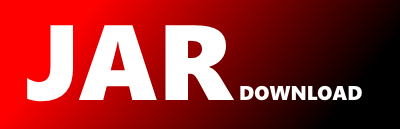
com.aegisql.util.function.ThrowingFunction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ftry Show documentation
Show all versions of ftry Show documentation
Functional wrapper for try-catch-finally
/*
*Copyright (c) 2015, AEGIS DATA SOLUTIONS, All rights reserved.
*/
package com.aegisql.util.function;
import java.util.function.Function;
import java.util.function.Supplier;
// TODO: Auto-generated Javadoc
/**
* The Interface ThrowingFunction.
* @author Mikhail Teplitskiy
*
* @param the generic type
* @param the generic type
* @param the element type
*/
@FunctionalInterface
public interface ThrowingFunction {
/**
* Applies this function to the given argument.
*
* @param t the function argument
* @return the function result
* @throws E the e
*/
public R apply(T t) throws E;
/**
* Wrap.
*
* @return the function
*/
public default Function > wrap() {
return t->{
try {
return new Result(this.apply(t));
} catch (Throwable e) {
return new Result(e);
}
};
}
/**
* Wrap exception.
*
* @return the throwing function
*/
public default ThrowingFunction wrapException() {
return this.wrapException(RuntimeException.class);
}
/**
* Wrap exception.
*
* @param message the message
* @return the throwing function
*/
public default ThrowingFunction wrapException(String message) {
return this.wrapException(RuntimeException.class,message);
}
/**
* Wrap exception.
*
* @param message the message
* @return the throwing function
*/
public default ThrowingFunction wrapException(Supplier message) {
return this.wrapException(RuntimeException.class,message);
}
/**
* Wrap exception.
*
* @param the generic type
* @param eClass the e class
* @return the throwing function
*/
public default ThrowingFunction wrapException(Class eClass) {
Function ef = ExceptionHandler.wrapThrowable().apply(eClass);
ThrowingFunction THIS = this;
return t->{
try {
return THIS.apply( t );
} catch(Throwable e) {
if(eClass.isAssignableFrom(e.getClass()))
throw (E1)e;
else
throw ef.apply(e);
}
};
}
/**
* Wrap exception.
*
* @param the generic type
* @param eClass the e class
* @param message the message
* @return the throwing function
*/
public default ThrowingFunction wrapException(Class eClass, String message) {
Function ef = ExceptionHandler.wrapCommentedThrowable().apply(eClass).apply(message);
return t->{
try {
return this.apply(t);
} catch(Throwable e) {
if(eClass.isAssignableFrom(e.getClass()))
throw (E1)e;
else
throw ef.apply(e);
}
};
}
/**
* Wrap exception.
*
* @param the generic type
* @param eClass the e class
* @param message the message
* @return the throwing function
*/
public default ThrowingFunction wrapException(Class eClass, Supplier message) {
Function ef = ExceptionHandler.wrapCommentedThrowable().apply(eClass).apply(message.get());
return t->{
try {
return this.apply(t);
} catch(Throwable e) {
if(eClass.isAssignableFrom(e.getClass()))
throw (E1)e;
else
throw ef.apply(e);
}
};
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy