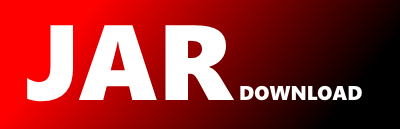
com.adobe.granite.crx2oak.profile.model.template.RawProfileTemplate Maven / Gradle / Ivy
The newest version!
/*************************************************************************
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2016 Adobe
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe
* and its suppliers and are protected by all applicable intellectual
* property laws, including trade secret and copyright laws.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe.
**************************************************************************/
package com.adobe.granite.crx2oak.profile.model.template;
import com.adobe.granite.crx2oak.profile.model.osgi.OsgiConfiguration;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import static com.google.common.base.Preconditions.checkNotNull;
/**
* The model of raw profile template (configurable by tags). A synonym of serialized disk profile.
* For both command line arguments and option arguments and OSGi configuration placeholder are
*/
public class RawProfileTemplate {
Map tagsWithValues;
List templatedCommandLineOptionsAndArguments;
Collection osgiConfigurationsTemplates;
public RawProfileTemplate(List templatedCommandLineOptionsAndArguments,
Collection osgiConfigurationsTemplates,
Map tagsWithValues) {
this.tagsWithValues = new HashMap(checkNotNull(tagsWithValues));
this.templatedCommandLineOptionsAndArguments = new ArrayList(
checkNotNull(templatedCommandLineOptionsAndArguments));
this.osgiConfigurationsTemplates = new HashSet(checkNotNull(osgiConfigurationsTemplates));
}
/**
* Create a raw profile template that doesn't contain any template tags
*
* @param templatedCommandLineOptionsAndArguments a list of options and argument that might contain template tags
* @param osgiConfigurationsTemplates the OSGi configuration templates that might contain template tags
*/
public RawProfileTemplate(List templatedCommandLineOptionsAndArguments,
Collection osgiConfigurationsTemplates) {
this(templatedCommandLineOptionsAndArguments, osgiConfigurationsTemplates, Collections.emptyMap());
}
/**
* Get the currently defined tags with their counterpart values that can be substituted.
*
* @return a non-null mappings of placeholder and its effective value
*/
public Map getTagsWithValues() {
return tagsWithValues;
}
/**
* The raw options and arguments mixed together in form of ordered string tokens. Arguments (both for options
* or standalone ones) can use tags at this level. To be able to say more
* what are options, what are arguments, the option parser needs to process this list.
*
* @return a non-null (optionally empty) list of string tokens
*/
public List getTemplatedCommandLineOptionsAndArguments() {
return templatedCommandLineOptionsAndArguments;
}
/**
* Get the list of OSGi configuration templates that are optionally annotated with tags.
*
* @return a non-null (optionally empty) list of non-null OSGi configuration objects
*/
public Collection getOsgiConfigurationsTemplates() {
return osgiConfigurationsTemplates;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
RawProfileTemplate that = (RawProfileTemplate) o;
if (!tagsWithValues.equals(that.tagsWithValues)) return false;
if (!templatedCommandLineOptionsAndArguments.equals(that.templatedCommandLineOptionsAndArguments)) return false;
return osgiConfigurationsTemplates.equals(that.osgiConfigurationsTemplates);
}
@Override
public int hashCode() {
int result = tagsWithValues.hashCode();
result = 31 * result + templatedCommandLineOptionsAndArguments.hashCode();
result = 31 * result + osgiConfigurationsTemplates.hashCode();
return result;
}
@Override
public String toString() {
return "RawProfileTemplate{" +
"tagsWithValues=" + tagsWithValues +
", templatedCommandLineOptionsAndArguments=" + templatedCommandLineOptionsAndArguments +
", osgiConfigurationsTemplates=" + osgiConfigurationsTemplates +
'}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy