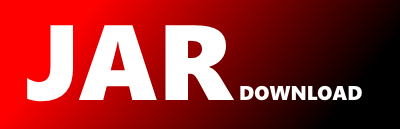
com.adobe.granite.crx2oak.util.filesystem.DirectoryActivator Maven / Gradle / Ivy
The newest version!
/*************************************************************************
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2016 Adobe
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe
* and its suppliers and are protected by all applicable intellectual
* property laws, including trade secret and copyright laws.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe.
**************************************************************************/
package com.adobe.granite.crx2oak.util.filesystem;
import com.adobe.granite.crx2oak.model.Topics;
import com.adobe.granite.crx2oak.pipeline.InputAggregatingComponent;
import com.adobe.granite.crx2oak.pipeline.PipeData;
import com.google.common.base.Optional;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import javax.annotation.Nonnull;
import java.io.File;
import java.io.IOException;
import static com.adobe.granite.crx2oak.util.FilesystemUtils.getCurrentTimestampFilenameSuffix;
import static com.google.common.base.Preconditions.checkNotNull;
import static java.lang.String.format;
/**
* The processor that activates the candidate directory (if exist) into a blessed directory (that might
* exist or not). If blessed directory exists it will be deactivated as the old backup directory.
*
* FIXME change to support move operation across filesystems instead of just rename
*/
public class DirectoryActivator extends InputAggregatingComponent {
private static final String LOGGER_PLACEHOLDER = "{}";
private static final String STRING_FORMAT_PLACEHOLDER = "%s";
private final Logger log = LoggerFactory.getLogger(DirectoryActivator.class);
private final String ERROR_MSG = "Could not move directory: {} into: {}. You need to manually swap directories";
private final File blessedDirectory;
private final File candidateDirectory;
private final File backupDirectory;
/**
* Create a processor with the specific directories: candidate one and blessed one and with backup suffix
* that will be used optionally if blessed directory needs to be first archived.
*
* @param blessedDirectory the blessed directory (might exist or not)
* @param candidateDirectory the candidate directory (that should exist before using {@linkplain #process(com.adobe.granite.crx2oak.pipeline.PipeData)})
* @param partialBackupSuffix the mandatory backup suffix that will be used only in some cases
* @throws IOException when there is a problem with calculating canonical paths from the given directories
*/
public DirectoryActivator(@Nonnull final File blessedDirectory,
@Nonnull final File candidateDirectory,
@Nonnull final String partialBackupSuffix) throws IOException {
checkNotNull(blessedDirectory);
checkNotNull(candidateDirectory);
checkNotNull(partialBackupSuffix);
this.blessedDirectory = blessedDirectory.getCanonicalFile();
this.candidateDirectory = candidateDirectory.getCanonicalFile();
final String fullSuffix = "-" + partialBackupSuffix + "backup-" + getCurrentTimestampFilenameSuffix();
backupDirectory = new File(this.blessedDirectory.getAbsolutePath() + fullSuffix);
}
@Override
protected PipeData preprocess(final PipeData input) {
final Optional optWarning = blessedDirectory.exists()
? deactivatePreviouslyBlessedAndBlessACandidate() : blessACandidateDirectory();
return optWarning.isPresent()
? PipeData.join(input, Topics.WARNINGS, optWarning.get()).toPipe()
: PipeData.EMPTY;
}
private Optional deactivatePreviouslyBlessedAndBlessACandidate() {
return blessedDirectory.renameTo(backupDirectory)
? blessACandidateDirectory() : announceMoveError(this.blessedDirectory, this.backupDirectory);
}
private Optional blessACandidateDirectory() {
return !candidateDirectory.renameTo(blessedDirectory)
? announceMoveError(candidateDirectory, blessedDirectory) : Optional.absent();
}
private Optional announceMoveError(final File fromDir, final File toDir) {
log.error(ERROR_MSG, fromDir, toDir);
return Optional.of(format(getStringFormatMessage(ERROR_MSG), fromDir, toDir));
}
private String getStringFormatMessage(final String loggerErrorMessage) {
return loggerErrorMessage.replace(LOGGER_PLACEHOLDER, STRING_FORMAT_PLACEHOLDER);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy