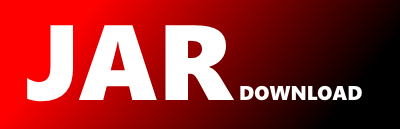
com.aeontronix.anypointsdk.amc.application.deployment.ApplicationDeploymentData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of anypoint-sdk Show documentation
Show all versions of anypoint-sdk Show documentation
Anypoint Software Development Toolkit
/*
* Copyright (c) 2023. Aeontronix Inc
*/
package com.aeontronix.anypointsdk.amc.application.deployment;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.Collections;
import java.util.List;
import java.util.Map;
public class ApplicationDeploymentData {
@JsonProperty("id")
private String id;
@JsonProperty("application")
private ApplicationState applicationState;
@JsonProperty("name")
private String name;
@JsonProperty("labels")
private List labels;
@JsonProperty("target")
private Target target;
@JsonProperty("lastSuccessfulVersion")
private String lastSuccessfulVersion;
@JsonProperty("desiredVersion")
private String desiredVersion;
@JsonProperty("creationDate")
private Long creationDate;
@JsonProperty("lastModifiedDate")
private Long lastModifiedDate;
@JsonProperty("status")
private String status;
@JsonProperty("replicas")
private List replicas;
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public ApplicationState getApplicationState(boolean create) {
if (applicationState == null && create) {
applicationState = new ApplicationState();
}
return applicationState;
}
public ApplicationState getApplicationState() {
return applicationState;
}
public void setApplicationState(ApplicationState applicationState) {
this.applicationState = applicationState;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public List getLabels() {
return labels;
}
public void setLabels(List labels) {
this.labels = labels;
}
public Long getCreationDate() {
return creationDate;
}
public void setCreationDate(Long creationDate) {
this.creationDate = creationDate;
}
public Long getLastModifiedDate() {
return lastModifiedDate;
}
public void setLastModifiedDate(Long lastModifiedDate) {
this.lastModifiedDate = lastModifiedDate;
}
public String getLastSuccessfulVersion() {
return lastSuccessfulVersion;
}
public void setLastSuccessfulVersion(String lastSuccessfulVersion) {
this.lastSuccessfulVersion = lastSuccessfulVersion;
}
public String getDesiredVersion() {
return desiredVersion;
}
public void setDesiredVersion(String desiredVersion) {
this.desiredVersion = desiredVersion;
}
public Target getTarget() {
return target;
}
public void setTarget(Target target) {
this.target = target;
}
public String getStatus() {
return status;
}
public void setStatus(String status) {
this.status = status;
}
public List getReplicas() {
return replicas;
}
public void setReplicas(List replicas) {
this.replicas = replicas;
}
@JsonIgnore
public ApplicationPropertiesService getApplicationPropertiesService() {
if (applicationState != null) {
ApplicationServices services = applicationState.getServices();
if (services != null) {
return services.getMuleAgentApplicationPropertiesService();
}
}
return null;
}
@JsonIgnore
public Map getNonSecureProperties() {
ApplicationPropertiesService propertiesService = getApplicationPropertiesService();
if (propertiesService != null && propertiesService.getProperties() != null) {
return propertiesService.getProperties();
}
return Collections.emptyMap();
}
@JsonIgnore
public Map getSecureProperties() {
ApplicationPropertiesService propertiesService = getApplicationPropertiesService();
if (propertiesService != null && propertiesService.getSecureProperties() != null) {
return propertiesService.getSecureProperties();
}
return Collections.emptyMap();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy