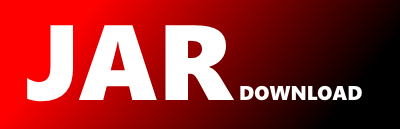
com.adobe.granite.crx2oak.util.CliUtils Maven / Gradle / Ivy
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2011 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.adobe.granite.crx2oak.util;
import joptsimple.OptionSet;
import org.apache.jackrabbit.oak.upgrade.cli.parser.MigrationCliArguments;
import javax.annotation.Nonnull;
import java.io.IOException;
import java.io.InputStream;
import java.util.ArrayList;
import java.util.List;
import java.util.Properties;
import static com.google.common.base.Preconditions.checkNotNull;
import static java.lang.System.exit;
import static org.apache.commons.lang3.StringUtils.isNotEmpty;
/**
* The command line related utilities methods.
* Please note that this class cannot be instantiated to the object.
*/
public final class CliUtils {
/**
* The application Maven group ID
*/
public static final String APP_GROUP_ID = "com.adobe.granite";
/**
* The application Maven artifact ID
*/
public static final String APP_ARTIFACT_ID = "crx2oak";
/**
* The default version string if the version cannot be determined
*/
public static final String UNKNOWN_VERSION = "unknown version";
/**
* The name of the property that will be used for checking the Maven version
*/
public static final String VERSION_PROPERTY_NAME = "version";
private CliUtils() {
}
/**
* Returns the version of crx2oak bundle from the classpath based on the Maven metadata pom.properties file
* that should be present in the classpath (most probably in correctly built JAR file at runtime).
*
* @return the version number if it can be determined or {@linkplain #UNKNOWN_VERSION} if the version is not known
*/
@Nonnull
public static String getVersion() {
InputStream stream = MigrationCliArguments.class
.getResourceAsStream("/META-INF/maven/" + APP_GROUP_ID + "/" + APP_ARTIFACT_ID + "/pom.properties");
if (stream != null) {
try {
try {
Properties properties = new Properties();
properties.load(stream);
final String versionValue = properties.getProperty(VERSION_PROPERTY_NAME);
return isNotEmpty(versionValue) ? versionValue : UNKNOWN_VERSION;
} finally {
stream.close();
}
} catch (IOException e) {
// ignore
}
}
return UNKNOWN_VERSION;
}
/**
* Exit JVM only and only if the exit code is not equal to 0.
*
* @param exitCode the exit code to be checked.
*/
public static void optionallyExitJVMIfExitCodeIsError(final int exitCode) {
if (exitCode != 0) {
exit(exitCode);
}
}
/**
* Get a list of string values that were given as arguments for the specified option (its name).
*
* @param option the option name which arguments will be retrieved and converted into strings
* @param parsedOptions the not-null already parsed set of options
* @return the not null (optionally empty) list of string values
*/
public static List getOptionValuesAsStrings(@Nonnull final String option,
@Nonnull final OptionSet parsedOptions) {
checkNotNull(option);
checkNotNull(parsedOptions);
final List> objects = parsedOptions.valuesOf(option);
List values = new ArrayList();
if (objects != null) {
for (Object arg : objects) {
values.add(arg.toString());
}
}
return values;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy