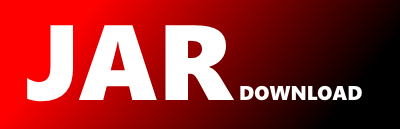
com.aeontronix.anypointsdk.cloudhub.CloudhubClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of anypoint-sdk Show documentation
Show all versions of anypoint-sdk Show documentation
Anypoint Software Development Toolkit
package com.aeontronix.anypointsdk.cloudhub;
import com.aeontronix.anypointsdk.AnypointClient;
import com.aeontronix.anypointsdk.AnypointException;
import com.aeontronix.commons.exception.UnexpectedException;
import com.aeontronix.commons.io.IOUtils;
import com.aeontronix.restclient.RESTClientHost;
import com.aeontronix.restclient.RESTException;
import com.aeontronix.restclient.RESTResponse;
import org.slf4j.Logger;
import java.io.IOException;
import java.io.OutputStream;
import java.util.List;
import java.util.Optional;
import java.util.stream.Collectors;
import static org.slf4j.LoggerFactory.getLogger;
public class CloudhubClient {
private static final Logger logger = getLogger(CloudhubClient.class);
public static final String PATH_LIST_CH_APPS = "cloudhub/api/v2/applications";
private final RESTClientHost restClient;
private AnypointClient anypointClient;
public CloudhubClient(AnypointClient anypointClient) {
this.anypointClient = anypointClient;
restClient = anypointClient.getAnypointRestClient();
}
public List listCHApplications(String envId) throws AnypointException {
return listCHApplications(envId, false, null, null);
}
public List listCHApplications(String envId, boolean retrieveStatistics, Long statsPeriod, Long statsIntervalCount) throws AnypointException {
try {
return restClient.get().path(PATH_LIST_CH_APPS)
.queryParam("period", statsPeriod)
.queryParam("intervalCount", statsIntervalCount)
.queryParam("retrieveStatistics", retrieveStatistics)
.build().header(AnypointClient.HEADER_ENV, envId)
.executeAndConvertToList(CHApplicationData.class).stream().map(CHApplication::new).collect(Collectors.toList());
} catch (RESTException e) {
throw new AnypointException(e);
}
}
public Optional describeApplication(String orgId, String envId, String domain) throws RESTException {
try {
return Optional.of(restClient.get().path(PATH_LIST_CH_APPS).path(domain, true)
.build()
.header(AnypointClient.HEADER_ORG, orgId)
.header(AnypointClient.HEADER_ENV, envId)
.executeAndConvertToObject(CHApplication.class));
} catch (RESTException e) {
if (e.getStatusCode() == 404) {
return Optional.empty();
} else {
throw e;
}
}
}
public void downloadApplicationFile(String orgId, String envId, String appDomain, String filename, OutputStream os) throws RESTException {
try (RESTResponse results = restClient.get()
.path("cloudhub/api/organizations/").path(orgId, true)
.path("/environments/").path(envId, true)
.path("/applications/").path(appDomain, true)
.path("/download").path(filename, true)
.build().execute()) {
IOUtils.copy(results.getContentStream(), os);
} catch (IOException e) {
throw new RESTException(e);
}
}
public List findCHMuleVersions() throws RESTException {
return restClient.get("/cloudhub/api/mule-versions").executeAndConvertToList(CHMuleVersion.class, "/data");
}
public CHMuleVersion findDefaultCHMuleVersion() throws RESTException {
for (CHMuleVersion version : findCHMuleVersions()) {
if (version.isRecommended()) {
return version;
}
}
throw new UnexpectedException("No default mule version found");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy