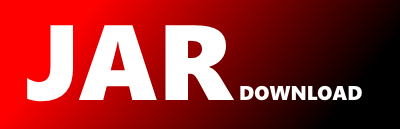
com.adobe.pdfservices.operation.pdfjobs.params.protectpdf.PasswordProtectParams Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdfservices-sdk Show documentation
Show all versions of pdfservices-sdk Show documentation
Adobe PDF Services SDK allows you to access RESTful APIs to create, convert, and manipulate PDFs within your applications.
Older versions can be found under groupId: com.adobe.documentservices, artifactId: pdftools-sdk
/*
* Copyright 2024 Adobe
* All Rights Reserved.
*
* NOTICE: Adobe permits you to use, modify, and distribute this file in
* accordance with the terms of the Adobe license agreement accompanying
* it. If you have received this file from a source other than Adobe,
* then your use, modification, or distribution of it requires the prior
* written permission of Adobe.
*/
package com.adobe.pdfservices.operation.pdfjobs.params.protectpdf;
import com.adobe.pdfservices.operation.internal.constants.CustomErrorMessages;
import com.adobe.pdfservices.operation.internal.util.ObjectUtil;
import com.adobe.pdfservices.operation.internal.util.ValidationUtil;
import com.adobe.pdfservices.operation.pdfjobs.jobs.ProtectPDFJob;
/**
* Parameters for securing PDF file with passwords and document permissions using {@link ProtectPDFJob}.
*/
public class PasswordProtectParams extends ProtectPDFParams {
private String userPassword;
private String ownerPassword;
private EncryptionAlgorithm encryptionAlgorithm;
private ContentEncryption contentEncryption;
private Permissions permissions;
private PasswordProtectParams(Builder builder) {
this.userPassword = builder.userPassword;
this.ownerPassword = builder.ownerPassword;
this.encryptionAlgorithm = builder.encryptionAlgorithm;
this.contentEncryption = builder.contentEncryption;
this.permissions = builder.permissions;
}
/**
* Returns the intended user password of the resulting encrypted PDF file.
*
* @return the user password
*/
public String getUserPassword() {
return userPassword;
}
/**
* Returns the intended owner password of the resulting encrypted PDF file.
*
* @return the owner password
*/
public String getOwnerPassword() {
return ownerPassword;
}
/**
* Returns the intended {@link EncryptionAlgorithm} of the resulting encrypted PDF file.
*
* @return the encryption algorithm
*/
public EncryptionAlgorithm getEncryptionAlgorithm() {
return encryptionAlgorithm;
}
/**
* Returns the intended type of {@link ContentEncryption} for the resulting encrypted PDF file.
*
* @return the type of content to encrypt
*/
public ContentEncryption getContentEncryption() {
return contentEncryption;
}
/**
* Returns the intended type of {@link ContentEncryption} for the resulting encrypted PDF file as a string.
*
* @return the type of content to encrypt as a string
*/
public String getContentEncryptionAsString() {
String contentEncryptionAsString = null;
if (contentEncryption != null)
contentEncryptionAsString = contentEncryption.getValue();
return contentEncryptionAsString;
}
/**
* Returns the intended document {@link Permissions} for the resulting encrypted PDF file.
*
* @return the document permissions
*/
public Permissions getPermissions() {
return permissions;
}
/**
* Builds a {@link PasswordProtectParams} instance.
*/
public static class Builder {
private String userPassword;
private String ownerPassword;
private EncryptionAlgorithm encryptionAlgorithm;
private ContentEncryption contentEncryption;
private Permissions permissions;
/**
* Constructs a {@code Builder} instance.
*/
public Builder() {
}
/**
* Sets the intended user password required for opening the encrypted PDF file. Allowed maximum length for
* the user
* password is 128 bytes.
*
* @param userPassword the user password; can not be null or empty
* @return this Builder instance to add any additional parameters
*/
public Builder setUserPassword(String userPassword) {
ObjectUtil.requireNonNull(userPassword, String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL, "User " +
"password"));
this.userPassword = userPassword;
return this;
}
/**
* Sets the intended owner password required to control access permissions in the encrypted PDF file. This
* password can also be used to open/view the encrypted PDF file. Allowed maximum length for the owner
* password is 128
* bytes.
*
* @param ownerPassword the owner password; can not be null or empty
* @return this Builder instance to add any additional parameters
*/
public Builder setOwnerPassword(String ownerPassword) {
ObjectUtil.requireNonNull(ownerPassword, String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL,
"Owner password"));
this.ownerPassword = ownerPassword;
return this;
}
/**
* Sets the intended encryption algorithm required for encrypting the PDF file. For AES-128 encryption, the
* password
* supports LATIN-I characters only. For AES-256 encryption, passwords supports Unicode character set.
*
* @param encryptionAlgorithm the encryption algorithm; can not be null
* @return this Builder instance to add any additional parameters
*/
public Builder setEncryptionAlgorithm(EncryptionAlgorithm encryptionAlgorithm) {
this.encryptionAlgorithm = encryptionAlgorithm;
return this;
}
/**
* Sets the intended type of content to encrypt in the PDF file.
*
* @param contentEncryption the type of content to encrypt; can not be null
* @return this Builder instance to add any additional parameters
*/
public Builder setContentEncryption(ContentEncryption contentEncryption) {
ObjectUtil.requireNonNull(contentEncryption, String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL,
"Content encryption"));
this.contentEncryption = contentEncryption;
return this;
}
/**
* Sets the intended permissions for the encrypted PDF file. This includes permissions to allow printing,
* editing
* and content copying in the PDF document. Permissions can only be used in case the owner password is set.
*
* @param permissions the permissions; can not be null
* @return this Builder instance to add any additional parameters
*/
public Builder setPermissions(Permissions permissions) {
ObjectUtil.requireNonNull(permissions, String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL,
"Permissions"));
this.permissions = permissions;
return this;
}
/**
* Returns a new {@link PasswordProtectParams} instance built from the current state of this builder.
*
* @return a new {@link ProtectPDFParams} instance
*/
public PasswordProtectParams build() {
PasswordProtectParams passwordProtectParams = new PasswordProtectParams(this);
ValidationUtil.validateProtectPDFParams(passwordProtectParams);
return passwordProtectParams;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy