsertAt(Asset inputAsset, Integer basePage) {
ObjectUtil.requireNonNull(inputAsset, String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL, "Input " +
"Asset"));
ObjectUtil.requireNonNull(basePage, String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL, "Base " +
"Page"));
PageRanges pageRanges = new PageRanges();
pageRanges.addAll();
addPagesToInsertAt(inputAsset, pageRanges, basePage);
return this;
}
/**
* Adds the specified pages of the input PDF file to be inserted at the specified page of the base PDF file
*
* This method can be invoked multiple times with the same or different input PDF files.
*
* @param inputAsset a PDF file for insertion; can not be null.
* @param pageRanges page ranges of the input PDF file; can not be null.
* @param basePage page of the base PDF file; can not be null.
*/
public Builder addPagesToInsertAt(Asset inputAsset, PageRanges pageRanges, Integer basePage) {
ObjectUtil.requireNonNull(inputAsset, String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL, "Input " +
"asset"));
ObjectUtil.requireNonNull(pageRanges, String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL, "Page " +
"ranges"));
ObjectUtil.requireNonNull(basePage, String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL, "Base " +
"page"));
CombinePDFJobInput combinePDFJobInput = new CombinePDFJobInput(inputAsset, pageRanges);
updateFilesToInsert(basePage, combinePDFJobInput);
return this;
}
private void updateFilesToInsert(Integer index, CombinePDFJobInput combinePDFJobInput) {
if (assetsToInsert.containsKey(index)) {
List inputList = assetsToInsert.get(index);
inputList.add(combinePDFJobInput);
} else {
List inputList = new ArrayList<>();
inputList.add(combinePDFJobInput);
assetsToInsert.put(index, inputList);
}
}
/**
* Returns a new {@link InsertPagesParams} instance built from the current state of this builder.
*
* @return a new {@code InsertPagesParams} instance
*/
public InsertPagesParams build() {
ValidationUtil.validateInsertAssetInputs(this.baseAsset, this.assetsToInsert);
return new InsertPagesParams(this);
}
}
}