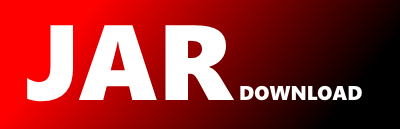
com.aeontronix.anypointsdk.cli.convert.ConvertCloudhub1To2Cmd Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of anypoint-sdk Show documentation
Show all versions of anypoint-sdk Show documentation
Anypoint Software Development Toolkit
/*
* Copyright (c) 2023. Aeontronix Inc
*/
package com.aeontronix.anypointsdk.cli.convert;
import com.aeontronix.anypointsdk.amc.application.ApplicationIdentifier;
import com.aeontronix.anypointsdk.amc.application.FileApplicationSource;
import com.aeontronix.anypointsdk.amc.application.deployment.*;
import com.aeontronix.anypointsdk.cli.AbstractCmd;
import com.aeontronix.anypointsdk.cloudhub.CHApplicationData;
import com.aeontronix.anypointsdk.cloudhub.CHApplicationPropertiesOption;
import com.aeontronix.anypointsdk.export.*;
import com.fasterxml.jackson.databind.ObjectMapper;
import picocli.CommandLine;
import java.io.File;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Map;
import java.util.concurrent.Callable;
@CommandLine.Command(name = "ch1to2")
public class ConvertCloudhub1To2Cmd extends AbstractCmd implements Callable {
@CommandLine.Parameters(defaultValue = "anypoint-export.json")
private File file;
@CommandLine.Option(names = "-o", description = "Cloudhub 2 target id", defaultValue = "cloudhub-us-east-2")
private String targetId;
@SuppressWarnings("unchecked")
@Override
public Integer call() throws Exception {
AnypointExportFile exportFile = new ObjectMapper().readValue(file, AnypointExportFile.class);
for (OrgExport org : exportFile.getOrgs()) {
for (EnvExport env : org.getEnvs()) {
ArrayList amcApps = env.getAmcApps();
ArrayList appList = env.getCloudhubApps();
while (!appList.isEmpty()) {
CloudhubAppExport app = appList.remove(0);
CHApplicationData ch = app.getData();
appList.remove(app);
ApplicationDeploymentData data = new ApplicationDeploymentData();
data.setName(ch.getDomain());
Target target = new Target();
target.setTargetId(targetId);
data.setTarget(target);
ApplicationState appState = new ApplicationState();
appState.setDesiredState("STARTED");
data.setApplicationState(appState);
Map propertiesOptions = ch.getPropertiesOptions();
ApplicationPropertiesService muleAgentApplicationPropertiesService = new ApplicationPropertiesService();
ApplicationServices services = new ApplicationServices();
services.setMuleAgentApplicationPropertiesService(muleAgentApplicationPropertiesService);
appState.setServices(services);
Map secureProperties = new HashMap<>();
muleAgentApplicationPropertiesService.setSecureProperties(secureProperties);
Map nonSecureProperties = new HashMap<>();
muleAgentApplicationPropertiesService.setProperties(nonSecureProperties);
for (Map.Entry p : ch.getProperties().entrySet()) {
String key = p.getKey();
CHApplicationPropertiesOption secProps = propertiesOptions.get(key);
muleAgentApplicationPropertiesService.setProperties(nonSecureProperties);
if (secProps != null && secProps.isSecure()) {
secureProperties.put(key, p.getValue());
} else {
nonSecureProperties.put(key, p.getValue());
}
}
DeploymentSettings deploymentSettings = new DeploymentSettings();
deploymentSettings.setClustered(true);
Inbound inbound = new Inbound();
inbound.setPublicUrl("FIXME");
inbound.setLastMileSecurity(false);
inbound.setForwardSslSession(false);
deploymentSettings.setRuntimeVersion(ch.getMuleVersion().getVersion());
deploymentSettings.setUpdateStrategy("rolling");
deploymentSettings.setHttp(new ApplicationHttp(inbound));
target.setDeploymentSettings(deploymentSettings);
target.setReplicas(ch.getWorkers().getAmount());
appState.setvCores("0.1");
AMCAppExport amcApp = new AMCAppExport(data);
amcApp.setFile(app.getFileName());
File appFile = new File(app.getFileName());
if (appFile.exists()) {
ApplicationIdentifier appId = new FileApplicationSource(appFile).getApplicationIdentifier();
appState.setRef(new ApplicationAssetRef(appId.getGroupId(), appId.getArtifactId(), appId.getVersion()));
}
amcApp.setFile(app.getFileName());
amcApps.add(amcApp);
}
}
}
exportFile.write(file);
return 0;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy