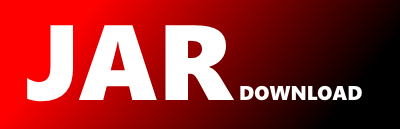
com.aeontronix.anypointsdk.amc.AMCClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of anypoint-sdk Show documentation
Show all versions of anypoint-sdk Show documentation
Anypoint Software Development Toolkit
/*
* Copyright (c) 2023. Aeontronix Inc
*/
package com.aeontronix.anypointsdk.amc;
import com.aeontronix.anypointsdk.AnypointClient;
import com.aeontronix.anypointsdk.amc.application.ApplicationIdentifier;
import com.aeontronix.anypointsdk.amc.application.ApplicationSource;
import com.aeontronix.anypointsdk.amc.application.FileApplicationSource;
import com.aeontronix.anypointsdk.amc.application.deployment.ApplicationDeployment;
import com.aeontronix.anypointsdk.amc.application.deployment.ApplicationDeploymentData;
import com.aeontronix.commons.exception.UnexpectedException;
import com.aeontronix.restclient.RESTClientHost;
import com.aeontronix.restclient.RESTClientHostPathBuilder;
import com.aeontronix.restclient.RESTException;
import com.aeontronix.restclient.json.JsonConvertionException;
import org.slf4j.Logger;
import java.io.IOException;
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
import java.util.*;
import static org.slf4j.LoggerFactory.getLogger;
public class AMCClient {
private static final Logger logger = getLogger(AMCClient.class);
private RESTClientHost restClient;
private AnypointClient anypointClient;
public AMCClient(AnypointClient anypointClient) {
this.restClient = anypointClient.getAnypointRestClient();
this.anypointClient = anypointClient;
}
public List listDeployments(String orgId, String envId) throws RESTException {
return deploymentPath(restClient.get(), orgId, envId).build()
.executeAndConvertToObject(AMCApplicationListResponse.class).getItems();
}
public Optional findDeploymentByName(String orgId, String envId, String name) throws RESTException {
return listDeployments(orgId, envId).stream().filter(a -> a.getName().equals(name)).findFirst();
}
public ApplicationDeployment findDeploymentById(String orgId, String envId, String appId) throws RESTException {
return new ApplicationDeployment(deploymentPath(restClient.get(), orgId, envId).path(appId, true).build()
.executeAndConvertToObject(ApplicationDeploymentData.class));
}
public AMCDeploymentResponse deployApplication(AMCAppDeploymentParameters params,
String appName, String orgId, String envId, String target,
ApplicationSource applicationSource,
Map properties, Set securePropertiesKeys,
String buildNumber) throws RESTException, IOException {
try {
ApplicationIdentifier appId = applicationSource.getApplicationIdentifier();
if (params.getAssetOrgId() != null) {
appId.setGroupId(params.getAssetOrgId());
}
if (params.getAssetArtifactId() != null) {
appId.setArtifactId(params.getAssetArtifactId());
}
if (params.getAssetVersion() != null) {
appId.setVersion(params.getAssetVersion());
}
if (appId.getVersion().toLowerCase().endsWith("-snapshot")) {
appId.setVersion(appId.getVersion() + "-" + (buildNumber != null ? buildNumber : DateTimeFormatter.ofPattern("yyyyMMddHHmmssSS").format(LocalDateTime.now())));
}
if (applicationSource instanceof FileApplicationSource) {
logger.info("Publishing archive to exchange: " + appId);
anypointClient.getExchangeClient().createAsset(orgId, appId.getArtifactId(), appId.getVersion(), appName)
.file("jar", "mule-application", null, applicationSource.getFilename(),
applicationSource.getFile())
.execute().close();
logger.info("Finished publishing archive to exchange: " + appId);
}
if (params.getRuntimeVersion() == null) {
params.setRuntimeVersion(anypointClient.getCloudhubClient().findDefaultCHMuleVersion().getVersion());
}
Optional existing = findDeploymentByName(orgId, envId, appName);
RESTClientHostPathBuilder method;
HashMap insecureProperties = new HashMap<>();
HashMap secureProperties = new HashMap<>();
if (existing.isPresent()) {
String deploymentId = existing.get().getId();
ApplicationDeployment existingDeployment = findDeploymentById(orgId, envId, deploymentId);
// copy properties
insecureProperties.putAll(existingDeployment.getData().getNonSecureProperties());
secureProperties.putAll(existingDeployment.getData().getSecureProperties());
// merge logging
Map existingLogLevels = existingDeployment.getLoggingLevels();
Map newLogLevels = params.getLogLevels(true);
for (Map.Entry e : existingLogLevels.entrySet()) {
newLogLevels.computeIfAbsent(e.getKey(), k -> e.getValue());
}
params.setLogLevels(newLogLevels);
method = deploymentPath(restClient.patch(), orgId, envId).path(deploymentId, true);
} else {
method = deploymentPath(restClient.post(), orgId, envId);
}
Set existingInsecureKeys = new HashSet<>(insecureProperties.keySet());
// If there are any insecure keys in existing API that are in securePropertiesKeys, move them to secure props
for (String existingInsecureKey : existingInsecureKeys) {
if (securePropertiesKeys.contains(existingInsecureKey)) {
secureProperties.put(existingInsecureKey, insecureProperties.remove(existingInsecureKey));
}
}
for (Map.Entry e : properties.entrySet()) {
String key = e.getKey();
if (!securePropertiesKeys.contains(key) && !secureProperties.containsKey(key)) {
// If a key is not in securePropertiesKeys, and not in existing secure keys, add them to insecure
insecureProperties.put(key, e.getValue());
} else {
// otherwise add as secure
secureProperties.put(key, e.getValue());
}
}
ApplicationDeploymentData applicationData = params.toDataObject(appName, target, appId,
insecureProperties, secureProperties);
ApplicationDeploymentData response = method.build().jsonBody(applicationData)
.executeAndConvertToObject(ApplicationDeploymentData.class);
return new AMCDeploymentResponse(this, orgId, envId, new ApplicationDeployment(response));
} catch (JsonConvertionException e) {
throw new UnexpectedException(e);
}
}
private static RESTClientHostPathBuilder deploymentPath(RESTClientHostPathBuilder builder, String orgId, String envId) {
return builder.path("/amc/application-manager/api/v2/organizations/").path(orgId, true).path("/environments/")
.path(envId, true).path("/deployments");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy