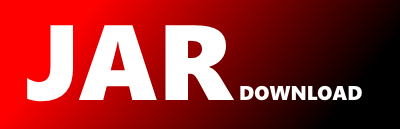
com.aeontronix.anypointsdk.amc.AMCDeploymentResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of anypoint-sdk Show documentation
Show all versions of anypoint-sdk Show documentation
Anypoint Software Development Toolkit
/*
* Copyright (c) 2023. Aeontronix Inc
*/
package com.aeontronix.anypointsdk.amc;
import com.aeontronix.anypointsdk.amc.application.DeploymentResponse;
import com.aeontronix.anypointsdk.amc.application.deployment.ApplicationDeployment;
import com.aeontronix.anypointsdk.amc.application.deployment.ApplicationDeploymentData;
import com.aeontronix.anypointsdk.amc.application.deployment.Replica;
import com.aeontronix.restclient.RESTException;
import org.slf4j.Logger;
import static org.slf4j.LoggerFactory.getLogger;
public class AMCDeploymentResponse extends DeploymentResponse {
private static final Logger logger = getLogger(AMCDeploymentResponse.class);
private AMCClient AMCClient;
private String orgId;
private String envId;
private ApplicationDeployment deployment;
public AMCDeploymentResponse(AMCClient AMCClient, String orgId, String envId, ApplicationDeployment deployment) {
this.AMCClient = AMCClient;
this.orgId = orgId;
this.envId = envId;
this.deployment = deployment;
}
public ApplicationDeployment getDeployment() {
return deployment;
}
@Override
public boolean checkDeploymentComplete() throws AMCDeploymentFailedException, RESTException {
logger.debug("Checking deployment");
ApplicationDeploymentData deploymentData = deployment.getData();
deployment = AMCClient.findDeploymentById(orgId, envId, deploymentData.getId());
String desiredVersion = deploymentData.getDesiredVersion();
logger.debug("Desired version is {}", desiredVersion);
for (Replica replica : deploymentData.getReplicas()) {
String replicaVersion = replica.getCurrentDeploymentVersion();
logger.debug("Replica version is {}", replicaVersion);
if (!replicaVersion.equals(desiredVersion)) {
logger.debug("Version is not desired one yet");
return false;
}
}
String status = deploymentData.getApplicationState().getStatus();
logger.debug("Deployment status is {}", status);
return "RUNNING".equals(status);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy