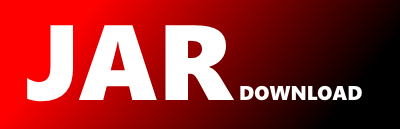
org.apache.sling.settings.impl.SlingOptionsFileReader Maven / Gradle / Ivy
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2016 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package org.apache.sling.settings.impl;
import com.google.common.base.Optional;
import org.apache.sling.settings.impl.RunModes.Group;
import org.apache.sling.settings.impl.SlingSettingsServiceImpl.Options;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.ObjectInputStream;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
/**
* Reads a sling.options.file that is expected ot be a serialized java object.
*/
public class SlingOptionsFileReader {
private static final Logger LOGGER = LoggerFactory.getLogger(SlingOptionsFileReader.class);
/**
* Read the sling.options.file
* @param file the file that is sling.options.file
* @return the result of read operation on that file
*/
public SlingOptionsReadResult readOptions(File file) {
List> optionsList = null;
if ( file.exists() ) {
FileInputStream fis = null;
ObjectInputStream ois = null;
try {
fis = new FileInputStream(file);
ois = new ObjectInputStream(fis);
final Object object = ois.readObject();
optionsList = (object instanceof List) ? (List>) object : null;
} catch (final IOException ioe) {
LOGGER.error("Unable to read from options data file.", ioe);
return new SlingOptionsReadResult();
} catch (final ClassNotFoundException cnfe) {
LOGGER.error("Unable to read from options data file.", cnfe);
return new SlingOptionsReadResult();
} finally {
if ( ois != null ) {
try { ois.close(); } catch ( final IOException ignore) {}
}
if ( fis != null ) {
try { fis.close(); } catch ( final IOException ignore) {}
}
}
}
final Optional> options = convertToOptionsList(optionsList);
return options.isPresent()
? new SlingOptionsReadResult(getSlingRunModes(options.get()))
: new SlingOptionsReadResult();
}
private RunModes getSlingRunModes(final List list) {
Collection groups = new ArrayList<>();
for (final Options options : list) {
groups.add(getRunModeGroup(options));
}
return new RunModes(groups);
}
private Group getRunModeGroup(final Options options) {
return options.selected == null
? new Group(Arrays.asList(options.modes))
: new Group(Arrays.asList(options.modes), options.selected);
}
private Optional> convertToOptionsList(final List> aList) {
return aList != null ? convertToOptionsListNotNull(aList) : Optional.>absent();
}
private Optional> convertToOptionsListNotNull(final List> aList) {
final List optionsList = new ArrayList<>();
for (Object object : aList) {
if (object instanceof Options) {
optionsList.add((Options) object);
} else {
return Optional.absent();
}
}
return Optional.of(optionsList);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy