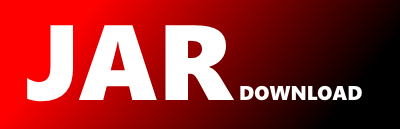
com.adobe.granite.crx2oak.profile.save.FilesystemProfileWriter Maven / Gradle / Ivy
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2016 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.adobe.granite.crx2oak.profile.save;
import com.adobe.granite.crx2oak.profile.ProfileStorageFormatConstants;
import com.adobe.granite.crx2oak.profile.model.osgi.OsgiConfiguration;
import com.adobe.granite.crx2oak.profile.model.template.RawProfileTemplate;
import com.google.common.base.Function;
import com.google.common.base.Joiner;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import static com.adobe.granite.crx2oak.profile.ProfileStorageFormatConstants.PROFILE_CHARSET;
import static com.google.common.base.Preconditions.checkNotNull;
import static com.google.common.collect.Iterables.transform;
/**
* The filesystem originated profile writer that stores the whole profile and all components to the filesystem
* for purpose of reading it back, archiving and examining.
*
* Future improvement might be to split OSGi configuration writer, Tags definition writer and command line writer
* into separate writer classes defined here as dependencies.
*/
public class FilesystemProfileWriter implements ProfileWriter {
private static final Logger LOGGER = LoggerFactory.getLogger(FilesystemProfileWriter.class);
private static final String THE_DIRECTORY_HASN_T_BEEN_CREATED_BY_SYSTEM_EXCEPTION_MESSAGE = "The directory hasn't been created by system.";
private static final char COMMAND_OPTION_OR_ARGUMENT_SEPARATOR = ' ';
private static final String SPACE = " ";
private static final String QUOTE_STRING = "\"";
@Override
public void write(@Nonnull final RawProfileTemplate rawProfileTemplateToWrite,
@Nonnull final String profileLocation)
throws ProfileWriteException {
checkNotNull(rawProfileTemplateToWrite, "The profile template to write cannot be null");
checkNotNull(profileLocation, "The new profile location to safe cannot be null");
File newProfileDirectory = new File(profileLocation);
checkForExistingFile(newProfileDirectory);
createProfile(newProfileDirectory, rawProfileTemplateToWrite);
}
private void createProfile(final File newProfileDirectory, final RawProfileTemplate rawProfileTemplateToWrite) {
createProfileDirectory(newProfileDirectory);
File osgiConfigurationsDirectory = createOsgiConfigurationsDirectory(newProfileDirectory);
createOsgiConfigurations(osgiConfigurationsDirectory, rawProfileTemplateToWrite.getOsgiConfigurationsTemplates());
File tagsDefinitionsDirectory = createTagsDefinitionsDirectory(newProfileDirectory);
createTagsDefinitions(tagsDefinitionsDirectory, rawProfileTemplateToWrite.getTagsWithValues());
createCommandLineFile(newProfileDirectory, rawProfileTemplateToWrite.getTemplatedCommandLineOptionsAndArguments());
}
private void createTagsDefinitions(final File tagsDefinitionsDirectory, final Map tagsWithValues) {
for (Map.Entry tagDefinition : tagsWithValues.entrySet()) {
createTagFile(tagsDefinitionsDirectory, tagDefinition);
}
}
private void createTagFile(final File tagsDefinitionsDirectory, final Map.Entry tagDefinition) {
File tagFile = new File(tagsDefinitionsDirectory, tagDefinition.getKey());
writeTagFile(tagDefinition.getValue(), tagFile);
}
private void writeTagFile(final String value, final File tagFile) {
try {
writeFileContent(value, tagFile.getAbsolutePath());
} catch (IOException e) {
LOGGER.error("Cannot write tag definition ({}) into profile.",
tagFile.getAbsolutePath());
throw new ProfileWriteException("Cannot write tag definition file: "
+ tagFile.getAbsolutePath(), e);
}
}
private void createOsgiConfigurations(final File osgiConfigurationsDirectory,
final Collection osgiConfigurationsTemplates) {
for (OsgiConfiguration osgiConfiguration : osgiConfigurationsTemplates) {
createOsgiConfigurationFile(osgiConfigurationsDirectory, osgiConfiguration);
}
}
private void createOsgiConfigurationFile(final File osgiConfigurationsDirectory,
final OsgiConfiguration osgiConfiguration) {
final File osgiConfigurationFile = new File(osgiConfigurationsDirectory, osgiConfiguration.getDestinationFilename());
writeOsgiConfigurationIntoFile(osgiConfiguration.getOsgiConfiguration(), osgiConfigurationFile);
}
private void writeOsgiConfigurationIntoFile(final String osgiConfiguration,
final File osgiConfigurationFile) {
try {
writeFileContent(osgiConfiguration, osgiConfigurationFile.getAbsolutePath());
} catch (IOException e) {
LOGGER.error("Cannot write OSGi configuration ({}) into profile.",
osgiConfigurationFile.getAbsolutePath());
throw new ProfileWriteException("Cannot write OSGi configuration profile: "
+ osgiConfigurationFile.getAbsolutePath(), e);
}
}
private void writeFileContent(final String fileContent,
final String absolutePathToFile) throws IOException {
Files.write(Paths.get(absolutePathToFile), fileContent.getBytes(PROFILE_CHARSET));
}
private void createCommandLineFile(final File newProfileDirectory,
final List templatedCommandLineOptionsAndArguments) {
try {
createCommandLineFileUnchecked(newProfileDirectory, templatedCommandLineOptionsAndArguments);
} catch (IOException e) {
final String theProfileFilePath = newProfileDirectory + File.separator +
ProfileStorageFormatConstants.PROFILE_COMMAND_LINE_FILE_NAME;
LOGGER.error("Cannot create command line profile file ({}). Internal or system error.",
theProfileFilePath);
throw new ProfileWriteException("Cannot create profile file: " + theProfileFilePath);
}
}
private void createCommandLineFileUnchecked(final File newProfileDirectory,
final List templatedCommandLineOptionsAndArguments)
throws IOException {
final File commandLineFile = new File(newProfileDirectory,
ProfileStorageFormatConstants.PROFILE_COMMAND_LINE_FILE_NAME);
final String commandLine = Joiner.on(COMMAND_OPTION_OR_ARGUMENT_SEPARATOR).join(
quoteStringsContainingSpaces(templatedCommandLineOptionsAndArguments));
writeFileContent(commandLine, commandLineFile.getAbsolutePath());
}
private Iterable quoteStringsContainingSpaces(List templatedCommandLineOptionsAndArguments) {
return transform(templatedCommandLineOptionsAndArguments,
new Function() {
@Nullable
@Override
public String apply(@Nullable String commandLineArgumentOrOption) {
return commandLineArgumentOrOption.contains(SPACE)
? (QUOTE_STRING + commandLineArgumentOrOption + QUOTE_STRING)
: commandLineArgumentOrOption;
}
});
}
private File createTagsDefinitionsDirectory(final File newProfileDirectory) {
final File tagsDefinitionsDirectory = new File(newProfileDirectory,
ProfileStorageFormatConstants.TAGS_DIRECTORY_NAME);
tagsDefinitionsDirectory.mkdir();
if (!tagsDefinitionsDirectory.exists() && !tagsDefinitionsDirectory.isDirectory()) {
LOGGER.error("Cannot create tags definitions profile directory ({}). Internal or system error.",
tagsDefinitionsDirectory.getAbsolutePath());
throw new ProfileWriteException(THE_DIRECTORY_HASN_T_BEEN_CREATED_BY_SYSTEM_EXCEPTION_MESSAGE);
}
return tagsDefinitionsDirectory;
}
private File createOsgiConfigurationsDirectory(final File newProfileDirectory) {
final File osgiConfigurationsDirectory = new File(newProfileDirectory,
ProfileStorageFormatConstants.OSGI_CONFIGURATIONS_DIRECTORY_NAME);
osgiConfigurationsDirectory.mkdir();
if (!osgiConfigurationsDirectory.exists() && !osgiConfigurationsDirectory.isDirectory()) {
LOGGER.error("Cannot create OSGi configurations profile directory ({}). Internal or system error.",
osgiConfigurationsDirectory.getAbsolutePath());
throw new ProfileWriteException(THE_DIRECTORY_HASN_T_BEEN_CREATED_BY_SYSTEM_EXCEPTION_MESSAGE);
}
return osgiConfigurationsDirectory;
}
private void createProfileDirectory(final File newProfileDirectory) {
newProfileDirectory.mkdirs();
if (!newProfileDirectory.exists() && !newProfileDirectory.isDirectory()) {
LOGGER.error("Cannot create profile directory ({}). Internal or system error.",
newProfileDirectory.getAbsolutePath());
throw new ProfileWriteException(THE_DIRECTORY_HASN_T_BEEN_CREATED_BY_SYSTEM_EXCEPTION_MESSAGE);
}
}
private void checkForExistingFile(final File newProfileDirectory) {
if (newProfileDirectory.exists() && newProfileDirectory.isFile()) {
LOGGER.error("Cannot create profile (directory) in a path that is a file: {}",
newProfileDirectory.getAbsolutePath());
throw new ProfileWriteException("Cannot create directory in place of existing file.");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy