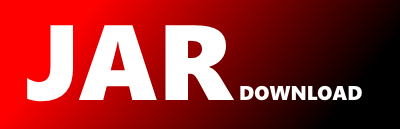
com.adobe.granite.crx2oak.engine.crx.CRX2MigrationFactory Maven / Gradle / Ivy
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2011 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.adobe.granite.crx2oak.engine.crx;
import com.adobe.granite.crx2oak.auth.UniquePrincipalNameHook;
import com.adobe.granite.crx2oak.ldap.LdapAuthorizableCommitHook;
import com.adobe.granite.crx2oak.ldap.LdapConfiguration;
import com.adobe.granite.crx2oak.sling.SlingFolderHook;
import com.google.common.io.Closer;
import org.apache.jackrabbit.core.RepositoryContext;
import org.apache.jackrabbit.core.fs.FileSystemException;
import org.apache.jackrabbit.oak.plugins.blob.datastore.DataStoreBlobStore;
import org.apache.jackrabbit.oak.spi.blob.BlobStore;
import org.apache.jackrabbit.oak.spi.commit.CommitHook;
import org.apache.jackrabbit.oak.spi.state.NodeStore;
import org.apache.jackrabbit.oak.upgrade.RepositoryUpgrade;
import org.apache.jackrabbit.oak.upgrade.cli.MigrationFactory;
import org.apache.jackrabbit.oak.upgrade.cli.parser.DatastoreArguments;
import org.apache.jackrabbit.oak.upgrade.cli.parser.MigrationCliArguments;
import org.apache.jackrabbit.oak.upgrade.cli.parser.MigrationOptions;
import org.apache.jackrabbit.oak.upgrade.cli.parser.StoreArguments;
import org.osgi.framework.InvalidSyntaxException;
import javax.jcr.RepositoryException;
import javax.naming.InvalidNameException;
import java.io.File;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import static com.adobe.granite.crx2oak.cli.CRX2OakOption.LDAP;
import static com.adobe.granite.crx2oak.cli.CRX2OakOption.LDAP_CONFIG;
public class CRX2MigrationFactory extends MigrationFactory {
private final CommitHook ldapHook;
public CRX2MigrationFactory(final MigrationCliArguments args,
final MigrationOptions opts,
final StoreArguments stores,
final DatastoreArguments dataStores,
final Closer closer) throws IOException {
super(opts, stores, dataStores, closer);
this.ldapHook = createLdapHook(args.hasOption(LDAP.option),
args.getOption(LDAP_CONFIG.option));
}
@Override
public RepositoryUpgrade createUpgrade() throws IOException, RepositoryException {
String[] paths = stores.getSrcPaths();
RepositoryContext src;
try {
src = new CRX2Factory(paths[0], paths[1]).create(closer);
} catch (FileSystemException e) {
throw new IOException(e);
}
BlobStore srcBlobStore = new DataStoreBlobStore(src.getDataStore());
NodeStore dstStore = createTarget(closer, srcBlobStore);
RepositoryUpgrade upgrade = createUpgrade(src, dstStore);
List hooks = new ArrayList<>(upgrade.getCustomCommitHooks());
if (ldapHook != null) {
hooks.add(ldapHook);
}
hooks.add(new SlingFolderHook());
hooks.add(new UniquePrincipalNameHook());
upgrade.setCustomCommitHooks(hooks);
return upgrade;
}
private LdapAuthorizableCommitHook createLdapHook(boolean ldap, String ldapConfig) throws IOException {
LdapAuthorizableCommitHook ldapHook = null;
if (ldapConfig != null) {
LdapConfiguration config;
try {
config = new LdapConfiguration(new File(ldapConfig));
} catch (InvalidNameException | InvalidSyntaxException e) {
throw new IOException(e);
}
ldapHook = new LdapAuthorizableCommitHook(config);
} else if (ldap) {
ldapHook = new LdapAuthorizableCommitHook();
}
return ldapHook;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy