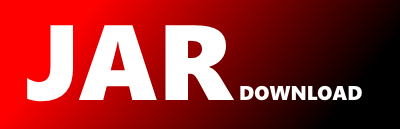
com.aeontronix.anypointsdk.amc.application.deployment.ApplicationState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of anypoint-sdk Show documentation
Show all versions of anypoint-sdk Show documentation
Anypoint Software Development Toolkit
/*
* Copyright (c) 2023. Aeontronix Inc
*/
package com.aeontronix.anypointsdk.amc.application.deployment;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.List;
public class ApplicationState {
@JsonProperty("status")
private String status;
@JsonProperty("desiredState")
private String desiredState;
@JsonProperty("ref")
private ApplicationAssetRef ref;
@JsonProperty("assets")
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy