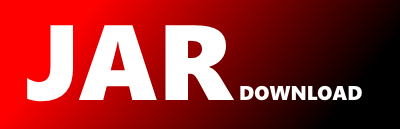
org.apache.sling.settings.impl.RunModes Maven / Gradle / Ivy
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2016 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package org.apache.sling.settings.impl;
import com.google.common.collect.ImmutableList;
import java.util.Collection;
import java.util.List;
import java.util.Objects;
import static java.util.Objects.requireNonNull;
public class RunModes {
private final Collection groups;
public RunModes(final Collection groups) {
this.groups = groups;
}
public static class Group {
private final List modes;
private final String selected;
public Group(final List modes) {
this(null, modes);
}
public Group(final List modes, final String selected) {
this(requireNonNull(selected), modes);
}
private Group(final String selected, final List modes) {
this.modes = requireNonNull(modes);
this.selected = selected;
}
public List getAvailableModes() {
return ImmutableList.copyOf(modes);
}
public String getSelectedMode() {
return selected;
}
@Override
public boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
final Group that = (Group) o;
return Objects.equals(modes, that.modes) &&
Objects.equals(selected, that.selected);
}
@Override
public int hashCode() {
return Objects.hash(modes, selected);
}
@Override
public String toString() {
return "Group{" +
"modes=" + modes +
", selected='" + selected + '\'' +
'}';
}
}
public Collection getGroups() {
return ImmutableList.copyOf(groups);
}
@Override
public String toString() {
return "RunModes{" +
"groups=" + groups +
'}';
}
@Override
public boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
final RunModes that = (RunModes) o;
return Objects.equals(groups, that.groups);
}
@Override
public int hashCode() {
return Objects.hash(groups);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy