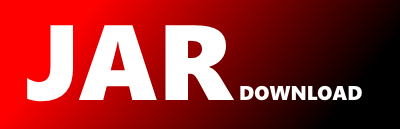
com.adobe.granite.crx2oak.cli.transformer.CommandLineOptionsTransformerEngine Maven / Gradle / Ivy
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2016 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.adobe.granite.crx2oak.cli.transformer;
import com.adobe.granite.crx2oak.cli.CmdLineOption;
import com.google.common.base.Predicate;
import com.google.common.collect.ImmutableList;
import joptsimple.OptionSet;
import joptsimple.OptionSpec;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import static com.adobe.granite.crx2oak.cli.CmdLineOption.withArgument;
import static com.adobe.granite.crx2oak.cli.CmdLineOption.withoutArgument;
import static com.google.common.base.Preconditions.checkState;
import static com.google.common.collect.Collections2.filter;
import static com.google.common.collect.ImmutableList.copyOf;
import static java.util.Collections.singletonList;
/**
* The universal command line option transformer engine transforms {@linkplain OptionSet} with the help of the
* event listener interface {@linkplain CommandLineEventTransformer} that is pluggable and might
* be implemented by a caller to achieve different results for command line options.
*/
public class CommandLineOptionsTransformerEngine {
private static final String STANDALONE_ARGUMENT_SPECIAL_OPTION_PLACEHOLDER = "[arguments]";
/**
* Transform options from the {@linkplain OptionSet} class into a not-null collection of
* {@linkplain CmdLineOption} objects using the provided {@linkplain CommandLineEventTransformer}
* reference object.
*
* @param optionSet the option set that will be transformed
* @param eventTransformer the event transformed to be used during transformation
* @return a not-null collection of output options that are transformed.
*/
Collection transformOptions(final OptionSet optionSet,
final CommandLineEventTransformer eventTransformer) {
final Collection outputOptions = new ArrayList();
outputOptions.addAll(getMandatoryOptions(eventTransformer));
outputOptions.addAll(getTransformedAndAdditionalOptions(optionSet, eventTransformer, outputOptions));
return outputOptions;
}
private Collection getTransformedAndAdditionalOptions(final OptionSet optionSet,
final CommandLineEventTransformer eventTransformer,
final Collection initialOptions) {
final Collection outputOptionsLocal = new ArrayList();
final Collection blacklistedOptions = new HashSet(getOnlyOptions(initialOptions));
final Collection transformedOptions = getTransformedCommandLineOptions(optionSet,
eventTransformer, blacklistedOptions);
blacklistedOptions.addAll(getOnlyOptions(transformedOptions));
outputOptionsLocal.addAll(transformedOptions);
outputOptionsLocal.addAll(getAdditionalOptions(eventTransformer, blacklistedOptions));
return outputOptionsLocal;
}
private Collection getTransformedCommandLineOptions(final OptionSet optionSet,
final CommandLineEventTransformer eventTransformer,
final Collection blacklistedOptions) {
final Collection newOptions = new ArrayList();
for (String optionName : getDetectedOptions(optionSet)) {
final Collection outputOptions = filterOptions(
transformOptionToOptions(eventTransformer, optionSet, optionName),
blacklistedOptions);
newOptions.addAll(outputOptions);
}
return newOptions;
}
private Collection filterOptions(final Collection cmdLineOptions,
final Collection blacklistedOptions) {
return filter(cmdLineOptions, new Predicate() {
@Override
public boolean apply(CmdLineOption option) {
return !blacklistedOptions.contains(option.getOption());
}
});
}
private Collection getDetectedOptions(OptionSet optionSet) {
final List> specs = optionSet.specs();
final int numberOfArguments = optionSet.nonOptionArguments().size();
final ImmutableList> onlyOptionSpecs = getSpecsOnlyForOptions(specs.iterator(), numberOfArguments);
final Collection detectedOptions = getDetectedOptions(getAllAcceptedOptions(onlyOptionSpecs),
optionSet);
return detectedOptions;
}
private Collection getDetectedOptions(Collection allOptions, OptionSet optionSet) {
final Collection detectedOptionsUnique = new HashSet();
final Collection detectedOptions = new ArrayList();
for (String optionName : allOptions) {
if (optionSet.has(optionName)
&& !detectedOptionsUnique.contains(optionName)) {
detectedOptions.add(optionName);
detectedOptionsUnique.add(optionName);
}
}
return detectedOptions;
}
private Collection getAllAcceptedOptions(ImmutableList> onlyOptionSpecs) {
Collection allOptions = new ArrayList();
Collection allOptionsUnique = new HashSet();
for (OptionSpec spec : onlyOptionSpecs) {
for (Object option : spec.options()) {
if (option instanceof String) {
String optionName = option.toString();
if (!allOptionsUnique.contains(optionName)) {
allOptions.add(optionName);
allOptionsUnique.add(optionName);
}
}
}
}
return allOptions;
}
private Collection transformOptionToOptions(CommandLineEventTransformer eventTransformer, OptionSet optionSet, String optionName) {
final List> values = optionSet.valuesOf(optionName);
final Collection cmdLineOptions =
(values.isEmpty()) ?
eventTransformer.transformOption(withoutArgument(optionName))
: getMultiValueOptions(optionName, values, eventTransformer);
checkState(cmdLineOptions != null);
return cmdLineOptions;
}
private Collection getAdditionalOptions(CommandLineEventTransformer eventTransformer, Collection alreadyAddedOptions) {
final Collection additionalOptions = eventTransformer.getAdditionalOptions();
checkState(additionalOptions != null, "Additional options cannot be null (but can be empty)");
final Collection filteredOptions = new ArrayList();
for (CmdLineOption compositeOption : additionalOptions) {
if (!alreadyAddedOptions.contains(compositeOption.getOption())) {
filteredOptions.add(compositeOption);
}
}
return filteredOptions;
}
private Collection getOnlyOptions(Collection compositeOptions) {
Collection options = new ArrayList();
for (CmdLineOption compositeOption : compositeOptions) {
options.add(compositeOption.getOption());
}
return options;
}
private Collection getMandatoryOptions(CommandLineEventTransformer eventTransformer) {
Collection compositeOptions = new ArrayList();
final Collection mandatoryOptions = eventTransformer.getMandatoryOptions();
checkState(mandatoryOptions != null, "Mandatory options needs to be not null (can be empty)");
for (CmdLineOption o : mandatoryOptions) {
compositeOptions.add(o);
}
return compositeOptions;
}
private ImmutableList> getSpecsOnlyForOptions(Iterator> specIterator, int numberOfArguments) {
ImmutableList> optionSpecs = copyOf(specIterator);
Collection> filteredOptionSpecs = new ArrayList>();
for (OptionSpec> optionSpec : optionSpecs) {
filteredOptionSpecs.addAll(getOptionsIfValid(optionSpec));
}
return copyOf(filteredOptionSpecs);
}
private Collection> getOptionsIfValid(OptionSpec> optionSpec) {
return isNotANonOptionArgument(optionSpec)
? Collections.>singleton(optionSpec)
: Collections.>emptyList();
}
private boolean isNotANonOptionArgument(OptionSpec optionSpec) {
return !optionSpec.options().equals(singletonList(STANDALONE_ARGUMENT_SPECIAL_OPTION_PLACEHOLDER));
}
private Collection getMultiValueOptions(String optionName, List> values, CommandLineEventTransformer eventTransformer) {
Collection list = new ArrayList();
for (Object value : values) {
final CmdLineOption inputOption = withArgument(optionName, value.toString());
final Collection outputOptions = eventTransformer.transformOption(inputOption);
checkState(outputOptions != null);
list.addAll(outputOptions);
}
return list;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy