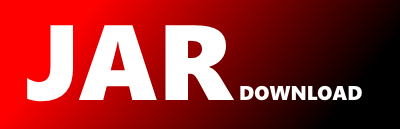
com.aeontronix.anypointsdk.accessmanagement.ConnectedAppData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of anypoint-sdk Show documentation
Show all versions of anypoint-sdk Show documentation
Anypoint Software Development Toolkit
/*
* Copyright (c) 2023. Aeontronix Inc
*/
package com.aeontronix.anypointsdk.accessmanagement;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.List;
public class ConnectedAppData {
@JsonProperty("audience")
private String audience;
@JsonProperty("client_uri")
private String clientUri;
@JsonProperty("grant_types")
private List grantTypes;
@JsonProperty("owner_user_id")
private String ownerUserId;
@JsonProperty("redirect_uris")
private List redirectUris;
@JsonProperty("cert_expiry")
private Object certExpiry;
@JsonProperty("client_id")
private String clientId;
@JsonProperty("enabled")
private boolean enabled;
@JsonProperty("public_keys")
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy