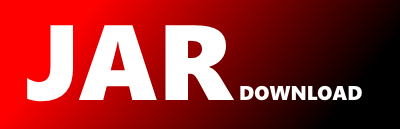
com.aeontronix.anypointsdk.exchange.ExchangeClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of anypoint-sdk Show documentation
Show all versions of anypoint-sdk Show documentation
Anypoint Software Development Toolkit
package com.aeontronix.anypointsdk.exchange;
import com.aeontronix.anypointsdk.AnypointClient;
import com.aeontronix.anypointsdk.apimanager.APIContractData;
import com.aeontronix.commons.exception.UnexpectedException;
import com.aeontronix.restclient.PaginatedResponse;
import com.aeontronix.restclient.RESTClientHostPathBuilder;
import com.aeontronix.restclient.RESTException;
import com.aeontronix.restclient.json.JsonConvertionException;
import org.jetbrains.annotations.NotNull;
import java.io.IOException;
import java.util.HashMap;
import java.util.List;
import java.util.stream.Collectors;
public class ExchangeClient {
private AnypointClient anypointClient;
public ExchangeClient(AnypointClient anypointClient) {
this.anypointClient = anypointClient;
}
public CreateExchangeAssetRequest createAsset() {
return new CreateExchangeAssetRequest(anypointClient);
}
public CreateExchangeAssetRequest createAsset(String orgId, String assetId, String version, String name) {
return new CreateExchangeAssetRequest(anypointClient, orgId, assetId, version, name);
}
public List listClientApplications(String orgId) throws RESTException {
try {
try (PaginatedResponse response = anypointClient.getAnypointRestClient().get().path("/exchange/api/v1/organizations").path(orgId)
.path("/applications").build().paginate().pageValuesPath("").executePaginated(ExchangeClientApplicationData.class)) {
return response.toList().stream().map(ExchangeClientApplication::new).collect(Collectors.toList());
}
} catch (IOException e) {
throw new RESTException(e);
}
}
public ExchangeClientApplication findClientApplicationById(String orgId, String id) throws RESTException {
try {
return new ExchangeClientApplication(anypointClient.getAnypointRestClient().get().path("/exchange/api/v1/organizations").path(orgId)
.path("/applications").path(id).build().executeAndConvertToObject(ExchangeClientApplicationData.class));
} catch (RESTException e) {
if (e.getStatusCode() == 403) {
throw new RESTException("Client application either doesn't exist or not authorized to access", e.getStatusCode(), e.getReason());
} else {
throw e;
}
}
}
public ExchangeClientApplication createClientApplication(@NotNull String orgId, @NotNull String name, String url,
String description, List redirectUri,
List grantTypes,
String apiEndpoints,
String accessedAPIInstanceId) throws JsonConvertionException, RESTException {
HashMap req = new HashMap<>();
req.put("name", name);
req.put("description", description != null ? description : "");
req.put("redirectUri", redirectUri);
req.put("apiEndpoints", false);
req.put("grantTypes", grantTypes);
req.put("url", url);
RESTClientHostPathBuilder builder = anypointClient.getAnypointRestClient().post()
.path("/exchange/api/v1/organizations/").path(orgId).path("/applications");
if (accessedAPIInstanceId != null) {
builder.queryParam("apiInstanceId", accessedAPIInstanceId);
}
return new ExchangeClientApplication(builder.build().jsonBody(req).executeAndConvertToObject(ExchangeClientApplicationData.class));
}
public APIContractData createAPIContract(String applicationId, String apiId, String organizationId, String environmentId, boolean acceptedTerms,
String groupId, String assetId, String version, String productAPIVersion, Long tierId) throws RESTException {
HashMap req = new HashMap<>();
req.put("apiId", apiId);
req.put("environmentId", environmentId);
req.put("acceptedTerms", acceptedTerms);
req.put("organizationId", organizationId);
req.put("groupId", groupId);
req.put("assetId", assetId);
req.put("version", version);
req.put("productAPIVersion", productAPIVersion);
if (tierId != null) {
req.put("requestedTierId", tierId);
}
try {
return anypointClient.getAnypointRestClient().post().path("/exchange/api/v1/organizations/").path(organizationId)
.path("/applications/").path(applicationId).path("/contracts").build().jsonBody(req)
.executeAndConvertToObject(APIContractData.class);
} catch (JsonConvertionException e) {
throw new UnexpectedException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy