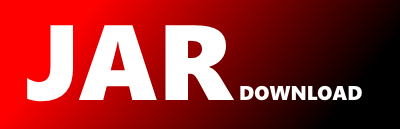
com.aegisql.util.function.ExceptionBlock Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ftry Show documentation
Show all versions of ftry Show documentation
Functional wrapper for try-catch-finally
/*
*Copyright (c) 2015, AEGIS DATA SOLUTIONS, All rights reserved.
*/
package com.aegisql.util.function;
import java.io.PrintStream;
import java.util.Objects;
import java.util.function.Function;
import org.slf4j.Logger;
// TODO: Auto-generated Javadoc
/**
* The Interface ExceptionBlock.
* @author Mikhail Teplitskiy
*
* @param the generic type
*/
@FunctionalInterface
public interface ExceptionBlock {
/**
* Accept.
*
* @param error the error
* @throws Throwable the throwable
*/
public void accept(T error) throws Throwable;
/**
* Identity.
*
* @param it the it
* @return the exception block
*/
public default ExceptionBlock identity(ExceptionBlock it) {
Objects.requireNonNull(it);
return it;
}
/**
* And then.
*
* @param after the after
* @return the exception block
*/
public default ExceptionBlock andThen(ExceptionBlock after) {
Objects.requireNonNull(after);
return (T t) -> {
this.accept(t);
after.accept(t);
};
}
/**
* Compose.
*
* @param after the after
* @return the exception block
*/
public default ExceptionBlock compose(ExceptionBlock after) {
Objects.requireNonNull(after);
return (T t) -> {
after.accept(t);
this.accept(t);
};
}
/**
* And die.
*
* @return the exception block
*/
public default ExceptionBlock andDie() {
return (T t) -> {
this.accept(t);
throw t;
};
}
/**
* And die as.
*
* @param wrapper the wrapper
* @param errMessage the err message
* @return the exception block
*/
public default ExceptionBlock andDieAs(Class extends Throwable> wrapper, String errMessage) {
Function f = ExceptionHandler.wrapCommentedThrowable().apply((Class) wrapper).apply(errMessage);
return (T t) -> {
this.accept(t);
Throwable wrapped = f.apply(t);
throw wrapped;
};
}
/**
* Prints the stack trace.
*
* @return the exception block
*/
public default ExceptionBlock printStackTrace() {
return andThen( t -> t.printStackTrace() );
}
/**
* Prints the stack trace.
*
* @param pStr the str
* @return the exception block
*/
public default ExceptionBlock printStackTrace(PrintStream pStr) {
return andThen( t -> t.printStackTrace(pStr) );
}
/**
* Prints the error message.
*
* @return the exception block
*/
public default ExceptionBlock printErrorMessage() {
return andThen( t -> System.out.println(t.getMessage()) );
}
/**
* Log debug stack trace.
*
* @param log the SLF4J logger
* @param preffix the preffix of the log message
* @param params - variables supported by the SLF4J
* @return the exception block
*/
public default ExceptionBlock logError(Logger log, String preffix, Object... params) {
return (T t) -> {
if(log.isErrorEnabled()) {
log.error(preffix + ExceptionHandler.toString(t), params);
}
this.accept(t);
};
}
/**
* Log warn stack trace.
*
* @param log the SLF4J logger
* @param preffix the preffix of the log message
* @param params - variables supported by the SLF4J
* @return the exception block
*/
public default ExceptionBlock logWarn(Logger log, String preffix, Object... params) {
return (T t) -> {
if(log.isWarnEnabled()) {
log.warn(preffix + ExceptionHandler.toString(t), params);
}
this.accept(t);
};
}
/**
* Log info stack trace.
*
* @param log the SLF4J logger
* @param preffix the preffix of the log message
* @param params - variables supported by the SLF4J
* @return the exception block
*/
public default ExceptionBlock logInfo(Logger log, String preffix, Object... params) {
return (T t) -> {
if(log.isInfoEnabled()) {
log.info(preffix + ExceptionHandler.toString(t), params);
}
this.accept(t);
};
}
/**
* Log debug stack trace.
*
* @param log the SLF4J logger
* @param preffix the preffix of the log message
* @param params - variables supported by the SLF4J
* @return the exception block
*/
public default ExceptionBlock logDebug(Logger log, String preffix, Object... params) {
return (T t) -> {
if(log.isDebugEnabled()) {
log.debug(preffix + ExceptionHandler.toString(t), params);
}
this.accept(t);
};
}
/**
* Log trace stack trace.
*
* @param log the SLF4J logger
* @param preffix the preffix of the log message
* @param params - variables supported by the SLF4J
* @return the exception block
*/
public default ExceptionBlock logTrace(Logger log, String preffix, Object... params) {
return (T t) -> {
if(log.isTraceEnabled()) {
log.trace(preffix + ExceptionHandler.toString(t), params);
}
this.accept(t);
};
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy