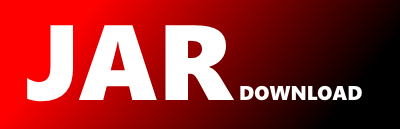
com.adobe.pdfservices.operation.pdfjobs.params.replacepages.ReplacePagesParams Maven / Gradle / Ivy
Show all versions of pdfservices-sdk Show documentation
/*
* Copyright 2024 Adobe
* All Rights Reserved.
*
* NOTICE: Adobe permits you to use, modify, and distribute this file in
* accordance with the terms of the Adobe license agreement accompanying
* it. If you have received this file from a source other than Adobe,
* then your use, modification, or distribution of it requires the prior
* written permission of Adobe.
*/
package com.adobe.pdfservices.operation.pdfjobs.params.replacepages;
import com.adobe.pdfservices.operation.internal.constants.CustomErrorMessages;
import com.adobe.pdfservices.operation.internal.params.CombinePDFJobInput;
import com.adobe.pdfservices.operation.internal.util.ObjectUtil;
import com.adobe.pdfservices.operation.internal.util.ValidationUtil;
import com.adobe.pdfservices.operation.io.Asset;
import com.adobe.pdfservices.operation.pdfjobs.jobs.ReplacePagesPDFJob;
import com.adobe.pdfservices.operation.pdfjobs.params.PDFServicesJobParams;
import com.adobe.pdfservices.operation.pdfjobs.params.PageRanges;
import java.util.Map;
import java.util.TreeMap;
/**
* Parameters for replacing pages of a pdf using {@link ReplacePagesPDFJob}.
*/
public class ReplacePagesParams implements PDFServicesJobParams {
private Map assetsToReplace;
private Asset baseAsset;
private ReplacePagesParams(Builder builder) {
this.baseAsset = builder.baseAsset;
this.assetsToReplace = builder.assetsToReplace;
}
/**
* Returns the mapping of base {@link Asset Asset's} page number and {@link Asset} to be replaced along with
* {@link PageRanges}.
*
* @return mapping of base {@link Asset} page number and {@link Asset} to be replaced along with {@link PageRanges}.
*/
public Map getAssetsToReplace() {
return assetsToReplace;
}
/**
* Returns the base PDF {@link Asset} to which pages will be replaced.
*
* @return an {@link Asset} instance
*/
public Asset getBaseAsset() {
return baseAsset;
}
/**
* Creates a new {@link Builder}.
*
* @return a {@link Builder} instance
*/
public static Builder replacePagesParamsBuilder(Asset asset) {
return new Builder(asset);
}
/**
* Builds a {@link ReplacePagesParams} instance.
*/
public static class Builder {
private Map assetsToReplace;
private Asset baseAsset;
/**
* Constructs a {@code ReplacePagesParams.Builder} instance.
*/
public Builder(Asset asset) {
ObjectUtil.requireNonNull(asset, String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL, "Base asset"));
this.baseAsset = asset;
this.assetsToReplace = new TreeMap<>();
}
/**
* Adds all the pages of the input PDF file to be replaced at the specified page of the base PDF file.
*
* This method can be invoked multiple times with the same or different input PDF files.
*
* @param inputAsset a PDF file for insertion; can not be null
* @param basePage page of the base PDF file
*/
public Builder addPagesForReplace(Asset inputAsset, Integer basePage) {
ObjectUtil.requireNonNull(inputAsset, String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL, "Input " +
"asset"));
ObjectUtil.requireNonNull(basePage, String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL, "Base " +
"page"));
PageRanges pageRanges = new PageRanges();
pageRanges.addAll();
addPagesForReplace(inputAsset, pageRanges, basePage);
return this;
}
/**
* Adds the specified pages of the input PDF file to be replaced at the specified page of the base PDF file
*
* This method can be invoked multiple times with the same or different input PDF files.
*
* @param inputAsset a PDF file for insertion; can not be null
* @param pageRanges page ranges of the input PDF file; can not be null or empty
* @param basePage page of the base PDF file
*/
public Builder addPagesForReplace(Asset inputAsset, PageRanges pageRanges, Integer basePage) {
ObjectUtil.requireNonNull(inputAsset, String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL, "Input " +
"asset"));
ObjectUtil.requireNonNull(pageRanges, String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL, "Page " +
"ranges"));
ObjectUtil.requireNonNull(basePage, String.format(CustomErrorMessages.GENERIC_CAN_NOT_BE_NULL, "Base " +
"page"));
CombinePDFJobInput combinePDFJobInput = new CombinePDFJobInput(inputAsset, pageRanges);
assetsToReplace.put(basePage, combinePDFJobInput);
return this;
}
/**
* Returns a new {@link ReplacePagesParams} instance built from the current state of this builder.
*
* @return a new {@link ReplacePagesParams} instance
*/
public ReplacePagesParams build() {
ValidationUtil.validateReplaceFilesInputs(this.baseAsset, this.assetsToReplace);
return new ReplacePagesParams(this);
}
}
}