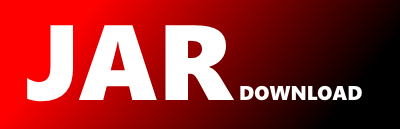
com.aerospike.documentapi.JsonConverters Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aerospike-document-api Show documentation
Show all versions of aerospike-document-api Show documentation
This project provides an API which allows Aerospike CDT (Collection Data Type) objects to be accessed and mutated using JSON like syntax.
Effectively this provides what can be termed a document API as CDT objects can be used to represent JSON in the Aerospike database.
package com.aerospike.documentapi;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import java.io.IOException;
import java.util.List;
import java.util.Map;
public class JsonConverters {
private static final ObjectMapper mapper = new ObjectMapper();
/**
* Given a serialized json object, return it's equivalent representation as a JsonNode.
*
* @param jsonString A given JSON as a String.
* @return The given JSON as a JsonNode.
* @throws IOException an IOException will be thrown in case of an error.
*/
public static JsonNode convertStringToJsonNode(String jsonString) throws IOException {
return mapper.readValue(jsonString, JsonNode.class);
}
/**
* Given a serialized JsonNode, return it's equivalent representation as a Java map.
*
* @param jsonNode A given JSON as a JsonNode.
* @return The given JSON as a Java Map.
*/
public static Map convertJsonNodeToMap(JsonNode jsonNode) {
return mapper.convertValue(jsonNode, new TypeReference
© 2015 - 2024 Weber Informatics LLC | Privacy Policy