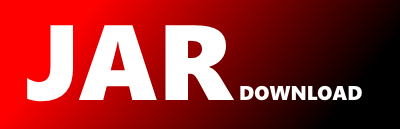
com.adobe.granite.crx2oak.engine.crx.CrxUpgradeEngine Maven / Gradle / Ivy
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2016 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.adobe.granite.crx2oak.engine.crx;
import com.adobe.granite.crx2oak.oak.OakConstants;
import com.google.common.collect.Iterators;
import com.google.common.io.Closer;
import org.apache.jackrabbit.oak.spi.lifecycle.CompositeInitializer;
import org.apache.jackrabbit.oak.spi.lifecycle.RepositoryInitializer;
import org.apache.jackrabbit.oak.upgrade.RepositoryUpgrade;
import org.apache.jackrabbit.oak.upgrade.cli.CliUtils;
import org.apache.jackrabbit.oak.upgrade.cli.MigrationFactory;
import org.apache.jackrabbit.oak.upgrade.cli.parser.CliArgumentException;
import org.apache.jackrabbit.oak.upgrade.cli.parser.DatastoreArguments;
import org.apache.jackrabbit.oak.upgrade.cli.parser.MigrationCliArguments;
import org.apache.jackrabbit.oak.upgrade.cli.parser.MigrationOptions;
import org.apache.jackrabbit.oak.upgrade.cli.parser.StoreArguments;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import javax.jcr.RepositoryException;
import java.io.File;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import java.util.ServiceLoader;
import java.util.regex.Pattern;
import static com.adobe.granite.crx2oak.cli.CRX2OakOption.DISABLE_INDEXES;
public class CrxUpgradeEngine {
private static final Logger log = LoggerFactory.getLogger(CrxUpgradeEngine.class);
public static void backupOldCrx2Files(String repositoryDirPath, boolean preserveDataStore) {
File repositoryDir = new File(repositoryDirPath);
File crx2 = new File(repositoryDir, "crx2");
log.info("Moving existing repository under {}", crx2.getAbsolutePath());
crx2.mkdir();
File datastore = new File(repositoryDir, "repository/datastore");
File tmpDatastore = new File(repositoryDir, "datastore");
if (preserveDataStore && datastore.isDirectory()) {
log.info("Preserving datastore under {}", datastore.toString());
datastore.renameTo(tmpDatastore);
}
Pattern pattern = Pattern.compile("crx2|" + OakConstants.SEGMENT_STORE_DIR + "|datastore");
for (File file : repositoryDir.listFiles()) {
String name = file.getName();
if (!pattern.matcher(name).matches()) {
file.renameTo(new File(crx2, name));
}
}
if (preserveDataStore && tmpDatastore.isDirectory()) {
new File(repositoryDir, "repository").mkdir();
tmpDatastore.renameTo(datastore);
}
}
private void upgradeFromCrx2(MigrationFactory factory, MigrationCliArguments args)
throws IOException, RepositoryException, CliArgumentException {
RepositoryUpgrade upgrade = factory.createUpgrade();
upgrade.copy(createComponentInitializer(args.getOptionList(DISABLE_INDEXES.option)));
}
private RepositoryInitializer createComponentInitializer(String[] disabledIndexes) {
ServiceLoader loader = ServiceLoader.load(RepositoryInitializer.class);
List initializers = new ArrayList();
initializers.add(new ConfigurableGraniteContent(disabledIndexes));
Iterators.addAll(initializers, loader.iterator());
return new CompositeInitializer(initializers);
}
public void migrateCrx2(final MigrationCliArguments args,
final MigrationOptions opts,
final StoreArguments stores,
final DatastoreArguments dataStores) throws IOException {
Closer closer = Closer.create();
CliUtils.handleSigInt(closer);
MigrationFactory migrationFactory = new CRX2MigrationFactory(args, opts, stores, dataStores,
closer);
try {
upgradeFromCrx2(migrationFactory, args);
} catch (Throwable t) {
throw closer.rethrow(t);
} finally {
closer.close();
}
if (stores.isInPlaceUpgrade()) {
backupOldCrx2Files(stores.getSrcPaths()[0], isPreserveDataStore(dataStores));
}
}
private boolean isPreserveDataStore(DatastoreArguments datastoreArguments) {
return datastoreArguments.getBlobMigrationCase() == DatastoreArguments.BlobMigrationCase.COPY_REFERENCES;
}
public void migrateCrx2() {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy