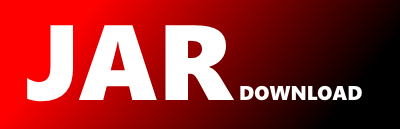
com.adobe.granite.crx2oak.util.FilesystemUtils Maven / Gradle / Ivy
The newest version!
/*************************************************************************
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2016 Adobe
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe
* and its suppliers and are protected by all applicable intellectual
* property laws, including trade secret and copyright laws.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe.
**************************************************************************/
package com.adobe.granite.crx2oak.util;
import com.google.common.base.Joiner;
import com.google.common.base.Splitter;
import org.apache.commons.codec.digest.DigestUtils;
import org.apache.commons.io.FileUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import javax.annotation.Nonnull;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.text.SimpleDateFormat;
import java.util.Date;
/**
* Stateless utility methods for filesystem.
*/
public final class FilesystemUtils {
private static final Logger LOGGER = LoggerFactory.getLogger(FilesystemUtils.class);
private FilesystemUtils() {
}
/**
* Return the timestamp suffix in human and filesystem friendly format (without spaces, separators etc) that
* might be used to create time dependent directories or files.
*
* @return the timestamp string without whitespaces.
*/
@Nonnull
public static String getCurrentTimestampFilenameSuffix() {
final SimpleDateFormat sdf = new SimpleDateFormat("yyyyMMdd-HHmmss");
return sdf.format(new Date());
}
/**
* Rewrite any line endings in input string (multi-line string) to be
* platform dependent based on the current system setting.
*
* @param fileContent the string that might contain multiple line definitions
* @return a properly formatted multi line string with new line endings compatible
* with current platform
*/
@Nonnull
public static String rewriteFileLines(@Nonnull final String fileContent) {
return Joiner.on(System.lineSeparator()).join(Splitter.onPattern("\r?\n").split(fileContent));
}
/**
* Copy the file if exists in the same folder adding a backup suffix with the current timestamp suffix.
* Please note that this method won't work with directories.
*
* @param file the file to backup
* @param backupSuffix the backup suffix
* @throws UncheckedIOException in case the rename is failing
*/
public static void backup(final File file, final String backupSuffix) throws UncheckedIOException {
try {
if (file.exists()) {
final String backupFileName = file.getAbsolutePath() + backupSuffix + getCurrentTimestampFilenameSuffix() + ".bak";
//noinspection ResultOfMethodCallIgnored
FileUtils.copyFile(file, new File(backupFileName));
}
} catch (IOException e) {
throw new UncheckedIOException(e);
}
}
/**
* Checks if the file already contains the same content (including line endings).
*
* @param file the file which content will be checked
* @param newContentOfFile the new content
* @return true if the checksum of the new content and existing content of current file is the same
*/
public static boolean areTheSame(final File file, final String newContentOfFile) {
return file.exists() && areTheSameByCheckingChecksum(file, newContentOfFile);
}
private static boolean areTheSameByCheckingChecksum(final File file, final String newContentOfFile) {
try {
final String existingContentChecksum = DigestUtils.sha512Hex(new FileInputStream(file));
final String newContentChecksum = DigestUtils.sha512Hex(newContentOfFile);
final boolean equals = newContentChecksum.equals(existingContentChecksum);
LOGGER.debug("The file {} with {} checksum and new content with {} checksum: are{} the same",
file.getAbsolutePath(), existingContentChecksum, newContentChecksum, equals ? "" : "n't");
return equals;
} catch (IOException e) {
LOGGER.warn("Cannot compare existing file content with new content. Assuming that files are different.", e);
return false;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy