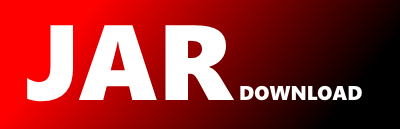
com.adobe.target.edge.client.http.DefaultTargetHttpClient Maven / Gradle / Ivy
/*
* Copyright 2021 Adobe. All rights reserved.
* This file is licensed to you under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License. You may obtain a copy
* of the License at http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under
* the License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR REPRESENTATIONS
* OF ANY KIND, either express or implied. See the License for the specific language
* governing permissions and limitations under the License.
*/
package com.adobe.target.edge.client.http;
import static com.adobe.target.edge.client.ondevice.OnDeviceDecisioningService.TIMING_EXECUTE_REQUEST;
import com.adobe.target.edge.client.ClientConfig;
import com.adobe.target.edge.client.ClientProxyConfig;
import com.adobe.target.edge.client.utils.MathUtils;
import com.adobe.target.edge.client.utils.StringUtils;
import com.adobe.target.edge.client.utils.TimingTool;
import java.io.UnsupportedEncodingException;
import java.time.Duration;
import java.util.Map;
import java.util.concurrent.CompletableFuture;
import java.util.function.Function;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import kong.unirest.Config;
import kong.unirest.HttpResponse;
import kong.unirest.ObjectMapper;
import kong.unirest.RawResponse;
import kong.unirest.Unirest;
import kong.unirest.UnirestException;
import kong.unirest.UnirestInstance;
import kong.unirest.apache.ApacheClient;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class DefaultTargetHttpClient implements TargetHttpClient {
private static final Logger logger = LoggerFactory.getLogger(DefaultTargetHttpClient.class);
private static final Pattern CHARSET_PATTERN =
Pattern.compile("(?i)\\bcharset=\\s*\"?([^\\s;\"]*)");
private UnirestInstance unirestInstance = Unirest.spawnInstance();
private ObjectMapper serializer = new JacksonObjectMapper();
private static final int DECIMAL_PLACES = 2;
public DefaultTargetHttpClient(ClientConfig clientConfig) {
Config config = unirestInstance.config();
config
.socketTimeout(clientConfig.getSocketTimeout())
.connectTimeout(clientConfig.getConnectTimeout())
.connectionTTL(Duration.ofMillis(clientConfig.getConnectionTtlMs()))
.concurrency(clientConfig.getMaxConnectionsTotal(), clientConfig.getMaxConnectionsPerHost())
.automaticRetries(clientConfig.isEnabledRetries())
.enableCookieManagement(false)
.setObjectMapper(getObjectMapper())
.setDefaultHeader("Accept", "application/json");
if (clientConfig.isLogRequestStatus()) {
config.instrumentWith(new TargetMetrics(new LoggingMetricConsumer()));
}
if (clientConfig.getRequestInterceptor() != null) {
config.addInterceptor(clientConfig.getRequestInterceptor());
}
if (clientConfig.isProxyEnabled()) {
ClientProxyConfig proxyConfig = clientConfig.getProxyConfig();
if (proxyConfig.isAuthProxy()) {
config.proxy(
proxyConfig.getHost(),
proxyConfig.getPort(),
proxyConfig.getUsername(),
proxyConfig.getPassword());
} else {
config.proxy(proxyConfig.getHost(), proxyConfig.getPort());
}
}
if (clientConfig.getHttpClient() != null) {
config.httpClient(new ApacheClient(clientConfig.getHttpClient(), config));
} else {
config.httpClient(ApacheClientFactory.initializeSyncClient(clientConfig, config));
}
config.asyncClient(ApacheClientFactory.initializeAsyncClient(clientConfig, config));
}
@Override
public void addDefaultHeader(String key, String value) {
unirestInstance.config().setDefaultHeader(key, value);
}
@Override
public ResponseWrapper execute(
Map queryParams, String url, T request, Class responseClass) {
ResponseWrapper responseWrapper = new ResponseWrapper<>();
HttpResponse
© 2015 - 2024 Weber Informatics LLC | Privacy Policy