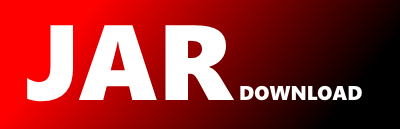
com.aeontronix.anypointsdk.exchange.CreateExchangeAssetRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of anypoint-sdk Show documentation
Show all versions of anypoint-sdk Show documentation
Anypoint Software Development Toolkit
/*
* Copyright (c) 2023. Aeontronix Inc
*/
package com.aeontronix.anypointsdk.exchange;
import com.aeontronix.anypointsdk.AnypointClient;
import com.aeontronix.commons.file.TempFile;
import com.aeontronix.restclient.RESTClientRequestBuilder;
import com.aeontronix.restclient.RESTException;
import com.aeontronix.restclient.RESTResponse;
import org.apache.http.Header;
import org.apache.http.HttpEntity;
import org.apache.http.entity.mime.MultipartEntityBuilder;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.*;
import java.util.stream.Collectors;
public class CreateExchangeAssetRequest {
private boolean syncPublish = true;
private AnypointClient anypointClient;
private String orgId;
private String groupId;
private String assetId;
private String version;
private String name;
private String description;
private String type;
private List dependencies;
private List keywords;
private List tags;
private Map> fields;
private Map> categories;
private String contactName;
private String contactEmail;
private Map properties;
private final List files = new ArrayList<>();
private Status status = Status.PUBLISHED;
CreateExchangeAssetRequest(AnypointClient anypointClient) {
this.anypointClient = anypointClient;
}
CreateExchangeAssetRequest(AnypointClient anypointClient, String orgId, String assetId, String version, String name) {
this.anypointClient = anypointClient;
this.orgId = orgId;
this.groupId = orgId;
this.assetId = assetId;
this.version = version;
this.orgId = orgId;
this.name = name;
}
public RESTResponse execute() throws IOException, RESTException {
MultipartEntityBuilder builder = MultipartEntityBuilder.create();
addPart(builder, "name", name);
addPart(builder, "description", description);
addPart(builder, "type", type);
addPart(builder, "dependencies", dependencies);
addPart(builder, "keywords", keywords);
addPart(builder, "tags", tags);
addPart(builder, "fields", fields);
addPart(builder, "categories", categories);
addPart(builder, "contactName", contactName);
addPart(builder, "contactEmail", contactEmail);
addPart(builder, "status", status.name().toLowerCase());
if (properties != null && !properties.isEmpty()) {
for (Map.Entry e : properties.entrySet()) {
addPart(builder, "properties." + e.getKey(), e.getValue());
}
}
for (FileFilePart file : files) {
builder = file.addPart(builder);
}
try (TempFile tmp = new TempFile("exchast")) {
String contentTypeHeaderKey;
String contentTypeHeaderValue;
String contentEncodingHeaderKey;
String contentEncodingHeaderValue;
try (FileOutputStream fos = new FileOutputStream(tmp)) {
HttpEntity entity = builder.build();
Header contentTypeHeader = entity.getContentType();
contentTypeHeaderKey = contentTypeHeader.getName();
contentTypeHeaderValue = contentTypeHeader.getValue();
Header contentEncoding = entity.getContentEncoding();
contentEncodingHeaderKey = contentEncoding != null ? contentEncoding.getName() : null;
contentEncodingHeaderValue = contentEncoding != null ? contentEncoding.getValue() : null;
entity.writeTo(fos);
}
RESTClientRequestBuilder requestBuilder = anypointClient.getAnypointRestClient().post().
path("/exchange/api/v2/organizations")
.path(orgId, true)
.path("assets")
.path(groupId != null ? groupId : orgId, true)
.path(assetId, true)
.path(version, true).build()
.entity(new FileInputStream(tmp))
.header(contentTypeHeaderKey, contentTypeHeaderValue)
.header("x-sync-publication", Boolean.toString(syncPublish));
if (contentTypeHeaderKey != null && contentEncodingHeaderValue != null) {
requestBuilder = requestBuilder.header(contentEncodingHeaderKey, contentEncodingHeaderValue);
}
try {
return requestBuilder.execute();
} catch (RESTException e) {
String message = e.getMessage();
if (e.getStatusCode() == 400 && message != null && message.contains("trying to set type: app. Expected type is: rest-api")) {
message = "Unable to upload application with artifact id " + assetId + " because there is " +
"an API specification with the same id. When deploying to RTF or CH2 your application's artifactId (in the pom.xml file) " +
" must have a different id from your REST API Specification exchange id";
throw new RESTException(message, 400, message);
} else {
throw e;
}
}
}
}
public CreateExchangeAssetRequest syncPublish(boolean syncPublish) {
this.syncPublish = syncPublish;
return this;
}
public CreateExchangeAssetRequest groupId(String groupId) {
this.groupId = groupId;
return this;
}
public CreateExchangeAssetRequest assetId(String assetId) {
this.assetId = assetId;
return this;
}
public CreateExchangeAssetRequest version(String version) {
this.version = version;
return this;
}
public CreateExchangeAssetRequest orgId(String orgId) {
this.orgId = orgId;
return this;
}
public CreateExchangeAssetRequest name(String name) {
this.name = name;
return this;
}
public CreateExchangeAssetRequest description(String description) {
this.description = description;
return this;
}
public CreateExchangeAssetRequest type(String type) {
this.type = type;
return this;
}
public CreateExchangeAssetRequest dependencies(List dependencies) {
this.dependencies = dependencies;
return this;
}
public CreateExchangeAssetRequest keywords(List keywords) {
this.keywords = keywords;
return this;
}
public CreateExchangeAssetRequest tags(List tags) {
this.tags = tags;
return this;
}
public CreateExchangeAssetRequest fields(Map> fields) {
this.fields = fields;
return this;
}
public CreateExchangeAssetRequest categories(Map> categories) {
this.categories = categories;
return this;
}
public CreateExchangeAssetRequest contactName(String contactName) {
this.contactName = contactName;
return this;
}
public CreateExchangeAssetRequest contactEmail(String contactEmail) {
this.contactEmail = contactEmail;
return this;
}
public CreateExchangeAssetRequest properties(Map properties) {
this.properties = properties;
return this;
}
public CreateExchangeAssetRequest file(String packaging, String classifier, String contentType, String filename, File file) {
this.files.add(new FileFilePart(packaging, classifier, contentType, filename, file));
return this;
}
public CreateExchangeAssetRequest files(List files) {
this.files.clear();
this.files.addAll(files);
return this;
}
private static void addPart(MultipartEntityBuilder builder, String prefix, Map> values) {
if (values != null && !values.isEmpty()) {
for (Map.Entry> entry : values.entrySet()) {
addPart(builder, prefix + "." + entry.getKey(), entry.getValue());
}
}
}
private static void addPart(MultipartEntityBuilder builder, String key, Collection values) {
if (values != null && !values.isEmpty()) {
addPart(builder, key, values.stream().collect(Collectors.joining(",")));
}
}
private static void addPart(MultipartEntityBuilder builder, String key, String value) {
if (value != null) {
builder.addTextBody(key, value);
}
}
public CreateExchangeAssetRequest status(Status status) {
this.status = status;
return this;
}
public enum Status {
DEVELOPMENT, PUBLISHED, DEPRECATED
}
public static abstract class FilePart {
private String packaging;
private String classifier;
private String contentType;
private String filename;
FilePart(String packaging, String classifier, String contentType, String filename) {
this.packaging = packaging;
this.classifier = classifier;
this.contentType = contentType;
this.filename = filename;
}
public String getFilename() {
return filename;
}
public void setFilename(String filename) {
this.filename = filename;
}
public String getPackaging() {
return packaging;
}
public void setPackaging(String packaging) {
this.packaging = packaging;
}
public String getClassifier() {
return classifier;
}
public void setClassifier(String classifier) {
this.classifier = classifier;
}
public String getContentType() {
return contentType;
}
public void setContentType(String contentType) {
this.contentType = contentType;
}
public abstract MultipartEntityBuilder addPart(MultipartEntityBuilder builder);
}
public static class FileFilePart extends FilePart {
private File file;
public FileFilePart(String packaging, String classifier, String contentType, String filename, File file) {
super(packaging, classifier, contentType, filename != null ? filename : file.getPath());
this.file = file;
}
@Override
public MultipartEntityBuilder addPart(MultipartEntityBuilder builder) {
String classifier = getClassifier();
String packaging = getPackaging();
if (classifier == null) {
if (file.getName().endsWith("-mule-application.jar")) {
classifier = "mule-application";
} else {
throw new IllegalArgumentException("Classifier not specified and couldn't be identified");
}
}
if (packaging == null) {
throw new IllegalArgumentException("Packaging not specified and couldn't be identified");
}
return builder.addBinaryBody("files." + classifier + "." + packaging, file);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy